diff --git a/README.md b/README.md index a65213ab..e84c8392 100644 --- a/README.md +++ b/README.md @@ -134,7 +134,7 @@ If you think this project is helpful to you, you can help the author buy a cup o 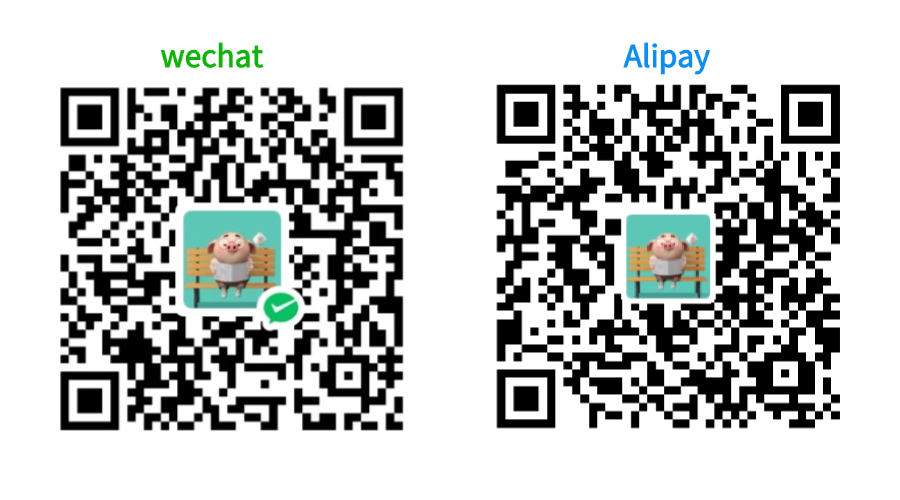 -Paypal Me +Paypal Me ## Contributor diff --git a/apps/web-antd/src/adapter/component/index.ts b/apps/web-antd/src/adapter/component/index.ts new file mode 100644 index 00000000..12849dc3 --- /dev/null +++ b/apps/web-antd/src/adapter/component/index.ts @@ -0,0 +1,127 @@ +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + AutoComplete, + Button, + Checkbox, + CheckboxGroup, + DatePicker, + Divider, + Input, + InputNumber, + InputPassword, + Mentions, + notification, + Radio, + RadioGroup, + RangePicker, + Rate, + Select, + Space, + Switch, + Textarea, + TimePicker, + TreeSelect, + Upload, +} from 'ant-design-vue'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'AutoComplete' + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'DefaultButton' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'InputPassword' + | 'Mentions' + | 'PrimaryButton' + | 'Radio' + | 'RadioGroup' + | 'RangePicker' + | 'Rate' + | 'Select' + | 'Space' + | 'Switch' + | 'Textarea' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + AutoComplete, + Checkbox, + CheckboxGroup, + DatePicker, + // 自定义默认按钮 + DefaultButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'default' }, slots); + }, + Divider, + Input: withDefaultPlaceholder(Input, 'input'), + InputNumber: withDefaultPlaceholder(InputNumber, 'input'), + InputPassword: withDefaultPlaceholder(InputPassword, 'input'), + Mentions: withDefaultPlaceholder(Mentions, 'input'), + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'primary' }, slots); + }, + Radio, + RadioGroup, + RangePicker, + Rate, + Select: withDefaultPlaceholder(Select, 'select'), + Space, + Switch, + Textarea: withDefaultPlaceholder(Textarea, 'input'), + TimePicker, + TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), + Upload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + notification.success({ + description: content, + message: title, + placement: 'bottomRight', + }); + }, + }); +} + +export { initComponentAdapter }; diff --git a/apps/web-antd/src/adapter/form.ts b/apps/web-antd/src/adapter/form.ts index 48c981f7..9b95ac75 100644 --- a/apps/web-antd/src/adapter/form.ts +++ b/apps/web-antd/src/adapter/form.ts @@ -1,105 +1,14 @@ import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import type { Component, SetupContext } from 'vue'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - AutoComplete, - Button, - Checkbox, - CheckboxGroup, - DatePicker, - Divider, - Input, - InputNumber, - InputPassword, - Mentions, - Radio, - RadioGroup, - RangePicker, - Rate, - Select, - Space, - Switch, - Textarea, - TimePicker, - TreeSelect, - Upload, -} from 'ant-design-vue'; - -// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 -export type FormComponentType = - | 'AutoComplete' - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'InputPassword' - | 'Mentions' - | 'Radio' - | 'RadioGroup' - | 'RangePicker' - | 'Rate' - | 'Select' - | 'Space' - | 'Switch' - | 'Textarea' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -const withDefaultPlaceholder = ( - component: T, - type: 'input' | 'select', -) => { - return (props: any, { attrs, slots }: Omit) => { - const placeholder = props?.placeholder || $t(`placeholder.${type}`); - return h(component, { ...props, ...attrs, placeholder }, slots); - }; -}; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - AutoComplete, - Checkbox, - CheckboxGroup, - DatePicker, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'default' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'primary' }, slots); - }, - Divider, - Input: withDefaultPlaceholder(Input, 'input'), - InputNumber: withDefaultPlaceholder(InputNumber, 'input'), - InputPassword: withDefaultPlaceholder(InputPassword, 'input'), - Mentions: withDefaultPlaceholder(Mentions, 'input'), - Radio, - RadioGroup, - RangePicker, - Rate, - Select: withDefaultPlaceholder(Select, 'select'), - Space, - Switch, - Textarea: withDefaultPlaceholder(Textarea, 'input'), - TimePicker, - TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), - Upload, - }, +setupVbenForm({ config: { // ant design vue组件库默认都是 v-model:value baseModelPropName: 'value', @@ -130,9 +39,9 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; diff --git a/apps/web-antd/src/bootstrap.ts b/apps/web-antd/src/bootstrap.ts index 1be9bbe0..f88b41b1 100644 --- a/apps/web-antd/src/bootstrap.ts +++ b/apps/web-antd/src/bootstrap.ts @@ -7,10 +7,14 @@ import '@vben/styles/antd'; import { setupI18n } from '#/locales'; +import { initComponentAdapter } from './adapter/component'; import App from './app.vue'; import { router } from './router'; async function bootstrap(namespace: string) { + // 初始化组件适配器 + await initComponentAdapter(); + const app = createApp(App); // 国际化 i18n 配置 diff --git a/apps/web-antd/src/router/routes/core.ts b/apps/web-antd/src/router/routes/core.ts index f793a57e..d85de5a4 100644 --- a/apps/web-antd/src/router/routes/core.ts +++ b/apps/web-antd/src/router/routes/core.ts @@ -32,6 +32,7 @@ const coreRoutes: RouteRecordRaw[] = [ { component: AuthPageLayout, meta: { + hideInTab: true, title: 'Authentication', }, name: 'Authentication', diff --git a/apps/web-ele/src/adapter/component/index.ts b/apps/web-ele/src/adapter/component/index.ts new file mode 100644 index 00000000..1d415bc8 --- /dev/null +++ b/apps/web-ele/src/adapter/component/index.ts @@ -0,0 +1,104 @@ +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + ElButton, + ElCheckbox, + ElCheckboxGroup, + ElDivider, + ElInput, + ElInputNumber, + ElNotification, + ElRadioGroup, + ElSelect, + ElSpace, + ElSwitch, + ElTimePicker, + ElTreeSelect, + ElUpload, +} from 'element-plus'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'RadioGroup' + | 'Select' + | 'Space' + | 'Switch' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + Checkbox: ElCheckbox, + CheckboxGroup: ElCheckboxGroup, + // 自定义默认按钮 + DefaulButton: (props, { attrs, slots }) => { + return h(ElButton, { ...props, attrs, type: 'info' }, slots); + }, + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(ElButton, { ...props, attrs, type: 'primary' }, slots); + }, + Divider: ElDivider, + Input: withDefaultPlaceholder(ElInput, 'input'), + InputNumber: withDefaultPlaceholder(ElInputNumber, 'input'), + RadioGroup: ElRadioGroup, + Select: withDefaultPlaceholder(ElSelect, 'select'), + Space: ElSpace, + Switch: ElSwitch, + TimePicker: ElTimePicker, + TreeSelect: withDefaultPlaceholder(ElTreeSelect, 'select'), + Upload: ElUpload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + ElNotification({ + title, + message: content, + position: 'bottom-right', + duration: 0, + type: 'success', + }); + }, + }); +} + +export { initComponentAdapter }; diff --git a/apps/web-ele/src/adapter/form.ts b/apps/web-ele/src/adapter/form.ts index 36e20033..832a6342 100644 --- a/apps/web-ele/src/adapter/form.ts +++ b/apps/web-ele/src/adapter/form.ts @@ -1,82 +1,14 @@ import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import type { Component, SetupContext } from 'vue'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - ElButton, - ElCheckbox, - ElCheckboxGroup, - ElDivider, - ElInput, - ElInputNumber, - ElRadioGroup, - ElSelect, - ElSpace, - ElSwitch, - ElTimePicker, - ElTreeSelect, - ElUpload, -} from 'element-plus'; -// 业务表单组件适配 - -export type FormComponentType = - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'RadioGroup' - | 'Select' - | 'Space' - | 'Switch' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -const withDefaultPlaceholder = ( - component: T, - type: 'input' | 'select', -) => { - return (props: any, { attrs, slots }: Omit) => { - const placeholder = props?.placeholder || $t(`placeholder.${type}`); - return h(component, { ...props, ...attrs, placeholder }, slots); - }; -}; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - Checkbox: ElCheckbox, - CheckboxGroup: ElCheckboxGroup, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(ElButton, { ...props, attrs, type: 'info' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(ElButton, { ...props, attrs, type: 'primary' }, slots); - }, - Divider: ElDivider, - Input: withDefaultPlaceholder(ElInput, 'input'), - InputNumber: withDefaultPlaceholder(ElInputNumber, 'input'), - RadioGroup: ElRadioGroup, - Select: withDefaultPlaceholder(ElSelect, 'select'), - Space: ElSpace, - Switch: ElSwitch, - TimePicker: ElTimePicker, - TreeSelect: withDefaultPlaceholder(ElTreeSelect, 'select'), - Upload: ElUpload, - }, +setupVbenForm({ config: { modelPropNameMap: { Upload: 'fileList', @@ -98,9 +30,9 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; diff --git a/apps/web-ele/src/bootstrap.ts b/apps/web-ele/src/bootstrap.ts index 5494cfba..7311d665 100644 --- a/apps/web-ele/src/bootstrap.ts +++ b/apps/web-ele/src/bootstrap.ts @@ -7,10 +7,13 @@ import '@vben/styles/ele'; import { setupI18n } from '#/locales'; +import { initComponentAdapter } from './adapter/component'; import App from './app.vue'; import { router } from './router'; async function bootstrap(namespace: string) { + // 初始化组件适配器 + await initComponentAdapter(); const app = createApp(App); // 国际化 i18n 配置 diff --git a/apps/web-ele/src/router/routes/core.ts b/apps/web-ele/src/router/routes/core.ts index f793a57e..d85de5a4 100644 --- a/apps/web-ele/src/router/routes/core.ts +++ b/apps/web-ele/src/router/routes/core.ts @@ -32,6 +32,7 @@ const coreRoutes: RouteRecordRaw[] = [ { component: AuthPageLayout, meta: { + hideInTab: true, title: 'Authentication', }, name: 'Authentication', diff --git a/apps/web-naive/src/adapter/component/index.ts b/apps/web-naive/src/adapter/component/index.ts new file mode 100644 index 00000000..d2b5f192 --- /dev/null +++ b/apps/web-naive/src/adapter/component/index.ts @@ -0,0 +1,103 @@ +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + NButton, + NCheckbox, + NCheckboxGroup, + NDatePicker, + NDivider, + NInput, + NInputNumber, + NRadioGroup, + NSelect, + NSpace, + NSwitch, + NTimePicker, + NTreeSelect, + NUpload, +} from 'naive-ui'; + +import { message } from '#/adapter/naive'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'RadioGroup' + | 'Select' + | 'Space' + | 'Switch' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + Checkbox: NCheckbox, + CheckboxGroup: NCheckboxGroup, + DatePicker: NDatePicker, + // 自定义默认按钮 + DefaultButton: (props, { attrs, slots }) => { + return h(NButton, { ...props, attrs, type: 'info' }, slots); + }, + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(NButton, { ...props, attrs, type: 'primary' }, slots); + }, + Divider: NDivider, + Input: withDefaultPlaceholder(NInput, 'input'), + InputNumber: withDefaultPlaceholder(NInputNumber, 'input'), + RadioGroup: NRadioGroup, + Select: withDefaultPlaceholder(NSelect, 'select'), + Space: NSpace, + Switch: NSwitch, + TimePicker: NTimePicker, + TreeSelect: withDefaultPlaceholder(NTreeSelect, 'select'), + Upload: NUpload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + message.success(content || title, { + duration: 0, + }); + }, + }); +} + +export { initComponentAdapter }; diff --git a/apps/web-naive/src/adapter/form.ts b/apps/web-naive/src/adapter/form.ts index f7142e78..511518cc 100644 --- a/apps/web-naive/src/adapter/form.ts +++ b/apps/web-naive/src/adapter/form.ts @@ -1,84 +1,14 @@ import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import type { Component, SetupContext } from 'vue'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - NButton, - NCheckbox, - NCheckboxGroup, - NDatePicker, - NDivider, - NInput, - NInputNumber, - NRadioGroup, - NSelect, - NSpace, - NSwitch, - NTimePicker, - NTreeSelect, - NUpload, -} from 'naive-ui'; -// 业务表单组件适配 - -export type FormComponentType = - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'RadioGroup' - | 'Select' - | 'Space' - | 'Switch' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -const withDefaultPlaceholder = ( - component: T, - type: 'input' | 'select', -) => { - return (props: any, { attrs, slots }: Omit) => { - const placeholder = props?.placeholder || $t(`placeholder.${type}`); - return h(component, { ...props, ...attrs, placeholder }, slots); - }; -}; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - Checkbox: NCheckbox, - CheckboxGroup: NCheckboxGroup, - DatePicker: NDatePicker, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(NButton, { ...props, attrs, type: 'info' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(NButton, { ...props, attrs, type: 'primary' }, slots); - }, - Divider: NDivider, - Input: withDefaultPlaceholder(NInput, 'input'), - InputNumber: withDefaultPlaceholder(NInputNumber, 'input'), - RadioGroup: NRadioGroup, - Select: withDefaultPlaceholder(NSelect, 'select'), - Space: NSpace, - Switch: NSwitch, - TimePicker: NTimePicker, - TreeSelect: withDefaultPlaceholder(NTreeSelect, 'select'), - Upload: NUpload, - }, +setupVbenForm({ config: { // naive-ui组件不接受onChang事件,所以需要禁用 disabledOnChangeListener: true, @@ -107,9 +37,9 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; diff --git a/apps/web-naive/src/bootstrap.ts b/apps/web-naive/src/bootstrap.ts index 6604dec2..13ab95cb 100644 --- a/apps/web-naive/src/bootstrap.ts +++ b/apps/web-naive/src/bootstrap.ts @@ -6,10 +6,13 @@ import '@vben/styles'; import { setupI18n } from '#/locales'; +import { initComponentAdapter } from './adapter/component'; import App from './app.vue'; import { router } from './router'; async function bootstrap(namespace: string) { + // 初始化组件适配器 + initComponentAdapter(); const app = createApp(App); // 国际化 i18n 配置 diff --git a/apps/web-naive/src/router/routes/core.ts b/apps/web-naive/src/router/routes/core.ts index f793a57e..d85de5a4 100644 --- a/apps/web-naive/src/router/routes/core.ts +++ b/apps/web-naive/src/router/routes/core.ts @@ -32,6 +32,7 @@ const coreRoutes: RouteRecordRaw[] = [ { component: AuthPageLayout, meta: { + hideInTab: true, title: 'Authentication', }, name: 'Authentication', diff --git a/docs/.vitepress/config/zh.mts b/docs/.vitepress/config/zh.mts index ff0f2256..5c8505ab 100644 --- a/docs/.vitepress/config/zh.mts +++ b/docs/.vitepress/config/zh.mts @@ -164,6 +164,10 @@ function sidebarComponents(): DefaultTheme.SidebarItem[] { link: 'common-ui/vben-form', text: 'Form 表单', }, + { + link: 'common-ui/vben-vxe-table', + text: 'Vxe Table 表格', + }, { link: 'common-ui/vben-count-to-animator', text: 'CountToAnimator 数字动画', diff --git a/docs/src/_env/adapter/component.ts b/docs/src/_env/adapter/component.ts new file mode 100644 index 00000000..12849dc3 --- /dev/null +++ b/docs/src/_env/adapter/component.ts @@ -0,0 +1,127 @@ +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + AutoComplete, + Button, + Checkbox, + CheckboxGroup, + DatePicker, + Divider, + Input, + InputNumber, + InputPassword, + Mentions, + notification, + Radio, + RadioGroup, + RangePicker, + Rate, + Select, + Space, + Switch, + Textarea, + TimePicker, + TreeSelect, + Upload, +} from 'ant-design-vue'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'AutoComplete' + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'DefaultButton' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'InputPassword' + | 'Mentions' + | 'PrimaryButton' + | 'Radio' + | 'RadioGroup' + | 'RangePicker' + | 'Rate' + | 'Select' + | 'Space' + | 'Switch' + | 'Textarea' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + AutoComplete, + Checkbox, + CheckboxGroup, + DatePicker, + // 自定义默认按钮 + DefaultButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'default' }, slots); + }, + Divider, + Input: withDefaultPlaceholder(Input, 'input'), + InputNumber: withDefaultPlaceholder(InputNumber, 'input'), + InputPassword: withDefaultPlaceholder(InputPassword, 'input'), + Mentions: withDefaultPlaceholder(Mentions, 'input'), + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'primary' }, slots); + }, + Radio, + RadioGroup, + RangePicker, + Rate, + Select: withDefaultPlaceholder(Select, 'select'), + Space, + Switch, + Textarea: withDefaultPlaceholder(Textarea, 'input'), + TimePicker, + TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), + Upload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + notification.success({ + description: content, + message: title, + placement: 'bottomRight', + }); + }, + }); +} + +export { initComponentAdapter }; diff --git a/docs/src/_env/adapter/form.ts b/docs/src/_env/adapter/form.ts index b55b9b0a..760cf7ce 100644 --- a/docs/src/_env/adapter/form.ts +++ b/docs/src/_env/adapter/form.ts @@ -1,99 +1,23 @@ import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - AutoComplete, - Button, - Checkbox, - CheckboxGroup, - DatePicker, - Divider, - Input, - InputNumber, - InputPassword, - Mentions, - Radio, - RadioGroup, - RangePicker, - Rate, - Select, - Space, - Switch, - Textarea, - TimePicker, - TreeSelect, - Upload, -} from 'ant-design-vue'; +import { initComponentAdapter } from './component'; -// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 -export type FormComponentType = - | 'AutoComplete' - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'InputPassword' - | 'Mentions' - | 'Radio' - | 'RadioGroup' - | 'RangePicker' - | 'Rate' - | 'Select' - | 'Space' - | 'Switch' - | 'Textarea' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - AutoComplete, - Checkbox, - CheckboxGroup, - DatePicker, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'default' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'primary' }, slots); - }, - Divider, - Input, - InputNumber, - InputPassword, - Mentions, - Radio, - RadioGroup, - RangePicker, - Rate, - Select, - Space, - Switch, - Textarea, - TimePicker, - TreeSelect, - Upload, - }, +initComponentAdapter(); +setupVbenForm({ config: { - // ant design vue组件库默认都是 v-model:value baseModelPropName: 'value', - - // 一些组件是 v-model:checked 或者 v-model:fileList + // naive-ui组件不接受onChang事件,所以需要禁用 + disabledOnChangeListener: true, + // naive-ui组件的空值为null,不能是undefined,否则重置表单时不生效 + emptyStateValue: null, modelPropNameMap: { Checkbox: 'checked', Radio: 'checked', @@ -102,14 +26,12 @@ setupVbenForm({ }, }, defineRules: { - // 输入项目必填国际化适配 required: (value, _params, ctx) => { if (value === undefined || value === null || value.length === 0) { return $t('formRules.required', [ctx.label]); } return true; }, - // 选择项目必填国际化适配 selectRequired: (value, _params, ctx) => { if (value === undefined || value === null) { return $t('formRules.selectRequired', [ctx.label]); @@ -119,9 +41,9 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; diff --git a/docs/src/commercial/community.md b/docs/src/commercial/community.md index b1033454..899d030d 100644 --- a/docs/src/commercial/community.md +++ b/docs/src/commercial/community.md @@ -3,7 +3,7 @@ 社区交流群主要是为了方便大家交流,提问,解答问题,分享经验等。偏自助方式,如果你有问题,可以通过以下方式加入社区交流群: - [QQ频道](https://pd.qq.com/s/16p8lvvob):推荐!!!主要提供问题解答,分享经验等。 -- QQ群:[1群](https://qm.qq.com/q/YacMHPYAMu)、[2群](https://qm.qq.com/q/ajVKZvFICk)、[3群](https://qm.qq.com/q/36zdwThP2E),[4群](https://qm.qq.com/q/sCzSlm3504),[老群](https://qm.qq.com/q/MEmHoCLbG0),主要使用者的交流群。 +- QQ群:[大群](https://qm.qq.com/q/MEmHoCLbG0),[1群](https://qm.qq.com/q/YacMHPYAMu)、[2群](https://qm.qq.com/q/ajVKZvFICk)、[3群](https://qm.qq.com/q/36zdwThP2E),[4群](https://qm.qq.com/q/sCzSlm3504),主要使用者的交流群。 - [Discord](https://discord.com/invite/VU62jTecad): 主要提供问题解答,分享经验等。 ::: tip diff --git a/docs/src/components/common-ui/vben-drawer.md b/docs/src/components/common-ui/vben-drawer.md index cbd92f5d..070e80ab 100644 --- a/docs/src/components/common-ui/vben-drawer.md +++ b/docs/src/components/common-ui/vben-drawer.md @@ -14,6 +14,12 @@ outline: deep ::: +::: tip README + +下方示例代码中的,存在一些国际化、主题色未适配问题,这些问题只在文档内会出现,实际使用并不会有这些问题,可忽略,不必纠结。 + +::: + ## 基础用法 使用 `useVbenDrawer` 创建最基础的模态框。 diff --git a/docs/src/components/common-ui/vben-form.md b/docs/src/components/common-ui/vben-form.md index ccb438c9..e9ca7666 100644 --- a/docs/src/components/common-ui/vben-form.md +++ b/docs/src/components/common-ui/vben-form.md @@ -20,116 +20,23 @@ outline: deep ### 适配器说明 -每个应用都有不同的 UI 框架,所以在应用的 `src/adapter/form` 内部,你可以根据自己的需求,进行组件适配。下面是 `Ant Design Vue` 的适配器示例代码,可根据注释查看说明: +每个应用都有不同的 UI 框架,所以在应用的 `src/adapter/form` 和 `src/adapter/component` 内部,你可以根据自己的需求,进行组件适配。下面是 `Ant Design Vue` 的适配器示例代码,可根据注释查看说明: -::: details ant design 适配器 +::: details ant design vue 表单适配器 ```ts import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import type { Component, SetupContext } from 'vue'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - AutoComplete, - Button, - Checkbox, - CheckboxGroup, - DatePicker, - Divider, - Input, - InputNumber, - InputPassword, - Mentions, - Radio, - RadioGroup, - RangePicker, - Rate, - Select, - Space, - Switch, - Textarea, - TimePicker, - TreeSelect, - Upload, -} from 'ant-design-vue'; - -// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 -export type FormComponentType = - | 'AutoComplete' - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'InputPassword' - | 'Mentions' - | 'Radio' - | 'RadioGroup' - | 'RangePicker' - | 'Rate' - | 'Select' - | 'Space' - | 'Switch' - | 'Textarea' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -const withDefaultPlaceholder = ( - component: T, - type: 'input' | 'select', -) => { - return (props: any, { attrs, slots }: Omit) => { - const placeholder = props?.placeholder || $t(`placeholder.${type}`); - return h(component, { ...props, ...attrs, placeholder }, slots); - }; -}; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - AutoComplete, - Checkbox, - CheckboxGroup, - DatePicker, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'default' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'primary' }, slots); - }, - Divider, - Input: withDefaultPlaceholder(Input, 'input'), - InputNumber: withDefaultPlaceholder(InputNumber, 'input'), - InputPassword: withDefaultPlaceholder(InputPassword, 'input'), - Mentions: withDefaultPlaceholder(Mentions, 'input'), - Radio, - RadioGroup, - RangePicker, - Rate, - Select: withDefaultPlaceholder(Select, 'select'), - Space, - Switch, - Textarea: withDefaultPlaceholder(Textarea, 'input'), - TimePicker, - TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), - Upload, - }, +setupVbenForm({ config: { - // 是否禁用onChange事件监听,naive ui组件库默认不需要监听onChange事件,否则会在控制台报错 - disabledOnChangeListener: true, // ant design vue组件库默认都是 v-model:value baseModelPropName: 'value', // 一些组件是 v-model:checked 或者 v-model:fileList @@ -158,16 +65,149 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; - -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; ``` ::: +::: details ant design vue 组件适配器 + +```ts +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + AutoComplete, + Button, + Checkbox, + CheckboxGroup, + DatePicker, + Divider, + Input, + InputNumber, + InputPassword, + Mentions, + notification, + Radio, + RadioGroup, + RangePicker, + Rate, + Select, + Space, + Switch, + Textarea, + TimePicker, + TreeSelect, + Upload, +} from 'ant-design-vue'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'AutoComplete' + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'DefaultButton' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'InputPassword' + | 'Mentions' + | 'PrimaryButton' + | 'Radio' + | 'RadioGroup' + | 'RangePicker' + | 'Rate' + | 'Select' + | 'Space' + | 'Switch' + | 'Textarea' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + AutoComplete, + Checkbox, + CheckboxGroup, + DatePicker, + // 自定义默认按钮 + DefaultButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'default' }, slots); + }, + Divider, + Input: withDefaultPlaceholder(Input, 'input'), + InputNumber: withDefaultPlaceholder(InputNumber, 'input'), + InputPassword: withDefaultPlaceholder(InputPassword, 'input'), + Mentions: withDefaultPlaceholder(Mentions, 'input'), + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'primary' }, slots); + }, + Radio, + RadioGroup, + RangePicker, + Rate, + Select: withDefaultPlaceholder(Select, 'select'), + Space, + Switch, + Textarea: withDefaultPlaceholder(Textarea, 'input'), + TimePicker, + TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), + Upload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + notification.success({ + description: content, + message: title, + placement: 'bottomRight', + }); + }, + }); +} + +export { initComponentAdapter }; +``` + +::: + ## 基础用法 ::: tip README diff --git a/docs/src/components/common-ui/vben-modal.md b/docs/src/components/common-ui/vben-modal.md index 26389fc6..487e5e8a 100644 --- a/docs/src/components/common-ui/vben-modal.md +++ b/docs/src/components/common-ui/vben-modal.md @@ -14,6 +14,12 @@ outline: deep ::: +::: tip README + +下方示例代码中的,存在一些国际化、主题色未适配问题,这些问题只在文档内会出现,实际使用并不会有这些问题,可忽略,不必纠结。 + +::: + ## 基础用法 使用 `useVbenModal` 创建最基础的模态框。 diff --git a/docs/src/components/common-ui/vben-vxe-table.md b/docs/src/components/common-ui/vben-vxe-table.md new file mode 100644 index 00000000..e6f6a8f0 --- /dev/null +++ b/docs/src/components/common-ui/vben-vxe-table.md @@ -0,0 +1,7 @@ +--- +outline: deep +--- + +# Vben Vxe Table 表格 + +TODO diff --git a/docs/src/friend-links/index.md b/docs/src/friend-links/index.md index 59de06c0..84b61906 100644 --- a/docs/src/friend-links/index.md +++ b/docs/src/friend-links/index.md @@ -1,6 +1,6 @@ # 友情链接 -如果您的网站是与 Vben Admin 相关的,或者也属于开源项目,欢迎联系我们,我们会将与您的网站加入交换友情链接。 +如果您的网站是与 Vben Admin 相关的,也属于开源项目,欢迎联系我们,我们会将与您的网站加入交换友情链接。 ## 交换方式 diff --git a/packages/@core/base/design/src/css/global.css b/packages/@core/base/design/src/css/global.css index 8881d3df..2cee217f 100644 --- a/packages/@core/base/design/src/css/global.css +++ b/packages/@core/base/design/src/css/global.css @@ -94,7 +94,6 @@ } /* 只有非mac下才进行调整,mac下使用默认滚动条 */ - html:not([data-platform='macOs']) { ::-webkit-scrollbar { @apply h-[10px] w-[10px]; diff --git a/packages/@core/base/design/src/design-tokens/dark/index.css b/packages/@core/base/design/src/design-tokens/dark.css similarity index 100% rename from packages/@core/base/design/src/design-tokens/dark/index.css rename to packages/@core/base/design/src/design-tokens/dark.css diff --git a/packages/@core/base/design/src/design-tokens/default/index.css b/packages/@core/base/design/src/design-tokens/default.css similarity index 100% rename from packages/@core/base/design/src/design-tokens/default/index.css rename to packages/@core/base/design/src/design-tokens/default.css diff --git a/packages/@core/base/design/src/design-tokens/index.ts b/packages/@core/base/design/src/design-tokens/index.ts index 9d27b4e8..2d031d81 100644 --- a/packages/@core/base/design/src/design-tokens/index.ts +++ b/packages/@core/base/design/src/design-tokens/index.ts @@ -1,4 +1,4 @@ -import './default/index.css'; -import './dark/index.css'; +import './default.css'; +import './dark.css'; export {}; diff --git a/packages/@core/base/shared/build.config.ts b/packages/@core/base/shared/build.config.ts index 9406af74..98e2209f 100644 --- a/packages/@core/base/shared/build.config.ts +++ b/packages/@core/base/shared/build.config.ts @@ -9,5 +9,6 @@ export default defineBuildConfig({ 'src/utils/index', 'src/color/index', 'src/cache/index', + 'src/global-state', ], }); diff --git a/packages/@core/base/shared/package.json b/packages/@core/base/shared/package.json index 54f151b3..134986fb 100644 --- a/packages/@core/base/shared/package.json +++ b/packages/@core/base/shared/package.json @@ -11,7 +11,8 @@ "license": "MIT", "type": "module", "scripts": { - "build": "pnpm unbuild" + "build": "pnpm unbuild", + "stub": "pnpm unbuild --stub" }, "files": [ "dist" @@ -42,6 +43,11 @@ "types": "./src/store.ts", "development": "./src/store.ts", "default": "./dist/store.mjs" + }, + "./global-state": { + "types": "./dist/global-state.d.ts", + "development": "./src/global-state.ts", + "default": "./dist/global-state.mjs" } }, "publishConfig": { @@ -63,8 +69,12 @@ "default": "./dist/cache/index.mjs" }, "./store": { - "types": "./dist/store/index.d.ts", + "types": "./dist/store.d.ts", "default": "./dist/store.mjs" + }, + "./global-state": { + "types": "./dist/global-state.d.ts", + "default": "./dist/global-state.mjs" } } }, diff --git a/packages/@core/base/shared/src/global-state.ts b/packages/@core/base/shared/src/global-state.ts new file mode 100644 index 00000000..2d71356b --- /dev/null +++ b/packages/@core/base/shared/src/global-state.ts @@ -0,0 +1,45 @@ +/** + * 全局复用的变量、组件、配置,各个模块之间共享 + * 通过单例模式实现,单例必须注意不受请求影响,例如用户信息这些需要根据请求获取的。后续如果有ssr需求,也不会影响 + */ + +interface ComponentsState { + [key: string]: any; +} + +interface MessageState { + copyPreferencesSuccess?: (title: string, content?: string) => void; +} + +export interface IGlobalSharedState { + components: ComponentsState; + message: MessageState; +} + +class GlobalShareState { + #components: ComponentsState = {}; + #message: MessageState = {}; + + /** + * 定义框架内部各个场景的消息提示 + */ + public defineMessage({ copyPreferencesSuccess }: MessageState) { + this.#message = { + copyPreferencesSuccess, + }; + } + + public getComponents(): ComponentsState { + return this.#components; + } + + public getMessage(): MessageState { + return this.#message; + } + + public setComponents(value: ComponentsState) { + this.#components = value; + } +} + +export const globalShareState = new GlobalShareState(); diff --git a/packages/@core/composables/src/use-sortable.ts b/packages/@core/composables/src/use-sortable.ts index 6659db83..57f87a64 100644 --- a/packages/@core/composables/src/use-sortable.ts +++ b/packages/@core/composables/src/use-sortable.ts @@ -10,13 +10,6 @@ function useSortable( // @ts-expect-error - This is a dynamic import 'sortablejs/modular/sortable.complete.esm.js' ); - // const { AutoScroll } = await import( - // // @ts-expect-error - This is a dynamic import - // 'sortablejs/modular/sortable.core.esm.js' - // ); - - // Sortable?.default?.mount?.(AutoScroll); - const sortable = Sortable?.default?.create?.(sortableContainer, { animation: 300, delay: 400, diff --git a/packages/@core/ui-kit/form-ui/src/components/form-actions.vue b/packages/@core/ui-kit/form-ui/src/components/form-actions.vue index 0ac5b24e..3e08b471 100644 --- a/packages/@core/ui-kit/form-ui/src/components/form-actions.vue +++ b/packages/@core/ui-kit/form-ui/src/components/form-actions.vue @@ -84,7 +84,7 @@ watch( :style="queryFormStyle" > = { - DefaultResetActionButton: h(VbenButton, { size: 'sm', variant: 'outline' }), - DefaultSubmitActionButton: h(VbenButton, { size: 'sm', variant: 'default' }), + DefaultButton: h(VbenButton, { size: 'sm', variant: 'outline' }), + PrimaryButton: h(VbenButton, { size: 'sm', variant: 'default' }), VbenCheckbox, VbenInput, VbenInputPassword, @@ -41,7 +42,7 @@ export const COMPONENT_BIND_EVENT_MAP: Partial< export function setupVbenForm< T extends BaseFormComponentType = BaseFormComponentType, >(options: VbenFormAdapterOptions) { - const { components, config, defineRules } = options; + const { config, defineRules } = options; const { disabledOnChangeListener = false, emptyStateValue = undefined } = (config || {}) as FormCommonConfig; @@ -63,6 +64,8 @@ export function setupVbenForm< | Record | undefined; + const components = globalShareState.getComponents(); + for (const component of Object.keys(components)) { const key = component as BaseFormComponentType; COMPONENT_MAP[key] = components[component as never]; diff --git a/packages/@core/ui-kit/form-ui/src/types.ts b/packages/@core/ui-kit/form-ui/src/types.ts index 8f082545..3463b779 100644 --- a/packages/@core/ui-kit/form-ui/src/types.ts +++ b/packages/@core/ui-kit/form-ui/src/types.ts @@ -9,8 +9,8 @@ import type { Component, HtmlHTMLAttributes, Ref } from 'vue'; export type FormLayout = 'horizontal' | 'vertical'; export type BaseFormComponentType = - | 'DefaultResetActionButton' - | 'DefaultSubmitActionButton' + | 'DefaultButton' + | 'PrimaryButton' | 'VbenCheckbox' | 'VbenInput' | 'VbenInputPassword' @@ -341,7 +341,6 @@ export type ExtendedFormApi = { export interface VbenFormAdapterOptions< T extends BaseFormComponentType = BaseFormComponentType, > { - components: Partial>; config?: { baseModelPropName?: string; disabledOnChangeListener?: boolean; diff --git a/packages/@core/ui-kit/popup-ui/src/drawer/drawer.vue b/packages/@core/ui-kit/popup-ui/src/drawer/drawer.vue index 4f80f9e5..4aed25f0 100644 --- a/packages/@core/ui-kit/popup-ui/src/drawer/drawer.vue +++ b/packages/@core/ui-kit/popup-ui/src/drawer/drawer.vue @@ -23,6 +23,7 @@ import { VbenLoading, VisuallyHidden, } from '@vben-core/shadcn-ui'; +import { globalShareState } from '@vben-core/shared/global-state'; import { cn } from '@vben-core/shared/utils'; interface Props extends DrawerProps { @@ -33,6 +34,8 @@ const props = withDefaults(defineProps(), { drawerApi: undefined, }); +const components = globalShareState.getComponents(); + const id = useId(); provide('DISMISSABLE_DRAWER_ID', id); @@ -187,7 +190,8 @@ function handleFocusOutside(e: Event) { > - drawerApi?.onCancel()" @@ -195,8 +199,10 @@ function handleFocusOutside(e: Event) { {{ cancelText || $t('cancel') }} - - + + drawerApi?.onConfirm()" @@ -204,7 +210,7 @@ function handleFocusOutside(e: Event) { {{ confirmText || $t('confirm') }} - + diff --git a/packages/@core/ui-kit/popup-ui/src/modal/modal.vue b/packages/@core/ui-kit/popup-ui/src/modal/modal.vue index 0413ef6d..5d409b94 100644 --- a/packages/@core/ui-kit/popup-ui/src/modal/modal.vue +++ b/packages/@core/ui-kit/popup-ui/src/modal/modal.vue @@ -22,6 +22,7 @@ import { VbenLoading, VisuallyHidden, } from '@vben-core/shadcn-ui'; +import { globalShareState } from '@vben-core/shared/global-state'; import { cn } from '@vben-core/shared/utils'; import { useModalDraggable } from './use-modal-draggable'; @@ -34,6 +35,8 @@ const props = withDefaults(defineProps(), { modalApi: undefined, }); +const components = globalShareState.getComponents(); + const contentRef = ref(); const wrapperRef = ref(); const dialogRef = ref(); @@ -256,7 +259,8 @@ function handleFocusOutside(e: Event) { > - modalApi?.onCancel()" @@ -264,8 +268,10 @@ function handleFocusOutside(e: Event) { {{ cancelText || $t('cancel') }} - - + + modalApi?.onConfirm()" @@ -273,7 +279,7 @@ function handleFocusOutside(e: Event) { {{ confirmText || $t('confirm') }} - + diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/index.ts b/packages/@core/ui-kit/shadcn-ui/src/ui/index.ts index d9fca452..2711f942 100644 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/index.ts +++ b/packages/@core/ui-kit/shadcn-ui/src/ui/index.ts @@ -23,7 +23,6 @@ export * from './sheet'; export * from './switch'; export * from './tabs'; export * from './textarea'; -export * from './toast'; export * from './toggle'; export * from './toggle-group'; export * from './tooltip'; diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toast.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toast.vue deleted file mode 100644 index a5b081ef..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toast.vue +++ /dev/null @@ -1,35 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastAction.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastAction.vue deleted file mode 100644 index f49edabe..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastAction.vue +++ /dev/null @@ -1,29 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastClose.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastClose.vue deleted file mode 100644 index 9112f95e..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastClose.vue +++ /dev/null @@ -1,34 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastDescription.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastDescription.vue deleted file mode 100644 index c74697f2..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastDescription.vue +++ /dev/null @@ -1,24 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastProvider.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastProvider.vue deleted file mode 100644 index 9e2194fc..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastProvider.vue +++ /dev/null @@ -1,11 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastTitle.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastTitle.vue deleted file mode 100644 index 93a261c5..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastTitle.vue +++ /dev/null @@ -1,24 +0,0 @@ - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastViewport.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastViewport.vue deleted file mode 100644 index d71527b6..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/ToastViewport.vue +++ /dev/null @@ -1,27 +0,0 @@ - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toaster.vue b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toaster.vue deleted file mode 100644 index 52c4a244..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/Toaster.vue +++ /dev/null @@ -1,36 +0,0 @@ - - - - - - - - {{ toast.title }} - - - - - - - {{ toast.description }} - - - - - - - - - diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/index.ts b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/index.ts deleted file mode 100644 index 151e820a..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/index.ts +++ /dev/null @@ -1,11 +0,0 @@ -export * from './toast'; -export { default as Toast } from './Toast.vue'; -export { default as ToastAction } from './ToastAction.vue'; -export { default as ToastClose } from './ToastClose.vue'; -export { default as ToastDescription } from './ToastDescription.vue'; -export { default as Toaster } from './Toaster.vue'; -export { default as ToastProvider } from './ToastProvider.vue'; -export { default as ToastTitle } from './ToastTitle.vue'; -export { default as ToastViewport } from './ToastViewport.vue'; - -export { toast, useToast } from './use-toast'; diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/toast.ts b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/toast.ts deleted file mode 100644 index 92f9737b..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/toast.ts +++ /dev/null @@ -1,27 +0,0 @@ -import type { ToastRootProps } from 'radix-vue'; - -import { cva, type VariantProps } from 'class-variance-authority'; - -export const toastVariants = cva( - 'group pointer-events-auto relative flex w-full items-center justify-between space-x-2 overflow-hidden rounded-md border border-border p-4 pr-6 shadow-lg transition-all data-[swipe=cancel]:translate-x-0 data-[swipe=end]:translate-x-[var(--radix-toast-swipe-end-x)] data-[swipe=move]:translate-x-[var(--radix-toast-swipe-move-x)] data-[swipe=move]:transition-none data-[state=open]:animate-in data-[state=closed]:animate-out data-[swipe=end]:animate-out data-[state=closed]:fade-out-80 data-[state=closed]:slide-out-to-right-full data-[state=open]:slide-in-from-top-full data-[state=open]:sm:slide-in-from-bottom-full', - { - defaultVariants: { - variant: 'default', - }, - variants: { - variant: { - default: 'border bg-background border-border text-foreground', - destructive: - 'destructive group border-destructive bg-destructive text-destructive-foreground', - }, - }, - }, -); - -type ToastVariants = VariantProps; - -export interface ToastProps extends ToastRootProps { - class?: any; - onOpenChange?: ((value: boolean) => void) | undefined; - variant?: ToastVariants['variant']; -} diff --git a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/use-toast.ts b/packages/@core/ui-kit/shadcn-ui/src/ui/toast/use-toast.ts deleted file mode 100644 index 96ff92e4..00000000 --- a/packages/@core/ui-kit/shadcn-ui/src/ui/toast/use-toast.ts +++ /dev/null @@ -1,168 +0,0 @@ -import type { ToastProps } from './toast'; - -import { computed, ref } from 'vue'; -import type { Component, VNode } from 'vue'; - -const TOAST_LIMIT = 1; -const TOAST_REMOVE_DELAY = 1_000_000; - -export type StringOrVNode = (() => VNode) | string | VNode; - -type ToasterToast = { - action?: Component; - description?: StringOrVNode; - id: string; - title?: string; -} & ToastProps; - -const actionTypes = { - ADD_TOAST: 'ADD_TOAST', - DISMISS_TOAST: 'DISMISS_TOAST', - REMOVE_TOAST: 'REMOVE_TOAST', - UPDATE_TOAST: 'UPDATE_TOAST', -} as const; - -let count = 0; - -function genId() { - count = (count + 1) % Number.MAX_VALUE; - return count.toString(); -} - -type ActionType = typeof actionTypes; - -type Action = - | { - toast: Partial; - type: ActionType['UPDATE_TOAST']; - } - | { - toast: ToasterToast; - type: ActionType['ADD_TOAST']; - } - | { - toastId?: ToasterToast['id']; - type: ActionType['DISMISS_TOAST']; - } - | { - toastId?: ToasterToast['id']; - type: ActionType['REMOVE_TOAST']; - }; - -interface State { - toasts: ToasterToast[]; -} - -const toastTimeouts = new Map>(); - -function addToRemoveQueue(toastId: string) { - if (toastTimeouts.has(toastId)) return; - - const timeout = setTimeout(() => { - toastTimeouts.delete(toastId); - dispatch({ - toastId, - type: actionTypes.REMOVE_TOAST, - }); - }, TOAST_REMOVE_DELAY); - - toastTimeouts.set(toastId, timeout); -} - -const state = ref({ - toasts: [], -}); - -function dispatch(action: Action) { - switch (action.type) { - case actionTypes.ADD_TOAST: { - state.value.toasts = [action.toast, ...state.value.toasts].slice( - 0, - TOAST_LIMIT, - ); - break; - } - - case actionTypes.UPDATE_TOAST: { - state.value.toasts = state.value.toasts.map((t) => - t.id === action.toast.id ? { ...t, ...action.toast } : t, - ); - break; - } - - case actionTypes.DISMISS_TOAST: { - const { toastId } = action; - - if (toastId) { - addToRemoveQueue(toastId); - } else { - state.value.toasts.forEach((toast) => { - addToRemoveQueue(toast.id); - }); - } - - state.value.toasts = state.value.toasts.map((t) => - t.id === toastId || toastId === undefined - ? { - ...t, - open: false, - } - : t, - ); - break; - } - - case actionTypes.REMOVE_TOAST: { - state.value.toasts = - action.toastId === undefined - ? [] - : state.value.toasts.filter((t) => t.id !== action.toastId); - - break; - } - } -} - -function useToast() { - return { - dismiss: (toastId?: string) => - dispatch({ toastId, type: actionTypes.DISMISS_TOAST }), - toast, - toasts: computed(() => state.value.toasts), - }; -} - -type Toast = Omit; - -function toast(props: Toast) { - const id = genId(); - - const update = (props: ToasterToast) => - dispatch({ - toast: { ...props, id }, - type: actionTypes.UPDATE_TOAST, - }); - - const dismiss = () => - dispatch({ toastId: id, type: actionTypes.DISMISS_TOAST }); - - dispatch({ - toast: { - ...props, - id, - onOpenChange: (open: boolean) => { - if (!open) dismiss(); - }, - open: true, - }, - type: actionTypes.ADD_TOAST, - }); - - return { - dismiss, - id, - update, - }; -} - -export { toast, useToast }; diff --git a/packages/effects/common-ui/src/components/index.ts b/packages/effects/common-ui/src/components/index.ts index fc5825e2..12d1cace 100644 --- a/packages/effects/common-ui/src/components/index.ts +++ b/packages/effects/common-ui/src/components/index.ts @@ -13,3 +13,5 @@ export { VbenPinInput, VbenSpinner, } from '@vben-core/shadcn-ui'; + +export { globalShareState } from '@vben-core/shared/global-state'; diff --git a/packages/effects/common-ui/src/index.ts b/packages/effects/common-ui/src/index.ts index 050c0829..27bd7186 100644 --- a/packages/effects/common-ui/src/index.ts +++ b/packages/effects/common-ui/src/index.ts @@ -1,3 +1,2 @@ export * from './components'; export * from './ui'; -export { useToast } from '@vben-core/shadcn-ui'; diff --git a/packages/effects/layouts/src/basic/layout.vue b/packages/effects/layouts/src/basic/layout.vue index 8b9933c5..0305eed4 100644 --- a/packages/effects/layouts/src/basic/layout.vue +++ b/packages/effects/layouts/src/basic/layout.vue @@ -13,7 +13,7 @@ import { import { useLockStore } from '@vben/stores'; import { deepToRaw, mapTree } from '@vben/utils'; import { VbenAdminLayout } from '@vben-core/layout-ui'; -import { Toaster, VbenBackTop, VbenLogo } from '@vben-core/shadcn-ui'; +import { VbenBackTop, VbenLogo } from '@vben-core/shadcn-ui'; import { Breadcrumb, CheckUpdates, Preferences } from '../widgets'; import { LayoutContent, LayoutContentSpinner } from './content'; @@ -312,7 +312,6 @@ const headerSlots = computed(() => { - -import { h, onMounted, onUnmounted, ref } from 'vue'; +import { onMounted, onUnmounted, ref } from 'vue'; import { $t } from '@vben/locales'; -import { ToastAction, useToast } from '@vben-core/shadcn-ui'; +import { useVbenModal } from '@vben-core/popup-ui'; interface Props { // 轮训时间,分钟 @@ -18,10 +18,21 @@ const props = withDefaults(defineProps(), { checkUpdateUrl: import.meta.env.BASE_URL || '/', }); -const lastVersionTag = ref(''); let isCheckingUpdates = false; +const currentVersionTag = ref(''); +const lastVersionTag = ref(''); const timer = ref>(); -const { toast } = useToast(); + +const [UpdateNoticeModal, modalApi] = useVbenModal({ + closable: false, + closeOnPressEscape: false, + closeOnClickModal: false, + onConfirm() { + lastVersionTag.value = currentVersionTag.value; + window.location.reload(); + // handleSubmitLogout(); + }, +}); async function getVersionTag() { try { @@ -63,38 +74,8 @@ async function checkForUpdates() { } } function handleNotice(versionTag: string) { - const { dismiss } = toast({ - action: h('div', { class: 'inline-flex items-center' }, [ - h( - ToastAction, - { - altText: $t('common.cancel'), - onClick: () => dismiss(), - }, - { - default: () => $t('common.cancel'), - }, - ), - h( - ToastAction, - { - altText: $t('common.refresh'), - class: - 'bg-primary text-primary-foreground hover:bg-primary-hover mx-1', - onClick: () => { - lastVersionTag.value = versionTag; - window.location.reload(); - }, - }, - { - default: () => $t('common.refresh'), - }, - ), - ]), - description: $t('widgets.checkUpdatesDescription'), - duration: 0, - title: $t('widgets.checkUpdatesTitle'), - }); + currentVersionTag.value = versionTag; + modalApi.open(); } function start() { @@ -138,5 +119,16 @@ onUnmounted(() => { }); - + + {{ $t('widgets.checkUpdatesDescription') }} + diff --git a/packages/effects/layouts/src/widgets/preferences/preferences-drawer.vue b/packages/effects/layouts/src/widgets/preferences/preferences-drawer.vue index 1cece3c1..77bf4d70 100644 --- a/packages/effects/layouts/src/widgets/preferences/preferences-drawer.vue +++ b/packages/effects/layouts/src/widgets/preferences/preferences-drawer.vue @@ -24,11 +24,11 @@ import { } from '@vben/preferences'; import { useVbenDrawer } from '@vben-core/popup-ui'; import { - useToast, VbenButton, VbenIconButton, VbenSegmented, } from '@vben-core/shadcn-ui'; +import { globalShareState } from '@vben-core/shared/global-state'; import { useClipboard } from '@vueuse/core'; @@ -54,7 +54,9 @@ import { } from './blocks'; const emit = defineEmits<{ clearPreferencesAndLogout: [] }>(); -const { toast } = useToast(); + +const message = globalShareState.getMessage(); + const appLocale = defineModel('appLocale'); const appDynamicTitle = defineModel('appDynamicTitle'); const appLayout = defineModel('appLayout'); @@ -196,10 +198,10 @@ const showBreadcrumbConfig = computed(() => { async function handleCopy() { await copy(JSON.stringify(diffPreference.value, null, 2)); - toast({ - description: $t('preferences.copyPreferences'), - title: $t('preferences.copyPreferencesSuccess'), - }); + message.copyPreferencesSuccess?.( + $t('preferences.copyPreferencesSuccessTitle'), + $t('preferences.copyPreferencesSuccess'), + ); } async function handleClearCache() { @@ -214,10 +216,6 @@ async function handleReset() { } resetPreferences(); await loadLocaleMessages(preferences.app.locale); - toast({ - description: $t('preferences.resetTitle'), - title: $t('preferences.resetSuccess'), - }); } diff --git a/packages/effects/layouts/src/widgets/user-dropdown/user-dropdown.vue b/packages/effects/layouts/src/widgets/user-dropdown/user-dropdown.vue index fe52c0e0..2cfa0ed3 100644 --- a/packages/effects/layouts/src/widgets/user-dropdown/user-dropdown.vue +++ b/packages/effects/layouts/src/widgets/user-dropdown/user-dropdown.vue @@ -149,7 +149,7 @@ if (enableShortcutKey.value) { :title="$t('common.prompt')" centered content-class="px-8 min-h-10" - footer-class="border-none mb-4 mr-4" + footer-class="border-none mb-3 mr-3" header-class="border-none" > {{ $t('widgets.logoutTip') }} diff --git a/packages/locales/src/i18n.ts b/packages/locales/src/i18n.ts index c0cc3159..b44fcfa6 100644 --- a/packages/locales/src/i18n.ts +++ b/packages/locales/src/i18n.ts @@ -35,7 +35,7 @@ function loadLocalesMap(modules: Record Promise>) { const localesMap: Record = {}; for (const [path, loadLocale] of Object.entries(modules)) { - const key = path.match(/([\w-]*)\.(yaml|yml|json)/)?.[1]; + const key = path.match(/([\w-]*)\.(json)/)?.[1]; if (key) { localesMap[key] = loadLocale as ImportLocaleFn; } diff --git a/packages/locales/src/langs/en-US.json b/packages/locales/src/langs/en-US.json index 32ec7388..2f303c23 100644 --- a/packages/locales/src/langs/en-US.json +++ b/packages/locales/src/langs/en-US.json @@ -178,6 +178,7 @@ "plain": "Plain", "rounded": "Rounded", "copyPreferences": "Copy Preferences", + "copyPreferencesSuccessTitle": "Copy successful", "copyPreferencesSuccess": "Copy successful, please override in `src/preferences.ts` under app", "clearAndLogout": "Clear Cache & Logout", "mode": "Mode", diff --git a/packages/locales/src/langs/zh-CN.json b/packages/locales/src/langs/zh-CN.json index 713658ce..0fa5981a 100644 --- a/packages/locales/src/langs/zh-CN.json +++ b/packages/locales/src/langs/zh-CN.json @@ -178,6 +178,7 @@ "plain": "朴素", "rounded": "圆润", "copyPreferences": "复制偏好设置", + "copyPreferencesSuccessTitle": "复制成功", "copyPreferencesSuccess": "复制成功,请在 app 下的 `src/preferences.ts`内进行覆盖", "clearAndLogout": "清空缓存 & 退出登录", "mode": "模式", diff --git a/packages/stores/src/modules/tabbar.ts b/packages/stores/src/modules/tabbar.ts index 0c3850e7..80d8853c 100644 --- a/packages/stores/src/modules/tabbar.ts +++ b/packages/stores/src/modules/tabbar.ts @@ -523,7 +523,8 @@ function isAffixTab(tab: TabDefinition) { * @param tab */ function isTabShown(tab: TabDefinition) { - return !tab.meta.hideInTab; + const matched = tab?.matched ?? []; + return !tab.meta.hideInTab && matched.every((item) => !item.meta.hideInTab); } /** diff --git a/packages/stores/src/setup.ts b/packages/stores/src/setup.ts index 4febc892..890c4dc8 100644 --- a/packages/stores/src/setup.ts +++ b/packages/stores/src/setup.ts @@ -37,7 +37,6 @@ export function resetAllStores() { return; } const allStores = (pinia as any)._s; - for (const [_key, store] of allStores) { store.$reset(); } diff --git a/playground/src/adapter/component/index.ts b/playground/src/adapter/component/index.ts new file mode 100644 index 00000000..12849dc3 --- /dev/null +++ b/playground/src/adapter/component/index.ts @@ -0,0 +1,127 @@ +/** + * 通用组件共同的使用的基础组件,原先放在 adapter/form 内部,限制了使用范围,这里提取出来,方便其他地方使用 + * 可用于 vben-form、vben-modal、vben-drawer 等组件使用, + */ + +import type { BaseFormComponentType } from '@vben/common-ui'; + +import type { Component, SetupContext } from 'vue'; +import { h } from 'vue'; + +import { globalShareState } from '@vben/common-ui'; +import { $t } from '@vben/locales'; + +import { + AutoComplete, + Button, + Checkbox, + CheckboxGroup, + DatePicker, + Divider, + Input, + InputNumber, + InputPassword, + Mentions, + notification, + Radio, + RadioGroup, + RangePicker, + Rate, + Select, + Space, + Switch, + Textarea, + TimePicker, + TreeSelect, + Upload, +} from 'ant-design-vue'; + +const withDefaultPlaceholder = ( + component: T, + type: 'input' | 'select', +) => { + return (props: any, { attrs, slots }: Omit) => { + const placeholder = props?.placeholder || $t(`placeholder.${type}`); + return h(component, { ...props, ...attrs, placeholder }, slots); + }; +}; + +// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 +export type ComponentType = + | 'AutoComplete' + | 'Checkbox' + | 'CheckboxGroup' + | 'DatePicker' + | 'DefaultButton' + | 'Divider' + | 'Input' + | 'InputNumber' + | 'InputPassword' + | 'Mentions' + | 'PrimaryButton' + | 'Radio' + | 'RadioGroup' + | 'RangePicker' + | 'Rate' + | 'Select' + | 'Space' + | 'Switch' + | 'Textarea' + | 'TimePicker' + | 'TreeSelect' + | 'Upload' + | BaseFormComponentType; + +async function initComponentAdapter() { + const components: Partial> = { + // 如果你的组件体积比较大,可以使用异步加载 + // Button: () => + // import('xxx').then((res) => res.Button), + + AutoComplete, + Checkbox, + CheckboxGroup, + DatePicker, + // 自定义默认按钮 + DefaultButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'default' }, slots); + }, + Divider, + Input: withDefaultPlaceholder(Input, 'input'), + InputNumber: withDefaultPlaceholder(InputNumber, 'input'), + InputPassword: withDefaultPlaceholder(InputPassword, 'input'), + Mentions: withDefaultPlaceholder(Mentions, 'input'), + // 自定义主要按钮 + PrimaryButton: (props, { attrs, slots }) => { + return h(Button, { ...props, attrs, type: 'primary' }, slots); + }, + Radio, + RadioGroup, + RangePicker, + Rate, + Select: withDefaultPlaceholder(Select, 'select'), + Space, + Switch, + Textarea: withDefaultPlaceholder(Textarea, 'input'), + TimePicker, + TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), + Upload, + }; + + // 将组件注册到全局共享状态中 + globalShareState.setComponents(components); + + // 定义全局共享状态中的消息提示 + globalShareState.defineMessage({ + // 复制成功消息提示 + copyPreferencesSuccess: (title, content) => { + notification.success({ + description: content, + message: title, + placement: 'bottomRight', + }); + }, + }); +} + +export { initComponentAdapter }; diff --git a/playground/src/adapter/form.ts b/playground/src/adapter/form.ts index 48c981f7..2bc7777d 100644 --- a/playground/src/adapter/form.ts +++ b/playground/src/adapter/form.ts @@ -1,109 +1,17 @@ import type { - BaseFormComponentType, VbenFormSchema as FormSchema, VbenFormProps, } from '@vben/common-ui'; -import type { Component, SetupContext } from 'vue'; -import { h } from 'vue'; +import type { ComponentType } from './component'; import { setupVbenForm, useVbenForm as useForm, z } from '@vben/common-ui'; import { $t } from '@vben/locales'; -import { - AutoComplete, - Button, - Checkbox, - CheckboxGroup, - DatePicker, - Divider, - Input, - InputNumber, - InputPassword, - Mentions, - Radio, - RadioGroup, - RangePicker, - Rate, - Select, - Space, - Switch, - Textarea, - TimePicker, - TreeSelect, - Upload, -} from 'ant-design-vue'; - -// 这里需要自行根据业务组件库进行适配,需要用到的组件都需要在这里类型说明 -export type FormComponentType = - | 'AutoComplete' - | 'Checkbox' - | 'CheckboxGroup' - | 'DatePicker' - | 'Divider' - | 'Input' - | 'InputNumber' - | 'InputPassword' - | 'Mentions' - | 'Radio' - | 'RadioGroup' - | 'RangePicker' - | 'Rate' - | 'Select' - | 'Space' - | 'Switch' - | 'Textarea' - | 'TimePicker' - | 'TreeSelect' - | 'Upload' - | BaseFormComponentType; - -const withDefaultPlaceholder = ( - component: T, - type: 'input' | 'select', -) => { - return (props: any, { attrs, slots }: Omit) => { - const placeholder = props?.placeholder || $t(`placeholder.${type}`); - return h(component, { ...props, ...attrs, placeholder }, slots); - }; -}; - -// 初始化表单组件,并注册到form组件内部 -setupVbenForm({ - components: { - AutoComplete, - Checkbox, - CheckboxGroup, - DatePicker, - // 自定义默认的重置按钮 - DefaultResetActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'default' }, slots); - }, - // 自定义默认的提交按钮 - DefaultSubmitActionButton: (props, { attrs, slots }) => { - return h(Button, { ...props, attrs, type: 'primary' }, slots); - }, - Divider, - Input: withDefaultPlaceholder(Input, 'input'), - InputNumber: withDefaultPlaceholder(InputNumber, 'input'), - InputPassword: withDefaultPlaceholder(InputPassword, 'input'), - Mentions: withDefaultPlaceholder(Mentions, 'input'), - Radio, - RadioGroup, - RangePicker, - Rate, - Select: withDefaultPlaceholder(Select, 'select'), - Space, - Switch, - Textarea: withDefaultPlaceholder(Textarea, 'input'), - TimePicker, - TreeSelect: withDefaultPlaceholder(TreeSelect, 'select'), - Upload, - }, +setupVbenForm({ config: { // ant design vue组件库默认都是 v-model:value baseModelPropName: 'value', - // 一些组件是 v-model:checked 或者 v-model:fileList modelPropNameMap: { Checkbox: 'checked', @@ -130,9 +38,8 @@ setupVbenForm({ }, }); -const useVbenForm = useForm; +const useVbenForm = useForm; export { useVbenForm, z }; - -export type VbenFormSchema = FormSchema; +export type VbenFormSchema = FormSchema; export type { VbenFormProps }; diff --git a/playground/src/bootstrap.ts b/playground/src/bootstrap.ts index 6dac2709..b522068c 100644 --- a/playground/src/bootstrap.ts +++ b/playground/src/bootstrap.ts @@ -9,10 +9,14 @@ import { VueQueryPlugin } from '@tanstack/vue-query'; import { setupI18n } from '#/locales'; +import { initComponentAdapter } from './adapter/component'; import App from './app.vue'; import { router } from './router'; async function bootstrap(namespace: string) { + // 初始化组件适配器 + await initComponentAdapter(); + const app = createApp(App); // 国际化 i18n 配置 diff --git a/playground/src/router/routes/core.ts b/playground/src/router/routes/core.ts index f793a57e..d85de5a4 100644 --- a/playground/src/router/routes/core.ts +++ b/playground/src/router/routes/core.ts @@ -32,6 +32,7 @@ const coreRoutes: RouteRecordRaw[] = [ { component: AuthPageLayout, meta: { + hideInTab: true, title: 'Authentication', }, name: 'Authentication', diff --git a/pnpm-lock.yaml b/pnpm-lock.yaml index 8a19673f..75ed22e9 100644 --- a/pnpm-lock.yaml +++ b/pnpm-lock.yaml @@ -482,7 +482,7 @@ catalogs: version: 4.2.19 vxe-table: specifier: ^4.7.86 - version: 4.7.86 + version: 4.7.87 watermark-js-plus: specifier: ^1.5.7 version: 1.5.7 @@ -883,13 +883,13 @@ importers: dependencies: eslint-config-turbo: specifier: 'catalog:' - version: 2.1.3(eslint@9.12.0(jiti@2.2.1)) + version: 2.1.3(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-command: specifier: 'catalog:' - version: 0.2.6(eslint@9.12.0(jiti@2.2.1)) + version: 0.2.6(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-import-x: specifier: 'catalog:' - version: 4.3.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + version: 4.3.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) devDependencies: '@eslint/js': specifier: 'catalog:' @@ -899,49 +899,49 @@ importers: version: 9.6.1 '@typescript-eslint/eslint-plugin': specifier: 'catalog:' - version: 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + version: 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) '@typescript-eslint/parser': specifier: 'catalog:' - version: 8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + version: 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) eslint: specifier: 'catalog:' - version: 9.12.0(jiti@2.2.1) + version: 9.12.0(jiti@2.3.3) eslint-plugin-eslint-comments: specifier: 'catalog:' - version: 3.2.0(eslint@9.12.0(jiti@2.2.1)) + version: 3.2.0(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-jsdoc: specifier: 'catalog:' - version: 50.3.2(eslint@9.12.0(jiti@2.2.1)) + version: 50.3.2(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-jsonc: specifier: 'catalog:' - version: 2.16.0(eslint@9.12.0(jiti@2.2.1)) + version: 2.16.0(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-n: specifier: 'catalog:' - version: 17.11.1(eslint@9.12.0(jiti@2.2.1)) + version: 17.11.1(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-no-only-tests: specifier: 'catalog:' version: 3.3.0 eslint-plugin-perfectionist: specifier: 'catalog:' - version: 3.8.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)(vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.2.1))) + version: 3.8.0(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)(vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.3.3))) eslint-plugin-prettier: specifier: 'catalog:' - version: 5.2.1(@types/eslint@9.6.1)(eslint@9.12.0(jiti@2.2.1))(prettier@3.3.3) + version: 5.2.1(@types/eslint@9.6.1)(eslint@9.12.0(jiti@2.3.3))(prettier@3.3.3) eslint-plugin-regexp: specifier: 'catalog:' - version: 2.6.0(eslint@9.12.0(jiti@2.2.1)) + version: 2.6.0(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-unicorn: specifier: 'catalog:' - version: 56.0.0(eslint@9.12.0(jiti@2.2.1)) + version: 56.0.0(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-unused-imports: specifier: 'catalog:' - version: 4.1.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1)) + version: 4.1.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3)) eslint-plugin-vitest: specifier: 'catalog:' - version: 0.5.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)(vitest@2.1.2(@types/node@22.7.5)(happy-dom@15.7.4)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)) + version: 0.5.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)(vitest@2.1.2(@types/node@22.7.5)(happy-dom@15.7.4)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)) eslint-plugin-vue: specifier: 'catalog:' - version: 9.29.0(eslint@9.12.0(jiti@2.2.1)) + version: 9.29.0(eslint@9.12.0(jiti@2.3.3)) globals: specifier: 'catalog:' version: 15.11.0 @@ -950,7 +950,7 @@ importers: version: 2.4.0 vue-eslint-parser: specifier: 'catalog:' - version: 9.4.3(eslint@9.12.0(jiti@2.2.1)) + version: 9.4.3(eslint@9.12.0(jiti@2.3.3)) internal/lint-configs/prettier-config: dependencies: @@ -1109,7 +1109,7 @@ importers: dependencies: '@intlify/unplugin-vue-i18n': specifier: 'catalog:' - version: 5.2.0(@vue/compiler-dom@3.5.12)(eslint@9.12.0(jiti@2.2.1))(rollup@4.24.0)(typescript@5.6.3)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3)) + version: 5.2.0(@vue/compiler-dom@3.5.12)(eslint@9.12.0(jiti@2.3.3))(rollup@4.24.0)(typescript@5.6.3)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3)) '@jspm/generator': specifier: 'catalog:' version: 2.3.1 @@ -1646,7 +1646,7 @@ importers: version: 4.2.19 vxe-table: specifier: 'catalog:' - version: 4.7.86 + version: 4.7.87 packages/effects/request: dependencies: @@ -1707,7 +1707,7 @@ importers: version: 2.2.2(typescript@5.6.3)(vue@3.5.12(typescript@5.6.3)) pinia-plugin-persistedstate: specifier: 'catalog:' - version: 4.1.1(pinia@2.2.2(typescript@5.6.3)(vue@3.5.12(typescript@5.6.3)))(rollup@3.29.5) + version: 4.1.1(pinia@2.2.2(typescript@5.6.3)(vue@3.5.12(typescript@5.6.3)))(rollup@4.24.0) vue: specifier: ^3.5.12 version: 3.5.12(typescript@5.6.3) @@ -1945,8 +1945,8 @@ packages: cpu: [arm64] os: [darwin] - '@ast-grep/napi-darwin-arm64@0.27.2': - resolution: {integrity: sha512-Rs4XH2z/j8zSZbazTeQZhRAEuKAdZMP9DnPIRNCXbvHZscWBs+iVMPw6XbJTbIQ1XsFzt4Pbwjidnm8gJxUXDw==} + '@ast-grep/napi-darwin-arm64@0.27.3': + resolution: {integrity: sha512-R+9cma8VikO74RLW3soO79KIorNKTvTU3kMAt9X+vSqkd8jkcVmQJ+9dcEfnas+1tPeeS7Uw7AoOhnyrKFvMXA==} engines: {node: '>= 10'} cpu: [arm64] os: [darwin] @@ -1957,8 +1957,8 @@ packages: cpu: [x64] os: [darwin] - '@ast-grep/napi-darwin-x64@0.27.2': - resolution: {integrity: sha512-kBIJS7tm+mmdsPVtX7LpDw3gNOwHmrFwGSmDIOpk/hT54aGvx+/R34VM1Niu4/D8iVkdl49laHpv2dTyZR922A==} + '@ast-grep/napi-darwin-x64@0.27.3': + resolution: {integrity: sha512-qfU+HTF0TIJ0UdyfzGg5qJxsugWtx+XC4QI125dB6FuXDrbeYG1NJJP6Epkf6OQl03zKEaIhU/LC0kLyZEbaHQ==} engines: {node: '>= 10'} cpu: [x64] os: [darwin] @@ -1970,15 +1970,15 @@ packages: os: [linux] libc: [glibc] - '@ast-grep/napi-linux-arm64-gnu@0.27.2': - resolution: {integrity: sha512-SI+DDHkGz23XiJVYvZ+m+PuURXKGlEHtuz3tNpA6QclEsNnCcOTkICtigafndGAgkx0oB4ehRtfR90zQR1jlEQ==} + '@ast-grep/napi-linux-arm64-gnu@0.27.3': + resolution: {integrity: sha512-0tb4PZ1jBGvH6E4YVwhDlVMQ5uuZ5c1D47skA0avl2HNH+OlK6wF4zAFPziI40PoBCztVkL8C+mETVpFWxba8A==} engines: {node: '>= 10'} cpu: [arm64] os: [linux] libc: [glibc] - '@ast-grep/napi-linux-arm64-musl@0.27.2': - resolution: {integrity: sha512-b2wAVcKJ3E23Ne7NyzBMwbtDXn3eaI59YBgbg0oJH2c4UygRTI1gd0wuopVotWtGg/fiEK21JBDeoVrNJ/ar8g==} + '@ast-grep/napi-linux-arm64-musl@0.27.3': + resolution: {integrity: sha512-py9CbDjn2Tpk1Rri4jHEcxFV1K00Q41cVUFH44C2uGL2wolAHOHWc45cb+U+ncarf2IpSdRXe+QEfzHZovyE6w==} engines: {node: '>= 10'} cpu: [arm64] os: [linux] @@ -1991,8 +1991,8 @@ packages: os: [linux] libc: [glibc] - '@ast-grep/napi-linux-x64-gnu@0.27.2': - resolution: {integrity: sha512-K7U68gMIJHLxAXAiex6CBRKsieAXLsvMgIo/RLSh46Bzr8O5z2zZbc2DamT28cke81FltbBOhYNICi68hYFegg==} + '@ast-grep/napi-linux-x64-gnu@0.27.3': + resolution: {integrity: sha512-eDYPOGiKvBNgRXl2W1RcvLND2O5gLYw4/8eMqHEzA4+EnVPlWes6Nw1VPIUMOc9kRLj0QD1ksj4hZdQ/Krx1Kg==} engines: {node: '>= 10'} cpu: [x64] os: [linux] @@ -2005,8 +2005,8 @@ packages: os: [linux] libc: [musl] - '@ast-grep/napi-linux-x64-musl@0.27.2': - resolution: {integrity: sha512-j437X4TaadXhUfCyYgKg1F5DD97ms02EaMJbiokUHHnCOZulqK7JD92Os9ufVwkGx2QjkEhCqBmHg+70hoczjQ==} + '@ast-grep/napi-linux-x64-musl@0.27.3': + resolution: {integrity: sha512-0If6+AtaxOFR7o6Lr8Borwi7K7IXg5ey0E/x0GroBr668BnHoiaCg6J68vv4Ad8rl0Gt2cW/A3rI/FXAUwareA==} engines: {node: '>= 10'} cpu: [x64] os: [linux] @@ -2018,8 +2018,8 @@ packages: cpu: [arm64] os: [win32] - '@ast-grep/napi-win32-arm64-msvc@0.27.2': - resolution: {integrity: sha512-aK0vVIJ/k2mtQzcm/nJhAg5m/wfQlK26wJ1oqwJxvIb3ks+CpMV9BpxQ4Fkai9rzCJN1Na+6AVTFEDsircEQbg==} + '@ast-grep/napi-win32-arm64-msvc@0.27.3': + resolution: {integrity: sha512-7L5GKTXWD9STntNbG1EkWqQ5WCtCb9XCg3bWuf7+nNiQe0QmUd6naPakDln21cIgL21XyOfdbpvoKb6883MZlg==} engines: {node: '>= 10'} cpu: [arm64] os: [win32] @@ -2030,8 +2030,8 @@ packages: cpu: [ia32] os: [win32] - '@ast-grep/napi-win32-ia32-msvc@0.27.2': - resolution: {integrity: sha512-M/Tk1srYu4r/LYezeeazeRprGzUFHUoMd+pxOi6y+MBVUXhp/p95J4LHG7uYdprxuSSqGzNnr+S6AGCtiWQljg==} + '@ast-grep/napi-win32-ia32-msvc@0.27.3': + resolution: {integrity: sha512-53e8oE4Qa/QIf/QWOgDqRJ4BW4UbzK5mYIMU0hRoIRAaZUstXTj86CYGFfArb1+EGsu9NmizhRqtg+wnVns+Ng==} engines: {node: '>= 10'} cpu: [ia32] os: [win32] @@ -2042,8 +2042,8 @@ packages: cpu: [x64] os: [win32] - '@ast-grep/napi-win32-x64-msvc@0.27.2': - resolution: {integrity: sha512-E/W38ZdacIilvoobQENmOB9t8AH4p41QSk1XR0za7m3mz2O2TZQCPUXVW6f4dNemv66GWqPmun+Cynhtt+yVuw==} + '@ast-grep/napi-win32-x64-msvc@0.27.3': + resolution: {integrity: sha512-1Bw+ZMlWUEIshIzq7LZ8MiScv550zFDNX3duTf7A/DKR4p1dMzKHcgNdau9+lClNOh5PHmzhZXSjse8rLTArHA==} engines: {node: '>= 10'} cpu: [x64] os: [win32] @@ -2052,24 +2052,20 @@ packages: resolution: {integrity: sha512-kNF87HiI4omHC7VzyBZSvqOAXtMlSDRF2YX+O5ya0XKv/7/GYms1opLQ+BQ9twLLDj0WsSFX4MYg0TrinZTxTg==} engines: {node: '>= 10'} - '@ast-grep/napi@0.27.2': - resolution: {integrity: sha512-DDFPthgmNTgK4t4z2gfYeSIwxqM4KigdWnNmGSKk9S1w0L2x/Dv788MRFMUNGzFot3nEqmjrf9yQ1Sw5x5AZsw==} + '@ast-grep/napi@0.27.3': + resolution: {integrity: sha512-tdjbiW2shIa1TwVyrzqdF5JuwwbqUSUG49yifXLeZ9IUPvkqgxLb2/gdKbu2yzcbTMshrP46YpBE16ldLM/GGQ==} engines: {node: '>= 10'} '@babel/code-frame@7.25.7': resolution: {integrity: sha512-0xZJFNE5XMpENsgfHYTw8FbX4kv53mFLn2i3XPoq69LyhYSCBJtitaHx9QnsVTrsogI4Z3+HtEfZ2/GFPOtf5g==} engines: {node: '>=6.9.0'} - '@babel/compat-data@7.25.7': - resolution: {integrity: sha512-9ickoLz+hcXCeh7jrcin+/SLWm+GkxE2kTvoYyp38p4WkdFXfQJxDFGWp/YHjiKLPx06z2A7W8XKuqbReXDzsw==} - engines: {node: '>=6.9.0'} - '@babel/compat-data@7.25.8': resolution: {integrity: sha512-ZsysZyXY4Tlx+Q53XdnOFmqwfB9QDTHYxaZYajWRoBLuLEAwI2UIbtxOjWh/cFaa9IKUlcB+DDuoskLuKu56JA==} engines: {node: '>=6.9.0'} - '@babel/core@7.25.7': - resolution: {integrity: sha512-yJ474Zv3cwiSOO9nXJuqzvwEeM+chDuQ8GJirw+pZ91sCGCyOZ3dJkVE09fTV0VEVzXyLWhh3G/AolYTPX7Mow==} + '@babel/core@7.25.8': + resolution: {integrity: sha512-Oixnb+DzmRT30qu9d3tJSQkxuygWm32DFykT4bRoORPa9hZ/L4KhVB/XiRm6KG+roIEM7DBQlmg27kw2HZkdZg==} engines: {node: '>=6.9.0'} '@babel/generator@7.25.7': @@ -2171,11 +2167,6 @@ packages: resolution: {integrity: sha512-iYyACpW3iW8Fw+ZybQK+drQre+ns/tKpXbNESfrhNnPLIklLbXr7MYJ6gPEd0iETGLOK+SxMjVvKb/ffmk+FEw==} engines: {node: '>=6.9.0'} - '@babel/parser@7.25.7': - resolution: {integrity: sha512-aZn7ETtQsjjGG5HruveUK06cU3Hljuhd9Iojm4M8WWv3wLE6OkE5PWbDUkItmMgegmccaITudyuW5RPYrYlgWw==} - engines: {node: '>=6.0.0'} - hasBin: true - '@babel/parser@7.25.8': resolution: {integrity: sha512-HcttkxzdPucv3nNFmfOOMfFf64KgdJVqm1KaCm25dPGMLElo9nsLvXeJECQg8UzPuBGLyTSA0ZzqCtDSzKTEoQ==} engines: {node: '>=6.0.0'} @@ -2585,8 +2576,8 @@ packages: resolution: {integrity: sha512-FjoyLe754PMiYsFaN5C94ttGiOmBNYTf6pLr4xXHAT5uctHb092PBszndLDR5XA/jghQvn4n7JMHl7dmTgbm9w==} engines: {node: '>=6.9.0'} - '@babel/standalone@7.25.7': - resolution: {integrity: sha512-7H+mK18Ew4C/pIIiZwF1eiVjUEh2Ju/BpwRZwcPeXltF/rIjHjFL0gol7PtGrHocmIq6P6ubJrylmmWQ3lGJPA==} + '@babel/standalone@7.25.8': + resolution: {integrity: sha512-UvRanvLCGPRscJ5Rw9o6vUBS5P+E+gkhl6eaokrIN+WM1kUkmj254VZhyihFdDZVDlI3cPcZoakbJJw24QPISw==} engines: {node: '>=6.9.0'} '@babel/template@7.25.7': @@ -2597,10 +2588,6 @@ packages: resolution: {integrity: sha512-jatJPT1Zjqvh/1FyJs6qAHL+Dzb7sTb+xr7Q+gM1b+1oBsMsQQ4FkVKb6dFlJvLlVssqkRzV05Jzervt9yhnzg==} engines: {node: '>=6.9.0'} - '@babel/types@7.25.7': - resolution: {integrity: sha512-vwIVdXG+j+FOpkwqHRcBgHLYNL7XMkufrlaFvL9o6Ai9sJn9+PdyIL5qa0XzTZw084c+u9LOls53eoZWP/W5WQ==} - engines: {node: '>=6.9.0'} - '@babel/types@7.25.8': resolution: {integrity: sha512-JWtuCu8VQsMladxVz/P4HzHUGCAwpuqacmowgXFs5XjxIgKuNjnLokQzuVjlTvIzODaDmpjT3oxcC48vyk9EWg==} engines: {node: '>=6.9.0'} @@ -2771,164 +2758,164 @@ packages: resolution: {integrity: sha512-6QY0147RmiXIpTMkwI7a6dx+/CtPserPxxsK2p0DFf4bTHOGExx3dlPg7eGL8UFO0/C/nrXPAMy5kZEstPclXA==} engines: {node: '>=18'} - '@cspell/dict-ada@4.0.2': - resolution: {integrity: sha512-0kENOWQeHjUlfyId/aCM/mKXtkEgV0Zu2RhUXCBr4hHo9F9vph+Uu8Ww2b0i5a4ZixoIkudGA+eJvyxrG1jUpA==} + '@cspell/dict-ada@4.0.5': + resolution: {integrity: sha512-6/RtZ/a+lhFVmrx/B7bfP7rzC4yjEYe8o74EybXcvu4Oue6J4Ey2WSYj96iuodloj1LWrkNCQyX5h4Pmcj0Iag==} - '@cspell/dict-aws@4.0.4': - resolution: {integrity: sha512-6AWI/Kkf+RcX/J81VX8+GKLeTgHWEr/OMhGk3dHQzWK66RaqDJCGDqi7494ghZKcBB7dGa3U5jcKw2FZHL/u3w==} + '@cspell/dict-aws@4.0.7': + resolution: {integrity: sha512-PoaPpa2NXtSkhGIMIKhsJUXB6UbtTt6Ao3x9JdU9kn7fRZkwD4RjHDGqulucIOz7KeEX/dNRafap6oK9xHe4RA==} - '@cspell/dict-bash@4.1.5': - resolution: {integrity: sha512-YGim/h7E2U5HCCb2ckNufT6/yyWygt9nSZ5C7qw6oOD3bygbObqD1+rlPor1JW+YyO+3GwTIHE70uKEEU6VZYw==} + '@cspell/dict-bash@4.1.8': + resolution: {integrity: sha512-I2CM2pTNthQwW069lKcrVxchJGMVQBzru2ygsHCwgidXRnJL/NTjAPOFTxN58Jc1bf7THWghfEDyKX/oyfc0yg==} - '@cspell/dict-companies@3.1.4': - resolution: {integrity: sha512-y9e0amzEK36EiiKx3VAA+SHQJPpf2Qv5cCt5eTUSggpTkiFkCh6gRKQ97rVlrKh5GJrqinDwYIJtTsxuh2vy2Q==} + '@cspell/dict-companies@3.1.7': + resolution: {integrity: sha512-ncVs/efuAkP1/tLDhWbXukBjgZ5xOUfe03neHMWsE8zvXXc5+Lw6TX5jaJXZLOoES/f4j4AhRE20jsPCF5pm+A==} - '@cspell/dict-cpp@5.1.19': - resolution: {integrity: sha512-i/odUPNFLdqWisOktu6c4qjUR4k+P9Al2RCri3Wso9EFblp53xt/5jIUdGMdDDVQGqX7s/KLtdqNxNKqP3/d+w==} + '@cspell/dict-cpp@5.1.22': + resolution: {integrity: sha512-g1/8P5/Q+xnIc8Js4UtBg3XOhcFrFlFbG3UWVtyEx49YTf0r9eyDtDt1qMMDBZT91pyCwLcAEbwS+4i5PIfNZw==} - '@cspell/dict-cryptocurrencies@5.0.0': - resolution: {integrity: sha512-Z4ARIw5+bvmShL+4ZrhDzGhnc9znaAGHOEMaB/GURdS/jdoreEDY34wdN0NtdLHDO5KO7GduZnZyqGdRoiSmYA==} + '@cspell/dict-cryptocurrencies@5.0.3': + resolution: {integrity: sha512-bl5q+Mk+T3xOZ12+FG37dB30GDxStza49Rmoax95n37MTLksk9wBo1ICOlPJ6PnDUSyeuv4SIVKgRKMKkJJglA==} - '@cspell/dict-csharp@4.0.2': - resolution: {integrity: sha512-1JMofhLK+4p4KairF75D3A924m5ERMgd1GvzhwK2geuYgd2ZKuGW72gvXpIV7aGf52E3Uu1kDXxxGAiZ5uVG7g==} + '@cspell/dict-csharp@4.0.5': + resolution: {integrity: sha512-c/sFnNgtRwRJxtC3JHKkyOm+U3/sUrltFeNwml9VsxKBHVmvlg4tk4ar58PdpW9/zTlGUkWi2i85//DN1EsUCA==} - '@cspell/dict-css@4.0.13': - resolution: {integrity: sha512-WfOQkqlAJTo8eIQeztaH0N0P+iF5hsJVKFuhy4jmARPISy8Efcv8QXk2/IVbmjJH0/ZV7dKRdnY5JFVXuVz37g==} + '@cspell/dict-css@4.0.16': + resolution: {integrity: sha512-70qu7L9z/JR6QLyJPk38fNTKitlIHnfunx0wjpWQUQ8/jGADIhMCrz6hInBjqPNdtGpYm8d1dNFyF8taEkOgrQ==} - '@cspell/dict-dart@2.2.1': - resolution: {integrity: sha512-yriKm7QkoPx3JPSSOcw6iX9gOb2N50bOo/wqWviqPYbhpMRh9Xiv6dkUy3+ot+21GuShZazO8X6U5+Vw67XEwg==} + '@cspell/dict-dart@2.2.4': + resolution: {integrity: sha512-of/cVuUIZZK/+iqefGln8G3bVpfyN6ZtH+LyLkHMoR5tEj+2vtilGNk9ngwyR8L4lEqbKuzSkOxgfVjsXf5PsQ==} - '@cspell/dict-data-science@2.0.2': - resolution: {integrity: sha512-VwAck6OZQVqrscKyOrvllixIugIPF+Q6YoFNvXZCPhHGtNyOAVraD3S7kOgPYBdUjgno4QbdMWm92BUPqL1QjQ==} + '@cspell/dict-data-science@2.0.5': + resolution: {integrity: sha512-nNSILXmhSJox9/QoXICPQgm8q5PbiSQP4afpbkBqPi/u/b3K9MbNH5HvOOa6230gxcGdbZ9Argl2hY/U8siBlg==} - '@cspell/dict-django@4.1.0': - resolution: {integrity: sha512-bKJ4gPyrf+1c78Z0Oc4trEB9MuhcB+Yg+uTTWsvhY6O2ncFYbB/LbEZfqhfmmuK/XJJixXfI1laF2zicyf+l0w==} + '@cspell/dict-django@4.1.3': + resolution: {integrity: sha512-yBspeL3roJlO0a1vKKNaWABURuHdHZ9b1L8d3AukX0AsBy9snSggc8xCavPmSzNfeMDXbH+1lgQiYBd3IW03fg==} - '@cspell/dict-docker@1.1.7': - resolution: {integrity: sha512-XlXHAr822euV36GGsl2J1CkBIVg3fZ6879ZOg5dxTIssuhUOCiV2BuzKZmt6aIFmcdPmR14+9i9Xq+3zuxeX0A==} + '@cspell/dict-docker@1.1.10': + resolution: {integrity: sha512-vWybMfsG/8jhN6kmPoilMon36GB3+Ef+m/mgYUfY8tJN23K/x4KD1rU1OOiNWzDqePhu3MMWVKO5W5x6VI6Gbw==} - '@cspell/dict-dotnet@5.0.5': - resolution: {integrity: sha512-gjg0L97ee146wX47dnA698cHm85e7EOpf9mVrJD8DmEaqoo/k1oPy2g7c7LgKxK9XnqwoXxhLNnngPrwXOoEtQ==} + '@cspell/dict-dotnet@5.0.8': + resolution: {integrity: sha512-MD8CmMgMEdJAIPl2Py3iqrx3B708MbCIXAuOeZ0Mzzb8YmLmiisY7QEYSZPg08D7xuwARycP0Ki+bb0GAkFSqg==} - '@cspell/dict-elixir@4.0.3': - resolution: {integrity: sha512-g+uKLWvOp9IEZvrIvBPTr/oaO6619uH/wyqypqvwpmnmpjcfi8+/hqZH8YNKt15oviK8k4CkINIqNhyndG9d9Q==} + '@cspell/dict-elixir@4.0.6': + resolution: {integrity: sha512-TfqSTxMHZ2jhiqnXlVKM0bUADtCvwKQv2XZL/DI0rx3doG8mEMS8SGPOmiyyGkHpR/pGOq18AFH3BEm4lViHIw==} - '@cspell/dict-en-common-misspellings@2.0.4': - resolution: {integrity: sha512-lvOiRjV/FG4pAGZL3PN2GCVHSTCE92cwhfLGGkOsQtxSmef6WCHfHwp9auafkBlX0yFQSKDfq6/TlpQbjbJBtQ==} + '@cspell/dict-en-common-misspellings@2.0.7': + resolution: {integrity: sha512-qNFo3G4wyabcwnM+hDrMYKN9vNVg/k9QkhqSlSst6pULjdvPyPs1mqz1689xO/v9t8e6sR4IKc3CgUXDMTYOpA==} '@cspell/dict-en-gb@1.1.33': resolution: {integrity: sha512-tKSSUf9BJEV+GJQAYGw5e+ouhEe2ZXE620S7BLKe3ZmpnjlNG9JqlnaBhkIMxKnNFkLY2BP/EARzw31AZnOv4g==} - '@cspell/dict-en_us@4.3.23': - resolution: {integrity: sha512-l0SoEQBsi3zDSl3OuL4/apBkxjuj4hLIg/oy6+gZ7LWh03rKdF6VNtSZNXWAmMY+pmb1cGA3ouleTiJIglbsIg==} + '@cspell/dict-en_us@4.3.26': + resolution: {integrity: sha512-hDbHYJsi3UgU1J++B0WLiYhWQdsmve3CH53FIaMRAdhrWOHcuw7h1dYkQXHFEP5lOjaq53KUHp/oh5su6VkIZg==} - '@cspell/dict-filetypes@3.0.4': - resolution: {integrity: sha512-IBi8eIVdykoGgIv5wQhOURi5lmCNJq0we6DvqKoPQJHthXbgsuO1qrHSiUVydMiQl/XvcnUWTMeAlVUlUClnVg==} + '@cspell/dict-filetypes@3.0.7': + resolution: {integrity: sha512-/DN0Ujp9/EXvpTcgih9JmBaE8n+G0wtsspyNdvHT5luRfpfol1xm/CIQb6xloCXCiLkWX+EMPeLSiVIZq+24dA==} '@cspell/dict-flutter@1.0.3': resolution: {integrity: sha512-52C9aUEU22ptpgYh6gQyIdA4MP6NPwzbEqndfgPh3Sra191/kgs7CVqXiO1qbtZa9gnYHUoVApkoxRE7mrXHfg==} - '@cspell/dict-fonts@4.0.0': - resolution: {integrity: sha512-t9V4GeN/m517UZn63kZPUYP3OQg5f0OBLSd3Md5CU3eH1IFogSvTzHHnz4Wqqbv8NNRiBZ3HfdY/pqREZ6br3Q==} + '@cspell/dict-fonts@4.0.3': + resolution: {integrity: sha512-sPd17kV5qgYXLteuHFPn5mbp/oCHKgitNfsZLFC3W2fWEgZlhg4hK+UGig3KzrYhhvQ8wBnmZrAQm0TFKCKzsA==} '@cspell/dict-fsharp@1.0.4': resolution: {integrity: sha512-G5wk0o1qyHUNi9nVgdE1h5wl5ylq7pcBjX8vhjHcO4XBq20D5eMoXjwqMo/+szKAqzJ+WV3BgAL50akLKrT9Rw==} - '@cspell/dict-fullstack@3.2.0': - resolution: {integrity: sha512-sIGQwU6G3rLTo+nx0GKyirR5dQSFeTIzFTOrURw51ISf+jKG9a3OmvsVtc2OANfvEAOLOC9Wfd8WYhmsO8KRDQ==} + '@cspell/dict-fullstack@3.2.3': + resolution: {integrity: sha512-62PbndIyQPH11mAv0PyiyT0vbwD0AXEocPpHlCHzfb5v9SspzCCbzQ/LIBiFmyRa+q5LMW35CnSVu6OXdT+LKg==} - '@cspell/dict-gaming-terms@1.0.5': - resolution: {integrity: sha512-C3riccZDD3d9caJQQs1+MPfrUrQ+0KHdlj9iUR1QD92FgTOF6UxoBpvHUUZ9YSezslcmpFQK4xQQ5FUGS7uWfw==} + '@cspell/dict-gaming-terms@1.0.8': + resolution: {integrity: sha512-7OL0zTl93WFWhhtpXFrtm9uZXItC3ncAs8d0iQDMMFVNU1rBr6raBNxJskxE5wx2Ant12fgI66ZGVagXfN+yfA==} '@cspell/dict-git@3.0.3': resolution: {integrity: sha512-LSxB+psZ0qoj83GkyjeEH/ZViyVsGEF/A6BAo8Nqc0w0HjD2qX/QR4sfA6JHUgQ3Yi/ccxdK7xNIo67L2ScW5A==} - '@cspell/dict-golang@6.0.13': - resolution: {integrity: sha512-uBUWi+AjFpluB6qF0rsC1gGyooqXeKPUdWHSmSXW/DCnS5PBSjRW6VWWp8efc1Fanob0QJxiZiYlc4U7oxuG6Q==} + '@cspell/dict-golang@6.0.16': + resolution: {integrity: sha512-hZOBlgcguv2Hdc93n2zjdAQm1j3grsN9T9WhPnQ1wh2vUDoCLEujg+6gWhjcLb8ECOcwZTWgNyQLWeOxEsAj/w==} '@cspell/dict-google@1.0.4': resolution: {integrity: sha512-JThUT9eiguCja1mHHLwYESgxkhk17Gv7P3b1S7ZJzXw86QyVHPrbpVoMpozHk0C9o+Ym764B7gZGKmw9uMGduQ==} - '@cspell/dict-haskell@4.0.1': - resolution: {integrity: sha512-uRrl65mGrOmwT7NxspB4xKXFUenNC7IikmpRZW8Uzqbqcu7ZRCUfstuVH7T1rmjRgRkjcIjE4PC11luDou4wEQ==} + '@cspell/dict-haskell@4.0.4': + resolution: {integrity: sha512-EwQsedEEnND/vY6tqRfg9y7tsnZdxNqOxLXSXTsFA6JRhUlr8Qs88iUUAfsUzWc4nNmmzQH2UbtT25ooG9x4nA==} - '@cspell/dict-html-symbol-entities@4.0.0': - resolution: {integrity: sha512-HGRu+48ErJjoweR5IbcixxETRewrBb0uxQBd6xFGcxbEYCX8CnQFTAmKI5xNaIt2PKaZiJH3ijodGSqbKdsxhw==} + '@cspell/dict-html-symbol-entities@4.0.3': + resolution: {integrity: sha512-aABXX7dMLNFdSE8aY844X4+hvfK7977sOWgZXo4MTGAmOzR8524fjbJPswIBK7GaD3+SgFZ2yP2o0CFvXDGF+A==} - '@cspell/dict-html@4.0.6': - resolution: {integrity: sha512-cLWHfuOhE4wqwC12up6Doxo2u1xxVhX1A8zriR4CUD+osFQzUIcBK1ykNXppga+rt1WyypaJdTU2eV6OpzYrgQ==} + '@cspell/dict-html@4.0.9': + resolution: {integrity: sha512-BNp7w3m910K4qIVyOBOZxHuFNbVojUY6ES8Y8r7YjYgJkm2lCuQoVwwhPjurnomJ7BPmZTb+3LLJ58XIkgF7JQ==} - '@cspell/dict-java@5.0.7': - resolution: {integrity: sha512-ejQ9iJXYIq7R09BScU2y5OUGrSqwcD+J5mHFOKbduuQ5s/Eh/duz45KOzykeMLI6KHPVxhBKpUPBWIsfewECpQ==} + '@cspell/dict-java@5.0.10': + resolution: {integrity: sha512-pVNcOnmoGiNL8GSVq4WbX/Vs2FGS0Nej+1aEeGuUY9CU14X8yAVCG+oih5ZoLt1jaR8YfR8byUF8wdp4qG4XIw==} '@cspell/dict-julia@1.0.4': resolution: {integrity: sha512-bFVgNX35MD3kZRbXbJVzdnN7OuEqmQXGpdOi9jzB40TSgBTlJWA4nxeAKV4CPCZxNRUGnLH0p05T/AD7Aom9/w==} - '@cspell/dict-k8s@1.0.6': - resolution: {integrity: sha512-srhVDtwrd799uxMpsPOQqeDJY+gEocgZpoK06EFrb4GRYGhv7lXo9Fb+xQMyQytzOW9dw4DNOEck++nacDuymg==} + '@cspell/dict-k8s@1.0.9': + resolution: {integrity: sha512-Q7GELSQIzo+BERl2ya/nBEnZeQC+zJP19SN1pI6gqDYraM51uYJacbbcWLYYO2Y+5joDjNt/sd/lJtLaQwoSlA==} - '@cspell/dict-latex@4.0.0': - resolution: {integrity: sha512-LPY4y6D5oI7D3d+5JMJHK/wxYTQa2lJMSNxps2JtuF8hbAnBQb3igoWEjEbIbRRH1XBM0X8dQqemnjQNCiAtxQ==} + '@cspell/dict-latex@4.0.3': + resolution: {integrity: sha512-2KXBt9fSpymYHxHfvhUpjUFyzrmN4c4P8mwIzweLyvqntBT3k0YGZJSriOdjfUjwSygrfEwiuPI1EMrvgrOMJw==} - '@cspell/dict-lorem-ipsum@4.0.0': - resolution: {integrity: sha512-1l3yjfNvMzZPibW8A7mQU4kTozwVZVw0AvFEdy+NcqtbxH+TvbSkNMqROOFWrkD2PjnKG0+Ea0tHI2Pi6Gchnw==} + '@cspell/dict-lorem-ipsum@4.0.3': + resolution: {integrity: sha512-WFpDi/PDYHXft6p0eCXuYnn7mzMEQLVeqpO+wHSUd+kz5ADusZ4cpslAA4wUZJstF1/1kMCQCZM6HLZic9bT8A==} - '@cspell/dict-lua@4.0.3': - resolution: {integrity: sha512-lDHKjsrrbqPaea13+G9s0rtXjMO06gPXPYRjRYawbNmo4E/e3XFfVzeci3OQDQNDmf2cPOwt9Ef5lu2lDmwfJg==} + '@cspell/dict-lua@4.0.6': + resolution: {integrity: sha512-Jwvh1jmAd9b+SP9e1GkS2ACbqKKRo9E1f9GdjF/ijmooZuHU0hPyqvnhZzUAxO1egbnNjxS/J2T6iUtjAUK2KQ==} - '@cspell/dict-makefile@1.0.0': - resolution: {integrity: sha512-3W9tHPcSbJa6s0bcqWo6VisEDTSN5zOtDbnPabF7rbyjRpNo0uHXHRJQF8gAbFzoTzBBhgkTmrfSiuyQm7vBUQ==} + '@cspell/dict-makefile@1.0.3': + resolution: {integrity: sha512-R3U0DSpvTs6qdqfyBATnePj9Q/pypkje0Nj26mQJ8TOBQutCRAJbr2ZFAeDjgRx5EAJU/+8txiyVF97fbVRViw==} - '@cspell/dict-monkeyc@1.0.6': - resolution: {integrity: sha512-oO8ZDu/FtZ55aq9Mb67HtaCnsLn59xvhO/t2mLLTHAp667hJFxpp7bCtr2zOrR1NELzFXmKln/2lw/PvxMSvrA==} + '@cspell/dict-monkeyc@1.0.9': + resolution: {integrity: sha512-Jvf6g5xlB4+za3ThvenYKREXTEgzx5gMUSzrAxIiPleVG4hmRb/GBSoSjtkGaibN3XxGx5x809gSTYCA/IHCpA==} - '@cspell/dict-node@5.0.1': - resolution: {integrity: sha512-lax/jGz9h3Dv83v8LHa5G0bf6wm8YVRMzbjJPG/9rp7cAGPtdrga+XANFq+B7bY5+jiSA3zvj10LUFCFjnnCCg==} + '@cspell/dict-node@5.0.4': + resolution: {integrity: sha512-Hz5hiuOvZTd7Cp1IBqUZ7/ChwJeQpD5BJuwCaDn4mPNq4iMcQ1iWBYMThvNVqCEDgKv63X52nT8RAWacss98qg==} - '@cspell/dict-npm@5.1.5': - resolution: {integrity: sha512-oAOGWuJYU3DlO+cAsStKMWN8YEkBue25cRC9EwdiL5Z84nchU20UIoYrLfIQejMlZca+1GyrNeyxRAgn4KiivA==} + '@cspell/dict-npm@5.1.8': + resolution: {integrity: sha512-AJELYXeB4fQdIoNfmuaQxB1Hli3cX6XPsQCjfBxlu0QYXhrjB/IrCLLQAjWIywDqJiWyGUFTz4DqaANm8C/r9Q==} - '@cspell/dict-php@4.0.10': - resolution: {integrity: sha512-NfTZdp6kcZDF1PvgQ6cY0zE4FUO5rSwNmBH/iwCBuaLfJAFQ97rgjxo+D2bic4CFwNjyHutnHPtjJBRANO5XQw==} + '@cspell/dict-php@4.0.13': + resolution: {integrity: sha512-P6sREMZkhElzz/HhXAjahnICYIqB/HSGp1EhZh+Y6IhvC15AzgtDP8B8VYCIsQof6rPF1SQrFwunxOv8H1e2eg==} - '@cspell/dict-powershell@5.0.10': - resolution: {integrity: sha512-U4H0zm94sNK+YP7jSFb7xb160XLf2dKIPVt5sOYctKlEyR9M16sP8FHbyWV2Yp1YtxXugoNdeCm2vwGEDAd8sg==} + '@cspell/dict-powershell@5.0.13': + resolution: {integrity: sha512-0qdj0XZIPmb77nRTynKidRJKTU0Fl+10jyLbAhFTuBWKMypVY06EaYFnwhsgsws/7nNX8MTEQuewbl9bWFAbsg==} - '@cspell/dict-public-licenses@2.0.8': - resolution: {integrity: sha512-Sup+tFS7cDV0fgpoKtUqEZ6+fA/H+XUgBiqQ/Fbs6vUE3WCjJHOIVsP+udHuyMH7iBfJ4UFYOYeORcY4EaKdMg==} + '@cspell/dict-public-licenses@2.0.11': + resolution: {integrity: sha512-rR5KjRUSnVKdfs5G+gJ4oIvQvm8+NJ6cHWY2N+GE69/FSGWDOPHxulCzeGnQU/c6WWZMSimG9o49i9r//lUQyA==} - '@cspell/dict-python@4.2.8': - resolution: {integrity: sha512-4y5dynLiajvowhB3PqlcwJ2C4okK1y2Hombec1+TGcV9sUBfo8FYNw6VRFUUrpsxO+Ut/3ncIifdZS5/zAWi5w==} + '@cspell/dict-python@4.2.11': + resolution: {integrity: sha512-bshNZqP5FYRO0CtZ9GgtVjHidrSuRRF537MU/sPew8oaqWPg066F9KQfPllbRi9AzFqqeS2l7/ACYUrFMe21gw==} - '@cspell/dict-r@2.0.1': - resolution: {integrity: sha512-KCmKaeYMLm2Ip79mlYPc8p+B2uzwBp4KMkzeLd5E6jUlCL93Y5Nvq68wV5fRLDRTf7N1LvofkVFWfDcednFOgA==} + '@cspell/dict-r@2.0.4': + resolution: {integrity: sha512-cBpRsE/U0d9BRhiNRMLMH1PpWgw+N+1A2jumgt1if9nBGmQw4MUpg2u9I0xlFVhstTIdzXiLXMxP45cABuiUeQ==} - '@cspell/dict-ruby@5.0.4': - resolution: {integrity: sha512-URw0jScj5pv8sKCVLNnde11qVCQR442rUpSd12u46Swl+5qBaSdnOUoCWQk419kd9/dpC6bB/3l4kOSY2fdYHw==} + '@cspell/dict-ruby@5.0.7': + resolution: {integrity: sha512-4/d0hcoPzi5Alk0FmcyqlzFW9lQnZh9j07MJzPcyVO62nYJJAGKaPZL2o4qHeCS/od/ctJC5AHRdoUm0ktsw6Q==} - '@cspell/dict-rust@4.0.6': - resolution: {integrity: sha512-Buzy9PfLbdRPibSth8CV1D8ZsYqybo26yNIlAN+8ehU0pSBss0Jv4aleL4vKQ3FjouXeAC27rtEsLd7yaMZTog==} + '@cspell/dict-rust@4.0.9': + resolution: {integrity: sha512-Dhr6TIZsMV92xcikKIWei6p/qswS4M+gTkivpWwz4/1oaVk2nRrxJmCdRoVkJlZkkAc17rjxrS12mpnJZI0iWw==} - '@cspell/dict-scala@5.0.3': - resolution: {integrity: sha512-4yGb4AInT99rqprxVNT9TYb1YSpq58Owzq7zi3ZS5T0u899Y4VsxsBiOgHnQ/4W+ygi+sp+oqef8w8nABR2lkg==} + '@cspell/dict-scala@5.0.6': + resolution: {integrity: sha512-tl0YWAfjUVb4LyyE4JIMVE8DlLzb1ecHRmIWc4eT6nkyDqQgHKzdHsnusxFEFMVLIQomgSg0Zz6hJ5S1E4W4ww==} - '@cspell/dict-software-terms@4.1.7': - resolution: {integrity: sha512-+fFTALseXszDN8/khonF1DpTcYzwyNqYxhATLakr7CUPtUCO1fCH4lidMtBN4UtPVpE6tbjc5D8tj51PJxEOcw==} + '@cspell/dict-software-terms@4.1.10': + resolution: {integrity: sha512-+9PuQ9MHQhlET6Hv1mGcWDh6Rb+StzjBMrjfksDeBHBIVdT66u9uCkaZapIzfgktflY4m9oK7+dEynr+BAxvtQ==} - '@cspell/dict-sql@2.1.5': - resolution: {integrity: sha512-FmxanytHXss7GAWAXmgaxl3icTCW7YxlimyOSPNfm+njqeUDjw3kEv4mFNDDObBJv8Ec5AWCbUDkWIpkE3IpKg==} + '@cspell/dict-sql@2.1.8': + resolution: {integrity: sha512-dJRE4JV1qmXTbbGm6WIcg1knmR6K5RXnQxF4XHs5HA3LAjc/zf77F95i5LC+guOGppVF6Hdl66S2UyxT+SAF3A==} - '@cspell/dict-svelte@1.0.2': - resolution: {integrity: sha512-rPJmnn/GsDs0btNvrRBciOhngKV98yZ9SHmg8qI6HLS8hZKvcXc0LMsf9LLuMK1TmS2+WQFAan6qeqg6bBxL2Q==} + '@cspell/dict-svelte@1.0.5': + resolution: {integrity: sha512-sseHlcXOqWE4Ner9sg8KsjxwSJ2yssoJNqFHR9liWVbDV+m7kBiUtn2EB690TihzVsEmDr/0Yxrbb5Bniz70mA==} - '@cspell/dict-swift@2.0.1': - resolution: {integrity: sha512-gxrCMUOndOk7xZFmXNtkCEeroZRnS2VbeaIPiymGRHj5H+qfTAzAKxtv7jJbVA3YYvEzWcVE2oKDP4wcbhIERw==} + '@cspell/dict-swift@2.0.4': + resolution: {integrity: sha512-CsFF0IFAbRtYNg0yZcdaYbADF5F3DsM8C4wHnZefQy8YcHP/qjAF/GdGfBFBLx+XSthYuBlo2b2XQVdz3cJZBw==} - '@cspell/dict-terraform@1.0.2': - resolution: {integrity: sha512-UZdJwWIpib2Rx02w6vtXTU3z+M/VMZU0F1dhSL3Ab9otQsFntT8U1CX7wBSqQCLg8bJiCfnUyVvMK3UBm3SR8A==} + '@cspell/dict-terraform@1.0.5': + resolution: {integrity: sha512-qH3epPB2d6d5w1l4hR2OsnN8qDQ4P0z6oDB7+YiNH+BoECXv4Z38MIV1H8cxIzD2wkzkt2JTcFYaVW72MDZAlg==} - '@cspell/dict-typescript@3.1.6': - resolution: {integrity: sha512-1beC6O4P/j23VuxX+i0+F7XqPVc3hhiAzGJHEKqnWf5cWAXQtg0xz3xQJ5MvYx2a7iLaSa+lu7+05vG9UHyu9Q==} + '@cspell/dict-typescript@3.1.9': + resolution: {integrity: sha512-ZtO1/cVWvvR477ftTl2TFR09+IIzXG1rcin8CGYA0FO5WhyDAbn8v3A85QikS158BhTVUoq09lPYuSF9HBzqvw==} '@cspell/dict-vue@3.0.3': resolution: {integrity: sha512-akmYbrgAGumqk1xXALtDJcEcOMYBYMnkjpmGzH13Ozhq1mkPF4VgllFQlm1xYde+BUKNnzMgPEzxrL2qZllgYA==} @@ -2984,22 +2971,12 @@ packages: '@csstools/css-parser-algorithms': ^3.0.2 '@csstools/css-tokenizer': ^3.0.2 - '@csstools/css-parser-algorithms@3.0.1': - resolution: {integrity: sha512-lSquqZCHxDfuTg/Sk2hiS0mcSFCEBuj49JfzPHJogDBT0mGCyY5A1AQzBWngitrp7i1/HAZpIgzF/VjhOEIJIg==} - engines: {node: '>=18'} - peerDependencies: - '@csstools/css-tokenizer': ^3.0.1 - '@csstools/css-parser-algorithms@3.0.2': resolution: {integrity: sha512-6tC/MnlEvs5suR4Ahef4YlBccJDHZuxGsAlxXmybWjZ5jPxlzLSMlRZ9mVHSRvlD+CmtE7+hJ+UQbfXrws/rUQ==} engines: {node: '>=18'} peerDependencies: '@csstools/css-tokenizer': ^3.0.2 - '@csstools/css-tokenizer@3.0.1': - resolution: {integrity: sha512-UBqaiu7kU0lfvaP982/o3khfXccVlHPWp0/vwwiIgDF0GmqqqxoiXC/6FCjlS9u92f7CoEz6nXKQnrn1kIAkOw==} - engines: {node: '>=18'} - '@csstools/css-tokenizer@3.0.2': resolution: {integrity: sha512-IuTRcD53WHsXPCZ6W7ubfGqReTJ9Ra0yRRFmXYP/Re8hFYYfoIYIK4080X5luslVLWimhIeFq0hj09urVMQzTw==} engines: {node: '>=18'} @@ -3282,8 +3259,8 @@ packages: cpu: [ppc64] os: [aix] - '@esbuild/aix-ppc64@0.23.1': - resolution: {integrity: sha512-6VhYk1diRqrhBAqpJEdjASR/+WVRtfjpqKuNw11cLiaWpAT/Uu+nokB+UJnevzy/P9C/ty6AOe0dwueMrGh/iQ==} + '@esbuild/aix-ppc64@0.24.0': + resolution: {integrity: sha512-WtKdFM7ls47zkKHFVzMz8opM7LkcsIp9amDUBIAWirg70RM71WRSjdILPsY5Uv1D42ZpUfaPILDlfactHgsRkw==} engines: {node: '>=18'} cpu: [ppc64] os: [aix] @@ -3306,8 +3283,8 @@ packages: cpu: [arm64] os: [android] - '@esbuild/android-arm64@0.23.1': - resolution: {integrity: sha512-xw50ipykXcLstLeWH7WRdQuysJqejuAGPd30vd1i5zSyKK3WE+ijzHmLKxdiCMtH1pHz78rOg0BKSYOSB/2Khw==} + '@esbuild/android-arm64@0.24.0': + resolution: {integrity: sha512-Vsm497xFM7tTIPYK9bNTYJyF/lsP590Qc1WxJdlB6ljCbdZKU9SY8i7+Iin4kyhV/KV5J2rOKsBQbB77Ab7L/w==} engines: {node: '>=18'} cpu: [arm64] os: [android] @@ -3330,8 +3307,8 @@ packages: cpu: [arm] os: [android] - '@esbuild/android-arm@0.23.1': - resolution: {integrity: sha512-uz6/tEy2IFm9RYOyvKl88zdzZfwEfKZmnX9Cj1BHjeSGNuGLuMD1kR8y5bteYmwqKm1tj8m4cb/aKEorr6fHWQ==} + '@esbuild/android-arm@0.24.0': + resolution: {integrity: sha512-arAtTPo76fJ/ICkXWetLCc9EwEHKaeya4vMrReVlEIUCAUncH7M4bhMQ+M9Vf+FFOZJdTNMXNBrWwW+OXWpSew==} engines: {node: '>=18'} cpu: [arm] os: [android] @@ -3354,8 +3331,8 @@ packages: cpu: [x64] os: [android] - '@esbuild/android-x64@0.23.1': - resolution: {integrity: sha512-nlN9B69St9BwUoB+jkyU090bru8L0NA3yFvAd7k8dNsVH8bi9a8cUAUSEcEEgTp2z3dbEDGJGfP6VUnkQnlReg==} + '@esbuild/android-x64@0.24.0': + resolution: {integrity: sha512-t8GrvnFkiIY7pa7mMgJd7p8p8qqYIz1NYiAoKc75Zyv73L3DZW++oYMSHPRarcotTKuSs6m3hTOa5CKHaS02TQ==} engines: {node: '>=18'} cpu: [x64] os: [android] @@ -3378,8 +3355,8 @@ packages: cpu: [arm64] os: [darwin] - '@esbuild/darwin-arm64@0.23.1': - resolution: {integrity: sha512-YsS2e3Wtgnw7Wq53XXBLcV6JhRsEq8hkfg91ESVadIrzr9wO6jJDMZnCQbHm1Guc5t/CdDiFSSfWP58FNuvT3Q==} + '@esbuild/darwin-arm64@0.24.0': + resolution: {integrity: sha512-CKyDpRbK1hXwv79soeTJNHb5EiG6ct3efd/FTPdzOWdbZZfGhpbcqIpiD0+vwmpu0wTIL97ZRPZu8vUt46nBSw==} engines: {node: '>=18'} cpu: [arm64] os: [darwin] @@ -3402,8 +3379,8 @@ packages: cpu: [x64] os: [darwin] - '@esbuild/darwin-x64@0.23.1': - resolution: {integrity: sha512-aClqdgTDVPSEGgoCS8QDG37Gu8yc9lTHNAQlsztQ6ENetKEO//b8y31MMu2ZaPbn4kVsIABzVLXYLhCGekGDqw==} + '@esbuild/darwin-x64@0.24.0': + resolution: {integrity: sha512-rgtz6flkVkh58od4PwTRqxbKH9cOjaXCMZgWD905JOzjFKW+7EiUObfd/Kav+A6Gyud6WZk9w+xu6QLytdi2OA==} engines: {node: '>=18'} cpu: [x64] os: [darwin] @@ -3426,8 +3403,8 @@ packages: cpu: [arm64] os: [freebsd] - '@esbuild/freebsd-arm64@0.23.1': - resolution: {integrity: sha512-h1k6yS8/pN/NHlMl5+v4XPfikhJulk4G+tKGFIOwURBSFzE8bixw1ebjluLOjfwtLqY0kewfjLSrO6tN2MgIhA==} + '@esbuild/freebsd-arm64@0.24.0': + resolution: {integrity: sha512-6Mtdq5nHggwfDNLAHkPlyLBpE5L6hwsuXZX8XNmHno9JuL2+bg2BX5tRkwjyfn6sKbxZTq68suOjgWqCicvPXA==} engines: {node: '>=18'} cpu: [arm64] os: [freebsd] @@ -3450,8 +3427,8 @@ packages: cpu: [x64] os: [freebsd] - '@esbuild/freebsd-x64@0.23.1': - resolution: {integrity: sha512-lK1eJeyk1ZX8UklqFd/3A60UuZ/6UVfGT2LuGo3Wp4/z7eRTRYY+0xOu2kpClP+vMTi9wKOfXi2vjUpO1Ro76g==} + '@esbuild/freebsd-x64@0.24.0': + resolution: {integrity: sha512-D3H+xh3/zphoX8ck4S2RxKR6gHlHDXXzOf6f/9dbFt/NRBDIE33+cVa49Kil4WUjxMGW0ZIYBYtaGCa2+OsQwQ==} engines: {node: '>=18'} cpu: [x64] os: [freebsd] @@ -3474,8 +3451,8 @@ packages: cpu: [arm64] os: [linux] - '@esbuild/linux-arm64@0.23.1': - resolution: {integrity: sha512-/93bf2yxencYDnItMYV/v116zff6UyTjo4EtEQjUBeGiVpMmffDNUyD9UN2zV+V3LRV3/on4xdZ26NKzn6754g==} + '@esbuild/linux-arm64@0.24.0': + resolution: {integrity: sha512-TDijPXTOeE3eaMkRYpcy3LarIg13dS9wWHRdwYRnzlwlA370rNdZqbcp0WTyyV/k2zSxfko52+C7jU5F9Tfj1g==} engines: {node: '>=18'} cpu: [arm64] os: [linux] @@ -3498,8 +3475,8 @@ packages: cpu: [arm] os: [linux] - '@esbuild/linux-arm@0.23.1': - resolution: {integrity: sha512-CXXkzgn+dXAPs3WBwE+Kvnrf4WECwBdfjfeYHpMeVxWE0EceB6vhWGShs6wi0IYEqMSIzdOF1XjQ/Mkm5d7ZdQ==} + '@esbuild/linux-arm@0.24.0': + resolution: {integrity: sha512-gJKIi2IjRo5G6Glxb8d3DzYXlxdEj2NlkixPsqePSZMhLudqPhtZ4BUrpIuTjJYXxvF9njql+vRjB2oaC9XpBw==} engines: {node: '>=18'} cpu: [arm] os: [linux] @@ -3522,8 +3499,8 @@ packages: cpu: [ia32] os: [linux] - '@esbuild/linux-ia32@0.23.1': - resolution: {integrity: sha512-VTN4EuOHwXEkXzX5nTvVY4s7E/Krz7COC8xkftbbKRYAl96vPiUssGkeMELQMOnLOJ8k3BY1+ZY52tttZnHcXQ==} + '@esbuild/linux-ia32@0.24.0': + resolution: {integrity: sha512-K40ip1LAcA0byL05TbCQ4yJ4swvnbzHscRmUilrmP9Am7//0UjPreh4lpYzvThT2Quw66MhjG//20mrufm40mA==} engines: {node: '>=18'} cpu: [ia32] os: [linux] @@ -3546,8 +3523,8 @@ packages: cpu: [loong64] os: [linux] - '@esbuild/linux-loong64@0.23.1': - resolution: {integrity: sha512-Vx09LzEoBa5zDnieH8LSMRToj7ir/Jeq0Gu6qJ/1GcBq9GkfoEAoXvLiW1U9J1qE/Y/Oyaq33w5p2ZWrNNHNEw==} + '@esbuild/linux-loong64@0.24.0': + resolution: {integrity: sha512-0mswrYP/9ai+CU0BzBfPMZ8RVm3RGAN/lmOMgW4aFUSOQBjA31UP8Mr6DDhWSuMwj7jaWOT0p0WoZ6jeHhrD7g==} engines: {node: '>=18'} cpu: [loong64] os: [linux] @@ -3570,8 +3547,8 @@ packages: cpu: [mips64el] os: [linux] - '@esbuild/linux-mips64el@0.23.1': - resolution: {integrity: sha512-nrFzzMQ7W4WRLNUOU5dlWAqa6yVeI0P78WKGUo7lg2HShq/yx+UYkeNSE0SSfSure0SqgnsxPvmAUu/vu0E+3Q==} + '@esbuild/linux-mips64el@0.24.0': + resolution: {integrity: sha512-hIKvXm0/3w/5+RDtCJeXqMZGkI2s4oMUGj3/jM0QzhgIASWrGO5/RlzAzm5nNh/awHE0A19h/CvHQe6FaBNrRA==} engines: {node: '>=18'} cpu: [mips64el] os: [linux] @@ -3594,8 +3571,8 @@ packages: cpu: [ppc64] os: [linux] - '@esbuild/linux-ppc64@0.23.1': - resolution: {integrity: sha512-dKN8fgVqd0vUIjxuJI6P/9SSSe/mB9rvA98CSH2sJnlZ/OCZWO1DJvxj8jvKTfYUdGfcq2dDxoKaC6bHuTlgcw==} + '@esbuild/linux-ppc64@0.24.0': + resolution: {integrity: sha512-HcZh5BNq0aC52UoocJxaKORfFODWXZxtBaaZNuN3PUX3MoDsChsZqopzi5UupRhPHSEHotoiptqikjN/B77mYQ==} engines: {node: '>=18'} cpu: [ppc64] os: [linux] @@ -3618,8 +3595,8 @@ packages: cpu: [riscv64] os: [linux] - '@esbuild/linux-riscv64@0.23.1': - resolution: {integrity: sha512-5AV4Pzp80fhHL83JM6LoA6pTQVWgB1HovMBsLQ9OZWLDqVY8MVobBXNSmAJi//Csh6tcY7e7Lny2Hg1tElMjIA==} + '@esbuild/linux-riscv64@0.24.0': + resolution: {integrity: sha512-bEh7dMn/h3QxeR2KTy1DUszQjUrIHPZKyO6aN1X4BCnhfYhuQqedHaa5MxSQA/06j3GpiIlFGSsy1c7Gf9padw==} engines: {node: '>=18'} cpu: [riscv64] os: [linux] @@ -3642,8 +3619,8 @@ packages: cpu: [s390x] os: [linux] - '@esbuild/linux-s390x@0.23.1': - resolution: {integrity: sha512-9ygs73tuFCe6f6m/Tb+9LtYxWR4c9yg7zjt2cYkjDbDpV/xVn+68cQxMXCjUpYwEkze2RcU/rMnfIXNRFmSoDw==} + '@esbuild/linux-s390x@0.24.0': + resolution: {integrity: sha512-ZcQ6+qRkw1UcZGPyrCiHHkmBaj9SiCD8Oqd556HldP+QlpUIe2Wgn3ehQGVoPOvZvtHm8HPx+bH20c9pvbkX3g==} engines: {node: '>=18'} cpu: [s390x] os: [linux] @@ -3666,8 +3643,8 @@ packages: cpu: [x64] os: [linux] - '@esbuild/linux-x64@0.23.1': - resolution: {integrity: sha512-EV6+ovTsEXCPAp58g2dD68LxoP/wK5pRvgy0J/HxPGB009omFPv3Yet0HiaqvrIrgPTBuC6wCH1LTOY91EO5hQ==} + '@esbuild/linux-x64@0.24.0': + resolution: {integrity: sha512-vbutsFqQ+foy3wSSbmjBXXIJ6PL3scghJoM8zCL142cGaZKAdCZHyf+Bpu/MmX9zT9Q0zFBVKb36Ma5Fzfa8xA==} engines: {node: '>=18'} cpu: [x64] os: [linux] @@ -3690,14 +3667,14 @@ packages: cpu: [x64] os: [netbsd] - '@esbuild/netbsd-x64@0.23.1': - resolution: {integrity: sha512-aevEkCNu7KlPRpYLjwmdcuNz6bDFiE7Z8XC4CPqExjTvrHugh28QzUXVOZtiYghciKUacNktqxdpymplil1beA==} + '@esbuild/netbsd-x64@0.24.0': + resolution: {integrity: sha512-hjQ0R/ulkO8fCYFsG0FZoH+pWgTTDreqpqY7UnQntnaKv95uP5iW3+dChxnx7C3trQQU40S+OgWhUVwCjVFLvg==} engines: {node: '>=18'} cpu: [x64] os: [netbsd] - '@esbuild/openbsd-arm64@0.23.1': - resolution: {integrity: sha512-3x37szhLexNA4bXhLrCC/LImN/YtWis6WXr1VESlfVtVeoFJBRINPJ3f0a/6LV8zpikqoUg4hyXw0sFBt5Cr+Q==} + '@esbuild/openbsd-arm64@0.24.0': + resolution: {integrity: sha512-MD9uzzkPQbYehwcN583yx3Tu5M8EIoTD+tUgKF982WYL9Pf5rKy9ltgD0eUgs8pvKnmizxjXZyLt0z6DC3rRXg==} engines: {node: '>=18'} cpu: [arm64] os: [openbsd] @@ -3720,8 +3697,8 @@ packages: cpu: [x64] os: [openbsd] - '@esbuild/openbsd-x64@0.23.1': - resolution: {integrity: sha512-aY2gMmKmPhxfU+0EdnN+XNtGbjfQgwZj43k8G3fyrDM/UdZww6xrWxmDkuz2eCZchqVeABjV5BpildOrUbBTqA==} + '@esbuild/openbsd-x64@0.24.0': + resolution: {integrity: sha512-4ir0aY1NGUhIC1hdoCzr1+5b43mw99uNwVzhIq1OY3QcEwPDO3B7WNXBzaKY5Nsf1+N11i1eOfFcq+D/gOS15Q==} engines: {node: '>=18'} cpu: [x64] os: [openbsd] @@ -3744,8 +3721,8 @@ packages: cpu: [x64] os: [sunos] - '@esbuild/sunos-x64@0.23.1': - resolution: {integrity: sha512-RBRT2gqEl0IKQABT4XTj78tpk9v7ehp+mazn2HbUeZl1YMdaGAQqhapjGTCe7uw7y0frDi4gS0uHzhvpFuI1sA==} + '@esbuild/sunos-x64@0.24.0': + resolution: {integrity: sha512-jVzdzsbM5xrotH+W5f1s+JtUy1UWgjU0Cf4wMvffTB8m6wP5/kx0KiaLHlbJO+dMgtxKV8RQ/JvtlFcdZ1zCPA==} engines: {node: '>=18'} cpu: [x64] os: [sunos] @@ -3768,8 +3745,8 @@ packages: cpu: [arm64] os: [win32] - '@esbuild/win32-arm64@0.23.1': - resolution: {integrity: sha512-4O+gPR5rEBe2FpKOVyiJ7wNDPA8nGzDuJ6gN4okSA1gEOYZ67N8JPk58tkWtdtPeLz7lBnY6I5L3jdsr3S+A6A==} + '@esbuild/win32-arm64@0.24.0': + resolution: {integrity: sha512-iKc8GAslzRpBytO2/aN3d2yb2z8XTVfNV0PjGlCxKo5SgWmNXx82I/Q3aG1tFfS+A2igVCY97TJ8tnYwpUWLCA==} engines: {node: '>=18'} cpu: [arm64] os: [win32] @@ -3792,8 +3769,8 @@ packages: cpu: [ia32] os: [win32] - '@esbuild/win32-ia32@0.23.1': - resolution: {integrity: sha512-BcaL0Vn6QwCwre3Y717nVHZbAa4UBEigzFm6VdsVdT/MbZ38xoj1X9HPkZhbmaBGUD1W8vxAfffbDe8bA6AKnQ==} + '@esbuild/win32-ia32@0.24.0': + resolution: {integrity: sha512-vQW36KZolfIudCcTnaTpmLQ24Ha1RjygBo39/aLkM2kmjkWmZGEJ5Gn9l5/7tzXA42QGIoWbICfg6KLLkIw6yw==} engines: {node: '>=18'} cpu: [ia32] os: [win32] @@ -3816,8 +3793,8 @@ packages: cpu: [x64] os: [win32] - '@esbuild/win32-x64@0.23.1': - resolution: {integrity: sha512-BHpFFeslkWrXWyUPnbKm+xYYVYruCinGcftSBaa8zoF9hZO4BcSCFUvHVTtzpIY6YzUnYtuEhZ+C9iEXjxnasg==} + '@esbuild/win32-x64@0.24.0': + resolution: {integrity: sha512-7IAFPrjSQIJrGsK6flwg7NFmwBoSTyF3rl7If0hNUFQU4ilTsEPL6GuMuU9BfIWVVGuRnuIidkSMC+c0Otu8IA==} engines: {node: '>=18'} cpu: [x64] os: [win32] @@ -3895,8 +3872,8 @@ packages: resolution: {integrity: sha512-JBxkERygn7Bv/GbN5Rv8Ul6LVknS+5Bp6RgDC/O8gEBU/yeH5Ui5C/OlWrTb6qct7LjjfT6Re2NxB0ln0yYybA==} engines: {node: '>=18.18'} - '@iconify-json/logos@1.2.2': - resolution: {integrity: sha512-Z78rxWqmAu9pBczootCg4/TjVTAqcoNdqzZ3KRD8Nof3BD1AMqyDXS7+BNBcsGMBgSkfUJYHk0iInBi6wUEvUw==} + '@iconify-json/logos@1.2.3': + resolution: {integrity: sha512-JLHS5hgZP1b55EONAWNeqBUuriRfRNKWXK4cqYx0PpVaJfIIMiiMxFfvoQiX/bkE9XgkLhcKmDUqL3LXPdXPwQ==} '@iconify-json/octicon@1.2.1': resolution: {integrity: sha512-4w7yMipQtp6s6aCHrSVlVXsf0OCBQ8CRmUTkMQUBexR42SXl4z5GnaUyaOMVoZttfgaBNnj0mlMB5T1cmRDgTg==} @@ -4624,9 +4601,6 @@ packages: '@types/node@12.20.55': resolution: {integrity: sha512-J8xLz7q2OFulZ2cyGTLE1TbbZcjpno7FaN6zdJNrgAdrJ+DZzh/uFR6YrTb4C+nXakvud8Q4+rbhoIWlYQbUFQ==} - '@types/node@22.7.4': - resolution: {integrity: sha512-y+NPi1rFzDs1NdQHHToqeiX2TIS79SWEAw9GYhkkx8bD0ChpfqC+n2j5OXOCpzfojBEBt6DnEnnG9MY0zk1XLg==} - '@types/node@22.7.5': resolution: {integrity: sha512-jML7s2NAzMWc//QSJ1a3prpk78cOPchGvXJsC3C6R6PSMoooztvRVQEz89gmBTBY1SPMaqo5teB4uNHPdetShQ==} @@ -4691,10 +4665,6 @@ packages: resolution: {integrity: sha512-jjhdIE/FPF2B7Z1uzc6i3oWKbGcHb87Qw7AWj6jmEqNOfDFbJWtjt/XfwCpvNkpGWlcJaog5vTR+VV8+w9JflA==} engines: {node: ^18.18.0 || >=20.0.0} - '@typescript-eslint/scope-manager@8.8.0': - resolution: {integrity: sha512-EL8eaGC6gx3jDd8GwEFEV091210U97J0jeEHrAYvIYosmEGet4wJ+g0SYmLu+oRiAwbSA5AVrt6DxLHfdd+bUg==} - engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} - '@typescript-eslint/scope-manager@8.8.1': resolution: {integrity: sha512-X4JdU+66Mazev/J0gfXlcC/dV6JI37h+93W9BRYXrSn0hrE64IoWgVkO9MSJgEzoWkxONgaQpICWg8vAN74wlA==} engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} @@ -4712,10 +4682,6 @@ packages: resolution: {integrity: sha512-iZqi+Ds1y4EDYUtlOOC+aUmxnE9xS/yCigkjA7XpTKV6nCBd3Hp/PRGGmdwnfkV2ThMyYldP1wRpm/id99spTQ==} engines: {node: ^18.18.0 || >=20.0.0} - '@typescript-eslint/types@8.8.0': - resolution: {integrity: sha512-QJwc50hRCgBd/k12sTykOJbESe1RrzmX6COk8Y525C9l7oweZ+1lw9JiU56im7Amm8swlz00DRIlxMYLizr2Vw==} - engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} - '@typescript-eslint/types@8.8.1': resolution: {integrity: sha512-WCcTP4SDXzMd23N27u66zTKMuEevH4uzU8C9jf0RO4E04yVHgQgW+r+TeVTNnO1KIfrL8ebgVVYYMMO3+jC55Q==} engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} @@ -4729,15 +4695,6 @@ packages: typescript: optional: true - '@typescript-eslint/typescript-estree@8.8.0': - resolution: {integrity: sha512-ZaMJwc/0ckLz5DaAZ+pNLmHv8AMVGtfWxZe/x2JVEkD5LnmhWiQMMcYT7IY7gkdJuzJ9P14fRy28lUrlDSWYdw==} - engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} - peerDependencies: - typescript: '*' - peerDependenciesMeta: - typescript: - optional: true - '@typescript-eslint/typescript-estree@8.8.1': resolution: {integrity: sha512-A5d1R9p+X+1js4JogdNilDuuq+EHZdsH9MjTVxXOdVFfTJXunKJR/v+fNNyO4TnoOn5HqobzfRlc70NC6HTcdg==} engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} @@ -4753,12 +4710,6 @@ packages: peerDependencies: eslint: ^8.56.0 - '@typescript-eslint/utils@8.8.0': - resolution: {integrity: sha512-QE2MgfOTem00qrlPgyByaCHay9yb1+9BjnMFnSFkUKQfu7adBXDTnCAivURnuPPAG/qiB+kzKkZKmKfaMT0zVg==} - engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} - peerDependencies: - eslint: ^8.57.0 || ^9.0.0 - '@typescript-eslint/utils@8.8.1': resolution: {integrity: sha512-/QkNJDbV0bdL7H7d0/y0qBbV2HTtf0TIyjSDTvvmQEzeVx8jEImEbLuOA4EsvE8gIgqMitns0ifb5uQhMj8d9w==} engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} @@ -4769,10 +4720,6 @@ packages: resolution: {integrity: sha512-cDF0/Gf81QpY3xYyJKDV14Zwdmid5+uuENhjH2EqFaF0ni+yAyq/LzMaIJdhNJXZI7uLzwIlA+V7oWoyn6Curg==} engines: {node: ^18.18.0 || >=20.0.0} - '@typescript-eslint/visitor-keys@8.8.0': - resolution: {integrity: sha512-8mq51Lx6Hpmd7HnA2fcHQo3YgfX1qbccxQOgZcb4tvasu//zXRaA1j5ZRFeCw/VRAdFi4mRM9DnZw0Nu0Q2d1g==} - engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} - '@typescript-eslint/visitor-keys@8.8.1': resolution: {integrity: sha512-0/TdC3aeRAsW7MDvYRwEc1Uwm0TIBfzjPFgg60UU2Haj5qsCs9cc3zNgY71edqE3LbWfF/WoZQd3lJoDXFQpag==} engines: {node: ^18.18.0 || ^20.9.0 || >=21.1.0} @@ -4841,14 +4788,14 @@ packages: '@vitest/utils@2.1.2': resolution: {integrity: sha512-zMO2KdYy6mx56btx9JvAqAZ6EyS3g49krMPPrgOp1yxGZiA93HumGk+bZ5jIZtOg5/VBYl5eBmGRQHqq4FG6uQ==} - '@volar/language-core@2.4.5': - resolution: {integrity: sha512-F4tA0DCO5Q1F5mScHmca0umsi2ufKULAnMOVBfMsZdT4myhVl4WdKRwCaKcfOkIEuyrAVvtq1ESBdZ+rSyLVww==} + '@volar/language-core@2.4.6': + resolution: {integrity: sha512-FxUfxaB8sCqvY46YjyAAV6c3mMIq/NWQMVvJ+uS4yxr1KzOvyg61gAuOnNvgCvO4TZ7HcLExBEsWcDu4+K4E8A==} - '@volar/source-map@2.4.5': - resolution: {integrity: sha512-varwD7RaKE2J/Z+Zu6j3mNNJbNT394qIxXwdvz/4ao/vxOfyClZpSDtLKkwWmecinkOVos5+PWkWraelfMLfpw==} + '@volar/source-map@2.4.6': + resolution: {integrity: sha512-Nsh7UW2ruK+uURIPzjJgF0YRGP5CX9nQHypA2OMqdM2FKy7rh+uv3XgPnWPw30JADbKvZ5HuBzG4gSbVDYVtiw==} - '@volar/typescript@2.4.5': - resolution: {integrity: sha512-mcT1mHvLljAEtHviVcBuOyAwwMKz1ibXTi5uYtP/pf4XxoAzpdkQ+Br2IC0NPCvLCbjPZmbf3I0udndkfB1CDg==} + '@volar/typescript@2.4.6': + resolution: {integrity: sha512-NMIrA7y5OOqddL9VtngPWYmdQU03htNKFtAYidbYfWA0TOhyGVd9tfcP4TsLWQ+RBWDZCbBqsr8xzU0ZOxYTCQ==} '@vue/babel-helper-vue-transform-on@1.2.5': resolution: {integrity: sha512-lOz4t39ZdmU4DJAa2hwPYmKc8EsuGa2U0L9KaZaOJUt0UwQNjNA3AZTq6uEivhOKhhG1Wvy96SvYBoFmCg3uuw==} @@ -4866,27 +4813,15 @@ packages: peerDependencies: '@babel/core': ^7.0.0-0 - '@vue/compiler-core@3.5.11': - resolution: {integrity: sha512-PwAdxs7/9Hc3ieBO12tXzmTD+Ln4qhT/56S+8DvrrZ4kLDn4Z/AMUr8tXJD0axiJBS0RKIoNaR0yMuQB9v9Udg==} - '@vue/compiler-core@3.5.12': resolution: {integrity: sha512-ISyBTRMmMYagUxhcpyEH0hpXRd/KqDU4ymofPgl2XAkY9ZhQ+h0ovEZJIiPop13UmR/54oA2cgMDjgroRelaEw==} - '@vue/compiler-dom@3.5.11': - resolution: {integrity: sha512-pyGf8zdbDDRkBrEzf8p7BQlMKNNF5Fk/Cf/fQ6PiUz9at4OaUfyXW0dGJTo2Vl1f5U9jSLCNf0EZJEogLXoeew==} - '@vue/compiler-dom@3.5.12': resolution: {integrity: sha512-9G6PbJ03uwxLHKQ3P42cMTi85lDRvGLB2rSGOiQqtXELat6uI4n8cNz9yjfVHRPIu+MsK6TE418Giruvgptckg==} - '@vue/compiler-sfc@3.5.11': - resolution: {integrity: sha512-gsbBtT4N9ANXXepprle+X9YLg2htQk1sqH/qGJ/EApl+dgpUBdTv3yP7YlR535uHZY3n6XaR0/bKo0BgwwDniw==} - '@vue/compiler-sfc@3.5.12': resolution: {integrity: sha512-2k973OGo2JuAa5+ZlekuQJtitI5CgLMOwgl94BzMCsKZCX/xiqzJYzapl4opFogKHqwJk34vfsaKpfEhd1k5nw==} - '@vue/compiler-ssr@3.5.11': - resolution: {integrity: sha512-P4+GPjOuC2aFTk1Z4WANvEhyOykcvEd5bIj2KVNGKGfM745LaXGr++5njpdBTzVz5pZifdlR1kpYSJJpIlSePA==} - '@vue/compiler-ssr@3.5.12': resolution: {integrity: sha512-eLwc7v6bfGBSM7wZOGPmRavSWzNFF6+PdRhE+VFJhNCgHiF8AM7ccoqcv5kBXA2eWUfigD7byekvf/JsOfKvPA==} @@ -4932,9 +4867,6 @@ packages: peerDependencies: vue: ^3.5.12 - '@vue/shared@3.5.11': - resolution: {integrity: sha512-W8GgysJVnFo81FthhzurdRAWP/byq3q2qIw70e0JWblzVhjgOMiC2GyovXrZTFQJnFVryYaKGP3Tc9vYzYm6PQ==} - '@vue/shared@3.5.12': resolution: {integrity: sha512-L2RPSAwUFbgZH20etwrXyVyCBu9OxRSi8T/38QsvnkJyvq2LufW2lDCOzm7t/U9C1mkhJGWYfCuFBCmIuNivrg==} @@ -5281,8 +5213,8 @@ packages: bintrees@1.0.2: resolution: {integrity: sha512-VOMgTMwjAaUG580SXn3LacVgjurrbMme7ZZNYGSSV7mmtY6QQRh0Eg3pwIcntQ77DErK1L0NxkbetjcoXzVwKw==} - birpc@0.2.17: - resolution: {integrity: sha512-+hkTxhot+dWsLpp3gia5AkVHIsKlZybNT5gIYiDlNzJrmYPcTM9k5/w2uaj3IPpd7LlEYpmCj4Jj1nC41VhDFg==} + birpc@0.2.19: + resolution: {integrity: sha512-5WeXXAvTmitV1RqJFppT5QtUiz2p1mRSYU000Jkft5ZUCLJIk4uQriYNO50HknxKwM6jd8utNc66K1qGIwwWBQ==} boolbase@1.0.0: resolution: {integrity: sha512-JZOSA7Mo9sNGB8+UjSgzdLtokWAky1zbztM3WRLCbZ70/3cTANmQmOdR7y2g+J0e2WXywy1yS468tY+IruqEww==} @@ -5376,8 +5308,8 @@ packages: caniuse-api@3.0.0: resolution: {integrity: sha512-bsTwuIg/BZZK/vreVTYYbSWoe2F+71P7K5QGEX+pT250DZbfU1MQ5prOKpPR+LL6uWKK3KMwMCAS74QB3Um1uw==} - caniuse-lite@1.0.30001667: - resolution: {integrity: sha512-7LTwJjcRkzKFmtqGsibMeuXmvFDfZq/nzIjnmgCGzKKRVzjD72selLDK1oPF/Oxzmt4fNcPvTDvGqSDG4tCALw==} + caniuse-lite@1.0.30001668: + resolution: {integrity: sha512-nWLrdxqCdblixUO+27JtGJJE/txpJlyUy5YN1u53wLZkP0emYCo5zgS6QYft7VUYR42LGgi/S5hdLZTrnyIddw==} ccount@2.0.1: resolution: {integrity: sha512-eyrF0jiFpY+3drT6383f1qhkbGsLSifNAjA61IUjZjmLCWjItY6LB9ft9YhoDgwfmclB2zhu51Lc7+95b8NRAg==} @@ -5602,9 +5534,6 @@ packages: concat-map@0.0.1: resolution: {integrity: sha512-/Srv4dswyQNBfohGpz9o6Yb3Gz3SrUDqBH5rTuhGR7ahtlbYKnVxw2bCFMRljaA7EXHaXZ8wsHdodFvbkhKmqg==} - confbox@0.1.7: - resolution: {integrity: sha512-uJcB/FKZtBMCJpK8MQji6bJHgu1tixKPxRLeGkNzBoOZzpnZUJm0jm2/sBDWcuBx1dYgxV4JU+g5hmNxCyAmdA==} - confbox@0.1.8: resolution: {integrity: sha512-RMtmw0iFkeR4YV+fUOSucriAQNb9g8zFR52MWCtl+cCZOFRNL6zeB395vPzFhEjjn4fMxXudmELnl/KF/WrK6w==} @@ -6145,8 +6074,8 @@ packages: engines: {node: '>=0.10.0'} hasBin: true - electron-to-chromium@1.5.32: - resolution: {integrity: sha512-M+7ph0VGBQqqpTT2YrabjNKSQ2fEl9PVx6AK3N558gDH9NO8O6XN9SXXFWRo9u9PbEg/bWq+tjXQr+eXmxubCw==} + electron-to-chromium@1.5.36: + resolution: {integrity: sha512-HYTX8tKge/VNp6FGO+f/uVDmUkq+cEfcxYhKf15Akc4M5yxt5YmorwlAitKWjWhWQnKcDRBAQKXkhqqXMqcrjw==} element-plus@2.8.5: resolution: {integrity: sha512-Px+kPbRTVvn5oa5+9saa7QEOnUweKXm0JVI7yJHzKF/doQGixwcFMsQEF2+3Fy62EA/7dRRKVuhsNGGZYNk3cw==} @@ -6261,8 +6190,8 @@ packages: engines: {node: '>=12'} hasBin: true - esbuild@0.23.1: - resolution: {integrity: sha512-VVNz/9Sa0bs5SELtn3f7qhJCDPCF5oMEl5cO9/SSinpE9hbPVvxbd572HH5AKiP7WD8INO53GgfDDhRjkylHEg==} + esbuild@0.24.0: + resolution: {integrity: sha512-FuLPevChGDshgSicjisSooU0cemp/sGXR841D5LHMB7mTVOmsEHcAxaH3irL53+8YDIeVNQEySh4DaYU/iuPqQ==} engines: {node: '>=18'} hasBin: true @@ -6653,8 +6582,8 @@ packages: resolution: {integrity: sha512-Ld2g8rrAyMYFXBhEqMz8ZAHBi4J4uS1i/CxGMDnjyFWddMXLVcDp051DZfu+t7+ab7Wv6SMqpWmyFIj5UbfFvg==} engines: {node: '>=14'} - form-data@4.0.0: - resolution: {integrity: sha512-ETEklSGi5t0QMZuiXoA/Q6vcnxcLQP5vdugSpuAyi6SVGi2clPPp+xgEhuMaHC+zGgn31Kd235W35f7Hykkaww==} + form-data@4.0.1: + resolution: {integrity: sha512-tzN8e4TX8+kkxGPK8D5u0FNmjPUjw3lwC9lSLxxoB/+GtsJG91CO8bSWy73APlgAZzZbXEYZJuxjkHH2w+Ezhw==} engines: {node: '>= 6'} fraction.js@4.3.7: @@ -6732,9 +6661,6 @@ packages: resolution: {integrity: sha512-2nk+7SIVb14QrgXFHcm84tD4bKQz0RxPuMT8Ag5KPOq7J5fEmAg0UbXdTOSHqNuHSU28k55qnceesxXRZGzKWA==} engines: {node: '>=18'} - get-func-name@2.0.2: - resolution: {integrity: sha512-8vXOvuE167CtIc3OyItco7N/dpRtBbYOsPsXCz7X/PMnlGjYjSGuZJgM1Y7mmew7BKf9BqvLX2tnOVy1BBUsxQ==} - get-intrinsic@1.2.4: resolution: {integrity: sha512-5uYhsJH8VJBTv7oslg4BznJYhDoRI6waYCxMmCdnTrcCrHA/fCFKoTFz2JKKE0HdDFUF7/oQuhzumXJK7paBRQ==} engines: {node: '>= 0.4'} @@ -7352,8 +7278,8 @@ packages: resolution: {integrity: sha512-2yTgeWTWzMWkHu6Jp9NKgePDaYHbntiwvYuuJLbbN9vl7DC9DvXKOB2BC3ZZ92D3cvV/aflH0osDfwpHepQ53w==} hasBin: true - jiti@2.2.1: - resolution: {integrity: sha512-weIl/Bv3G0J3UKamLxEA2G+FfQ33Z1ZkQJGPjKFV21zQdKWu2Pi6o4elpj2uEl5XdFJZ9xzn1fsanWTFSt45zw==} + jiti@2.3.3: + resolution: {integrity: sha512-EX4oNDwcXSivPrw2qKH2LB5PoFxEvgtv2JgwW0bU858HoLQ+kutSvjLMUqBd0PeJYEinLWhoI9Ol0eYMqj/wNQ==} hasBin: true jju@1.4.0: @@ -7628,8 +7554,8 @@ packages: resolution: {integrity: sha512-lyuxPGr/Wfhrlem2CL/UcnUc1zcqKAImBDzukY7Y5F/yQiNdko6+fRLevlw1HgMySw7f611UIY408EtxRSoK3Q==} hasBin: true - loupe@3.1.1: - resolution: {integrity: sha512-edNu/8D5MKVfGVFRhFf8aAxiTM6Wumfz5XsaatSxlD3w4R1d/WEKUTydCdPGbl9K7QG/Ca3GnDV2sIKIpXRQcw==} + loupe@3.1.2: + resolution: {integrity: sha512-23I4pFZHmAemUnz8WZXbYRSKYj801VDaNv9ETuMh7IrMc7VuVVSo+Z9iLE3ni30+U48iDWfi30d3twAXBYmnCg==} lower-case@2.0.2: resolution: {integrity: sha512-7fm3l3NAF9WfN6W3JOmf5drwpVqX78JtoGJ3A6W0a6ZnldM41w2fV5D490psKFTpMds8TJse/eHLFFsNHHjHgg==} @@ -7659,9 +7585,6 @@ packages: magic-string@0.25.9: resolution: {integrity: sha512-RmF0AsMzgt25qzqqLc1+MbHmhdx0ojF2Fvs4XnOqz2ZOBXzzkEwc/dJQZCYHAn7v1jbVOjAZfK8msRn4BxO4VQ==} - magic-string@0.30.11: - resolution: {integrity: sha512-+Wri9p0QHMy+545hKww7YAu5NyzF8iomPL/RQazugQ9+Ez4Ic3mERMd8ZTX5rfK944j+560ZJi8iAwgak1Ac7A==} - magic-string@0.30.12: resolution: {integrity: sha512-Ea8I3sQMVXr8JhN4z+H/d8zwo+tYDgHE9+5G4Wnrwhs0gaK9fXTKx0Tw5Xwsd/bCPTTZNRAdpyzvoeORe9LYpw==} @@ -7846,8 +7769,8 @@ packages: engines: {node: '>=10'} hasBin: true - mkdist@1.5.9: - resolution: {integrity: sha512-PdJimzhcgDxaHpk1SUabw56gT3BU15vBHUTHkeeus8Kl7jUkpgG7+z0PiS/y23XXgO8TiU/dKP3L1oG55qrP1g==} + mkdist@1.6.0: + resolution: {integrity: sha512-nD7J/mx33Lwm4Q4qoPgRBVA9JQNKgyE7fLo5vdPWVDdjz96pXglGERp/fRnGPCTB37Kykfxs5bDdXa9BWOT9nw==} hasBin: true peerDependencies: sass: ^1.78.0 @@ -7861,9 +7784,6 @@ packages: vue-tsc: optional: true - mlly@1.7.1: - resolution: {integrity: sha512-rrVRZRELyQzrIUAVMHxP97kv+G786pHmOKzuFII8zDYahFBS7qnHh2AlYSl1GAHhaMPCz6/oHjVMcfFYgFYHgA==} - mlly@1.7.2: resolution: {integrity: sha512-tN3dvVHYVz4DhSXinXIk7u9syPYaJvio118uomkovAtWBT+RdbP6Lfh/5Lvo519YMmwBafwlh20IPTXIStscpA==} @@ -8045,8 +7965,8 @@ packages: resolution: {integrity: sha512-byy+U7gp+FVwmyzKPYhW2h5l3crpmGsxl7X2s8y43IgxvG4g3QZ6CffDtsNQy1WsmZpQbO+ybo0AlW7TY6DcBQ==} engines: {node: '>= 0.4'} - ofetch@1.4.0: - resolution: {integrity: sha512-MuHgsEhU6zGeX+EMh+8mSMrYTnsqJQQrpM00Q6QHMKNqQ0bKy0B43tk8tL1wg+CnsSTy1kg4Ir2T5Ig6rD+dfQ==} + ofetch@1.4.1: + resolution: {integrity: sha512-QZj2DfGplQAr2oj9KzceK9Hwz6Whxazmn85yYeVuS3u9XTMOGMRx0kO95MQ+vLsj/S/NwBDMMLU5hpxvI6Tklw==} ohash@1.1.4: resolution: {integrity: sha512-FlDryZAahJmEF3VR3w1KogSEdWX3WhA5GPakFx4J81kEAiHyLMpdLLElS8n8dfNadMgAne/MywcvmogzscVt4g==} @@ -8143,8 +8063,8 @@ packages: resolution: {integrity: sha512-ua1L4OgXSBdsu1FPb7F3tYH0F48a6kxvod4pLUlGY9COeJAJQNX/sNH2IiEmsxw7lqYiAwrdHMjz1FctOsyDQg==} engines: {node: '>=18'} - package-manager-detector@0.2.0: - resolution: {integrity: sha512-E385OSk9qDcXhcM9LNSe4sdhx8a9mAPrZ4sMLW+tmxl5ZuGtPUcdFu+MPP2jbgiWAZ6Pfe5soGFMd+0Db5Vrog==} + package-manager-detector@0.2.2: + resolution: {integrity: sha512-VgXbyrSNsml4eHWIvxxG/nTL4wgybMTXCV2Un/+yEc3aDKKU6nQBZjbeP3Pl3qm9Qg92X/1ng4ffvCeD/zwHgg==} param-case@3.0.4: resolution: {integrity: sha512-RXlj7zCYokReqWpOPH9oYivUzLYZ5vAPIfEmCTNViosC78F8F0H9y7T7gG2M39ymgutxF5gcFEsyZQSph9Bp3A==} @@ -8177,14 +8097,14 @@ packages: resolution: {integrity: sha512-1Y1A//QUXEZK7YKz+rD9WydcE1+EuPr6ZBgKecAB8tmoW6UFv0NREVJe1p+jRxtThkcbbKkfwIbWJe/IeE6m2Q==} engines: {node: '>=0.10.0'} - parse5-htmlparser2-tree-adapter@7.0.0: - resolution: {integrity: sha512-B77tOZrqqfUfnVcOrUvfdLbz4pu4RopLD/4vmu3HUPswwTA8OH0EMW9BlWR2B0RCoiZRAHEUu7IxeP1Pd1UU+g==} + parse5-htmlparser2-tree-adapter@7.1.0: + resolution: {integrity: sha512-ruw5xyKs6lrpo9x9rCZqZZnIUntICjQAd0Wsmp396Ul9lN/h+ifgVV1x1gZHi8euej6wTfpqX8j+BFQxF0NS/g==} parse5-parser-stream@7.1.2: resolution: {integrity: sha512-JyeQc9iwFLn5TbvvqACIF/VXG6abODeB3Fwmv/TGdLk2LfbWkaySGY72at4+Ty7EkPZj854u4CrICqNk2qIbow==} - parse5@7.1.2: - resolution: {integrity: sha512-Czj1WaSVpaoj0wbhMzLmWD69anp2WH7FXMB9n1Sy8/ZFF9jolSQVMu1Ij5WIyGmcBmhk7EOndpO4mIpihVqAXw==} + parse5@7.2.0: + resolution: {integrity: sha512-ZkDsAOcxsUMZ4Lz5fVciOehNcJ+Gb8gTzcA4yl3wnc273BAybYWrQ+Ks/OjCjSEpjvQkDSeZbybK9qj2VHHdGA==} parseurl@1.3.3: resolution: {integrity: sha512-CiyeOxFT/JZyN5m0z9PfXw4SCBJ6Sygz1Dpl0wqjlhDEGGBP1GnsUVEL0p63hoG1fcj3fHynXi9NYO4nWOL+qQ==} @@ -8299,9 +8219,6 @@ packages: resolution: {integrity: sha512-saLsH7WeYYPiD25LDuLRRY/i+6HaPYr6G1OUlN39otzkSTxKnubR9RTxS3/Kk50s1g2JTgFwWQDQyplC5/SHZg==} engines: {node: '>= 6'} - pkg-types@1.2.0: - resolution: {integrity: sha512-+ifYuSSqOQ8CqP4MbZA5hDpb97n3E8SVWdJe+Wms9kj745lmd3b7EZJiqvmLwAlmRfjrI7Hi5z3kdBJ93lFNPA==} - pkg-types@1.2.1: resolution: {integrity: sha512-sQoqa8alT3nHjGuTjuKgOnvjo4cljkufdtLMnO2LBP/wRwuDlo1tkaEdMxCRhyGRPacv/ztlZgDPm2b7FAmEvw==} @@ -9177,8 +9094,8 @@ packages: resolution: {integrity: sha512-vfD3pmTzGpufjScBh50YHKzEu2lxBWhVEHsNGoEXmCmn2hKGfeNLYMzCJpe8cD7gqX7TJluOVpBkAequ6dgMmA==} engines: {node: '>=4'} - seemly@0.3.8: - resolution: {integrity: sha512-MW8Qs6vbzo0pHmDpFSYPna+lwpZ6Zk1ancbajw/7E8TKtHdV+1DfZZD+kKJEhG/cAoB/i+LiT+5msZOqj0DwRA==} + seemly@0.3.9: + resolution: {integrity: sha512-bMLcaEqhIViiPbaumjLN8t1y+JpD/N8SiyYOyp0i0W6RgdyLWboIsUWAbZojF//JyerxPZR5Tgda+x3Pdne75A==} semver-compare@1.0.0: resolution: {integrity: sha512-YM3/ITh2MJ5MtzaM429anh+x2jiLVjqILF4m4oyQB18W7Ggea7BfqdH/wGMK7dDiMghv/6WG7znWMwUDzJiXow==} @@ -9624,8 +9541,8 @@ packages: resolution: {integrity: sha512-Vhf+bUa//YSTYKseDiiEuQmhGCoIF3CVBhunm3r/DQnYiGT4JssmnKQc44BIyOZRK2pKjXXAgbhfmbeoC9CJpA==} engines: {node: '>=12.20'} - synckit@0.9.1: - resolution: {integrity: sha512-7gr8p9TQP6RAHusBOSLs46F4564ZrjV8xFmw5zCmgmhGUcw2hxsShhJ6CEiHQMgPDwAQ1fWHPM0ypc4RMAig4A==} + synckit@0.9.2: + resolution: {integrity: sha512-vrozgXDQwYO72vHjUb/HnFbQx1exDjoKzqx23aXEg2a9VIg2TSFZ8FmeZpTjUCFMYw7mpX4BE2SFu8wI7asYsw==} engines: {node: ^14.18.0 || >=16.0.0} system-architecture@0.1.0: @@ -9859,11 +9776,6 @@ packages: engines: {node: '>=14.17'} hasBin: true - typescript@5.6.2: - resolution: {integrity: sha512-NW8ByodCSNCwZeghjN3o+JX5OFH0Ojg6sadjEKY4huZ52TqbJTJnDo5+Tw98lSy63NZvi4n+ez5m2u5d4PkZyw==} - engines: {node: '>=14.17'} - hasBin: true - typescript@5.6.3: resolution: {integrity: sha512-hjcS1mhfuyi4WW8IWtjP7brDrG2cuDZukyrYrSauoXGNgx0S7zceP07adYkJycEr56BOUTNPzbInooiN3fn1qw==} engines: {node: '>=14.17'} @@ -9897,8 +9809,8 @@ packages: resolution: {integrity: sha512-72RFADWFqKmUb2hmmvNODKL3p9hcB6Gt2DOQMis1SEBaV6a4MH8soBvzg+95CYhCKPFedut2JY9bMfrDl9D23g==} engines: {node: '>=14.0'} - undici@6.19.8: - resolution: {integrity: sha512-U8uCCl2x9TK3WANvmBavymRzxbfFYG+tAu+fgx3zxQy3qdagQqBLwJVrdyO1TBfUXvfKveMKJZhpvUYoOjM+4g==} + undici@6.20.0: + resolution: {integrity: sha512-AITZfPuxubm31Sx0vr8bteSalEbs9wQb/BOBi9FPlD9Qpd6HxZ4Q0+hI742jBhkPb4RT2v5MQzaW5VhRVyj+9A==} engines: {node: '>=18.17'} unenv@1.10.0: @@ -10025,8 +9937,8 @@ packages: resolution: {integrity: sha512-4luGP9LMYszMRZwsvyUd9MrxgEGZdZuZgpVQHEEX0lCYFESasVRvZd0EYpCkOIbJKHMuv0LskpXc/8Un+MJzEQ==} hasBin: true - untyped@1.5.0: - resolution: {integrity: sha512-o2Vjmn2dal08BzCcINxSmWuAteReUUiXseii5VRhmxyLF0b21K0iKZQ9fMYK7RWspVkY+0saqaVQNq4roe3Efg==} + untyped@1.5.1: + resolution: {integrity: sha512-reBOnkJBFfBZ8pCKaeHgfZLcehXtM6UTxc+vqs1JvCps0c4amLNp3fhdGBZwYp+VLyoY9n3X5KOP7lCyWBUX9A==} hasBin: true unwasm@0.3.9: @@ -10289,8 +10201,8 @@ packages: vxe-pc-ui@4.2.19: resolution: {integrity: sha512-mWxRhjomh+Ded5xMc8M5LRTX99MaOSSqJpE5MRJp8j/9NqlEsSs0B5Xshw0VE/QPXN18X/kM4FNm5ltKhFAhNg==} - vxe-table@4.7.86: - resolution: {integrity: sha512-7VJjOb/jZf6JMobFER6ZfJI6Mt5L7N85dEOSLR20ZXwfSxRF6vfkXb7kAvuZ4FOXJxT2D7yaAEICPZHPCwwp4g==} + vxe-table@4.7.87: + resolution: {integrity: sha512-9NjDtbg02HZk3OlnwcNNJG3U1YKA3epGeyVPGC8PNeMEYCNbO535s8y3prPVm4PNcD0yJ3q3KMvTEaxAl2il6g==} warning@4.0.3: resolution: {integrity: sha512-rpJyN222KWIvHJ/F53XSZv0Zl/accqHR8et1kpaMTD/fLCRxtV8iX8czMzY7sVZupTI3zcUTg8eycS2kNF9l6w==} @@ -10444,9 +10356,6 @@ packages: resolution: {integrity: sha512-GCPAHLvrIH13+c0SuacwvRYj2SxJXQ4kaVTT5xgL3kPrz56XxkF21IGhjSE1+W0aw7gpBWRGXLCPnPby6lSpmQ==} engines: {node: '>=12'} - xe-utils@3.5.30: - resolution: {integrity: sha512-5Ez6JUANpMakduiTLxrNObzqMebnM4697KvHW5okedkUjXvYgGvkbg0tABTkvwDW/Pb09v7vT68dzBOeAuOu0g==} - xe-utils@3.5.31: resolution: {integrity: sha512-oS4yv8qktvlE0wc9yYkitDidEmThc5qN0UTRvKCvrWnejxbTyIxbwfrdZmPKdKGZtB+/U8cEAMFywLJjHtD11A==} @@ -10537,8 +10446,8 @@ packages: zwitch@2.0.4: resolution: {integrity: sha512-bXE4cR/kVZhKZX/RjPEflHaKVhUVl85noU3v6b8apfQEc1x4A+zBxjZ4lN8LqGd6WZ3dl98pY4o717VFmoPp+A==} - zx@8.1.8: - resolution: {integrity: sha512-m8s48skYQ8EcRz9KXfc7rZCjqlZevOGiNxq5tNhDiGnhOvXKRGxVr+ajUma9B6zxMdHGSSbnjV/R/r7Ue2xd+A==} + zx@8.1.9: + resolution: {integrity: sha512-UHuLHphHmsBYKkAchkSrEN4nzDyagafqC9HUxtc1J7eopaScW6H9dsLJ1lmkAntnLtDTGoM8fa+jrJrXiIfKFA==} engines: {node: '>= 12.17.0'} hasBin: true @@ -10669,7 +10578,7 @@ snapshots: '@antfu/install-pkg@0.4.1': dependencies: - package-manager-detector: 0.2.0 + package-manager-detector: 0.2.2 tinyexec: 0.3.0 '@antfu/utils@0.7.10': {} @@ -10684,52 +10593,52 @@ snapshots: '@ast-grep/napi-darwin-arm64@0.22.6': optional: true - '@ast-grep/napi-darwin-arm64@0.27.2': + '@ast-grep/napi-darwin-arm64@0.27.3': optional: true '@ast-grep/napi-darwin-x64@0.22.6': optional: true - '@ast-grep/napi-darwin-x64@0.27.2': + '@ast-grep/napi-darwin-x64@0.27.3': optional: true '@ast-grep/napi-linux-arm64-gnu@0.22.6': optional: true - '@ast-grep/napi-linux-arm64-gnu@0.27.2': + '@ast-grep/napi-linux-arm64-gnu@0.27.3': optional: true - '@ast-grep/napi-linux-arm64-musl@0.27.2': + '@ast-grep/napi-linux-arm64-musl@0.27.3': optional: true '@ast-grep/napi-linux-x64-gnu@0.22.6': optional: true - '@ast-grep/napi-linux-x64-gnu@0.27.2': + '@ast-grep/napi-linux-x64-gnu@0.27.3': optional: true '@ast-grep/napi-linux-x64-musl@0.22.6': optional: true - '@ast-grep/napi-linux-x64-musl@0.27.2': + '@ast-grep/napi-linux-x64-musl@0.27.3': optional: true '@ast-grep/napi-win32-arm64-msvc@0.22.6': optional: true - '@ast-grep/napi-win32-arm64-msvc@0.27.2': + '@ast-grep/napi-win32-arm64-msvc@0.27.3': optional: true '@ast-grep/napi-win32-ia32-msvc@0.22.6': optional: true - '@ast-grep/napi-win32-ia32-msvc@0.27.2': + '@ast-grep/napi-win32-ia32-msvc@0.27.3': optional: true '@ast-grep/napi-win32-x64-msvc@0.22.6': optional: true - '@ast-grep/napi-win32-x64-msvc@0.27.2': + '@ast-grep/napi-win32-x64-msvc@0.27.3': optional: true '@ast-grep/napi@0.22.6': @@ -10743,39 +10652,37 @@ snapshots: '@ast-grep/napi-win32-ia32-msvc': 0.22.6 '@ast-grep/napi-win32-x64-msvc': 0.22.6 - '@ast-grep/napi@0.27.2': + '@ast-grep/napi@0.27.3': optionalDependencies: - '@ast-grep/napi-darwin-arm64': 0.27.2 - '@ast-grep/napi-darwin-x64': 0.27.2 - '@ast-grep/napi-linux-arm64-gnu': 0.27.2 - '@ast-grep/napi-linux-arm64-musl': 0.27.2 - '@ast-grep/napi-linux-x64-gnu': 0.27.2 - '@ast-grep/napi-linux-x64-musl': 0.27.2 - '@ast-grep/napi-win32-arm64-msvc': 0.27.2 - '@ast-grep/napi-win32-ia32-msvc': 0.27.2 - '@ast-grep/napi-win32-x64-msvc': 0.27.2 + '@ast-grep/napi-darwin-arm64': 0.27.3 + '@ast-grep/napi-darwin-x64': 0.27.3 + '@ast-grep/napi-linux-arm64-gnu': 0.27.3 + '@ast-grep/napi-linux-arm64-musl': 0.27.3 + '@ast-grep/napi-linux-x64-gnu': 0.27.3 + '@ast-grep/napi-linux-x64-musl': 0.27.3 + '@ast-grep/napi-win32-arm64-msvc': 0.27.3 + '@ast-grep/napi-win32-ia32-msvc': 0.27.3 + '@ast-grep/napi-win32-x64-msvc': 0.27.3 '@babel/code-frame@7.25.7': dependencies: '@babel/highlight': 7.25.7 picocolors: 1.1.0 - '@babel/compat-data@7.25.7': {} - '@babel/compat-data@7.25.8': {} - '@babel/core@7.25.7': + '@babel/core@7.25.8': dependencies: '@ampproject/remapping': 2.3.0 '@babel/code-frame': 7.25.7 '@babel/generator': 7.25.7 '@babel/helper-compilation-targets': 7.25.7 - '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.7) + '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.8) '@babel/helpers': 7.25.7 - '@babel/parser': 7.25.7 + '@babel/parser': 7.25.8 '@babel/template': 7.25.7 '@babel/traverse': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 convert-source-map: 2.0.0 debug: 4.3.7 gensync: 1.0.0-beta.2 @@ -10786,14 +10693,14 @@ snapshots: '@babel/generator@7.25.7': dependencies: - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 '@jridgewell/gen-mapping': 0.3.5 '@jridgewell/trace-mapping': 0.3.25 jsesc: 3.0.2 '@babel/helper-annotate-as-pure@7.25.7': dependencies: - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 '@babel/helper-builder-binary-assignment-operator-visitor@7.25.7': dependencies: @@ -10804,35 +10711,35 @@ snapshots: '@babel/helper-compilation-targets@7.25.7': dependencies: - '@babel/compat-data': 7.25.7 + '@babel/compat-data': 7.25.8 '@babel/helper-validator-option': 7.25.7 browserslist: 4.24.0 lru-cache: 5.1.1 semver: 6.3.1 - '@babel/helper-create-class-features-plugin@7.25.7(@babel/core@7.25.7)': + '@babel/helper-create-class-features-plugin@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 '@babel/helper-member-expression-to-functions': 7.25.7 '@babel/helper-optimise-call-expression': 7.25.7 - '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.7) + '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.8) '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 '@babel/traverse': 7.25.7 semver: 6.3.1 transitivePeerDependencies: - supports-color - '@babel/helper-create-regexp-features-plugin@7.25.7(@babel/core@7.25.7)': + '@babel/helper-create-regexp-features-plugin@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 regexpu-core: 6.1.1 semver: 6.3.1 - '@babel/helper-define-polyfill-provider@0.6.2(@babel/core@7.25.7)': + '@babel/helper-define-polyfill-provider@0.6.2(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-compilation-targets': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 debug: 4.3.7 @@ -10851,13 +10758,13 @@ snapshots: '@babel/helper-module-imports@7.25.7': dependencies: '@babel/traverse': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 transitivePeerDependencies: - supports-color - '@babel/helper-module-transforms@7.25.7(@babel/core@7.25.7)': + '@babel/helper-module-transforms@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-module-imports': 7.25.7 '@babel/helper-simple-access': 7.25.7 '@babel/helper-validator-identifier': 7.25.7 @@ -10871,18 +10778,18 @@ snapshots: '@babel/helper-plugin-utils@7.25.7': {} - '@babel/helper-remap-async-to-generator@7.25.7(@babel/core@7.25.7)': + '@babel/helper-remap-async-to-generator@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 '@babel/helper-wrap-function': 7.25.7 '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/helper-replace-supers@7.25.7(@babel/core@7.25.7)': + '@babel/helper-replace-supers@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-member-expression-to-functions': 7.25.7 '@babel/helper-optimise-call-expression': 7.25.7 '@babel/traverse': 7.25.7 @@ -10892,14 +10799,14 @@ snapshots: '@babel/helper-simple-access@7.25.7': dependencies: '@babel/traverse': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 transitivePeerDependencies: - supports-color '@babel/helper-skip-transparent-expression-wrappers@7.25.7': dependencies: '@babel/traverse': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 transitivePeerDependencies: - supports-color @@ -10920,7 +10827,7 @@ snapshots: '@babel/helpers@7.25.7': dependencies: '@babel/template': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 '@babel/highlight@7.25.7': dependencies: @@ -10929,515 +10836,511 @@ snapshots: js-tokens: 4.0.0 picocolors: 1.1.0 - '@babel/parser@7.25.7': - dependencies: - '@babel/types': 7.25.7 - '@babel/parser@7.25.8': dependencies: '@babel/types': 7.25.8 - '@babel/plugin-bugfix-firefox-class-in-computed-class-key@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-bugfix-firefox-class-in-computed-class-key@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-bugfix-safari-class-field-initializer-scope@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-bugfix-safari-class-field-initializer-scope@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-bugfix-safari-id-destructuring-collision-in-function-expression@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-bugfix-safari-id-destructuring-collision-in-function-expression@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-bugfix-v8-spread-parameters-in-optional-chaining@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-bugfix-v8-spread-parameters-in-optional-chaining@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 - '@babel/plugin-transform-optional-chaining': 7.25.8(@babel/core@7.25.7) + '@babel/plugin-transform-optional-chaining': 7.25.8(@babel/core@7.25.8) transitivePeerDependencies: - supports-color - '@babel/plugin-bugfix-v8-static-class-fields-redefine-readonly@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-bugfix-v8-static-class-fields-redefine-readonly@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-proposal-decorators@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-proposal-decorators@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-decorators': 7.25.7(@babel/core@7.25.7) + '@babel/plugin-syntax-decorators': 7.25.7(@babel/core@7.25.8) transitivePeerDependencies: - supports-color - '@babel/plugin-proposal-private-property-in-object@7.21.0-placeholder-for-preset-env.2(@babel/core@7.25.7)': + '@babel/plugin-proposal-private-property-in-object@7.21.0-placeholder-for-preset-env.2(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 - '@babel/plugin-syntax-decorators@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-syntax-decorators@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-import-assertions@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-syntax-import-assertions@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-import-attributes@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-syntax-import-attributes@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-import-meta@7.10.4(@babel/core@7.25.7)': + '@babel/plugin-syntax-import-meta@7.10.4(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-jsx@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-syntax-jsx@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-typescript@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-syntax-typescript@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-unicode-sets-regex@7.18.6(@babel/core@7.25.7)': + '@babel/plugin-syntax-unicode-sets-regex@7.18.6(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-arrow-functions@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-arrow-functions@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-async-generator-functions@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-async-generator-functions@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/helper-remap-async-to-generator': 7.25.7(@babel/core@7.25.7) + '@babel/helper-remap-async-to-generator': 7.25.7(@babel/core@7.25.8) '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-async-to-generator@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-async-to-generator@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-module-imports': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 - '@babel/helper-remap-async-to-generator': 7.25.7(@babel/core@7.25.7) + '@babel/helper-remap-async-to-generator': 7.25.7(@babel/core@7.25.8) transitivePeerDependencies: - supports-color - '@babel/plugin-transform-block-scoped-functions@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-block-scoped-functions@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-block-scoping@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-block-scoping@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-class-properties@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-class-properties@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-class-static-block@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-class-static-block@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-classes@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-classes@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 '@babel/helper-compilation-targets': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 - '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.7) + '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.8) '@babel/traverse': 7.25.7 globals: 11.12.0 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-computed-properties@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-computed-properties@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/template': 7.25.7 - '@babel/plugin-transform-destructuring@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-destructuring@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-dotall-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-dotall-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-duplicate-keys@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-duplicate-keys@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-duplicate-named-capturing-groups-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-duplicate-named-capturing-groups-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-dynamic-import@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-dynamic-import@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-exponentiation-operator@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-exponentiation-operator@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-builder-binary-assignment-operator-visitor': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-export-namespace-from@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-export-namespace-from@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-for-of@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-for-of@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-function-name@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-function-name@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-compilation-targets': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-json-strings@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-json-strings@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-literals@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-literals@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-logical-assignment-operators@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-logical-assignment-operators@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-member-expression-literals@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-member-expression-literals@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-modules-amd@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-modules-amd@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-modules-commonjs@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-modules-commonjs@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-simple-access': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-modules-systemjs@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-modules-systemjs@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-validator-identifier': 7.25.7 '@babel/traverse': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-modules-umd@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-modules-umd@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-module-transforms': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-named-capturing-groups-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-named-capturing-groups-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-new-target@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-new-target@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-nullish-coalescing-operator@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-nullish-coalescing-operator@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-numeric-separator@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-numeric-separator@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-object-rest-spread@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-object-rest-spread@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-compilation-targets': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-parameters': 7.25.7(@babel/core@7.25.7) + '@babel/plugin-transform-parameters': 7.25.7(@babel/core@7.25.8) - '@babel/plugin-transform-object-super@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-object-super@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.7) + '@babel/helper-replace-supers': 7.25.7(@babel/core@7.25.8) transitivePeerDependencies: - supports-color - '@babel/plugin-transform-optional-catch-binding@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-optional-catch-binding@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-optional-chaining@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-optional-chaining@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-parameters@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-parameters@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-private-methods@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-private-methods@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-private-property-in-object@7.25.8(@babel/core@7.25.7)': + '@babel/plugin-transform-private-property-in-object@7.25.8(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-property-literals@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-property-literals@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-regenerator@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-regenerator@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 regenerator-transform: 0.15.2 - '@babel/plugin-transform-reserved-words@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-reserved-words@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-shorthand-properties@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-shorthand-properties@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-spread@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-spread@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 transitivePeerDependencies: - supports-color - '@babel/plugin-transform-sticky-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-sticky-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-template-literals@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-template-literals@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-typeof-symbol@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-typeof-symbol@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-typescript@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-typescript@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-annotate-as-pure': 7.25.7 - '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/helper-create-class-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-skip-transparent-expression-wrappers': 7.25.7 - '@babel/plugin-syntax-typescript': 7.25.7(@babel/core@7.25.7) + '@babel/plugin-syntax-typescript': 7.25.7(@babel/core@7.25.8) transitivePeerDependencies: - supports-color - '@babel/plugin-transform-unicode-escapes@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-unicode-escapes@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-unicode-property-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-unicode-property-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-unicode-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-unicode-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-transform-unicode-sets-regex@7.25.7(@babel/core@7.25.7)': + '@babel/plugin-transform-unicode-sets-regex@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 - '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-create-regexp-features-plugin': 7.25.7(@babel/core@7.25.8) '@babel/helper-plugin-utils': 7.25.7 - '@babel/preset-env@7.25.8(@babel/core@7.25.7)': + '@babel/preset-env@7.25.8(@babel/core@7.25.8)': dependencies: '@babel/compat-data': 7.25.8 - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-compilation-targets': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-validator-option': 7.25.7 - '@babel/plugin-bugfix-firefox-class-in-computed-class-key': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-bugfix-safari-class-field-initializer-scope': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-bugfix-safari-id-destructuring-collision-in-function-expression': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-bugfix-v8-spread-parameters-in-optional-chaining': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-bugfix-v8-static-class-fields-redefine-readonly': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-proposal-private-property-in-object': 7.21.0-placeholder-for-preset-env.2(@babel/core@7.25.7) - '@babel/plugin-syntax-import-assertions': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-syntax-unicode-sets-regex': 7.18.6(@babel/core@7.25.7) - '@babel/plugin-transform-arrow-functions': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-async-generator-functions': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-async-to-generator': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-block-scoped-functions': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-block-scoping': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-class-properties': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-class-static-block': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-classes': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-computed-properties': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-destructuring': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-dotall-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-duplicate-keys': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-duplicate-named-capturing-groups-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-dynamic-import': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-exponentiation-operator': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-export-namespace-from': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-for-of': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-function-name': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-json-strings': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-literals': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-logical-assignment-operators': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-member-expression-literals': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-modules-amd': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-modules-commonjs': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-modules-systemjs': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-modules-umd': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-named-capturing-groups-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-new-target': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-nullish-coalescing-operator': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-numeric-separator': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-object-rest-spread': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-object-super': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-optional-catch-binding': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-optional-chaining': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-parameters': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-private-methods': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-private-property-in-object': 7.25.8(@babel/core@7.25.7) - '@babel/plugin-transform-property-literals': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-regenerator': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-reserved-words': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-shorthand-properties': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-spread': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-sticky-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-template-literals': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-typeof-symbol': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-unicode-escapes': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-unicode-property-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-unicode-regex': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-unicode-sets-regex': 7.25.7(@babel/core@7.25.7) - '@babel/preset-modules': 0.1.6-no-external-plugins(@babel/core@7.25.7) - babel-plugin-polyfill-corejs2: 0.4.11(@babel/core@7.25.7) - babel-plugin-polyfill-corejs3: 0.10.6(@babel/core@7.25.7) - babel-plugin-polyfill-regenerator: 0.6.2(@babel/core@7.25.7) + '@babel/plugin-bugfix-firefox-class-in-computed-class-key': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-bugfix-safari-class-field-initializer-scope': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-bugfix-safari-id-destructuring-collision-in-function-expression': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-bugfix-v8-spread-parameters-in-optional-chaining': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-bugfix-v8-static-class-fields-redefine-readonly': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-proposal-private-property-in-object': 7.21.0-placeholder-for-preset-env.2(@babel/core@7.25.8) + '@babel/plugin-syntax-import-assertions': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-syntax-unicode-sets-regex': 7.18.6(@babel/core@7.25.8) + '@babel/plugin-transform-arrow-functions': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-async-generator-functions': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-async-to-generator': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-block-scoped-functions': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-block-scoping': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-class-properties': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-class-static-block': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-classes': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-computed-properties': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-destructuring': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-dotall-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-duplicate-keys': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-duplicate-named-capturing-groups-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-dynamic-import': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-exponentiation-operator': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-export-namespace-from': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-for-of': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-function-name': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-json-strings': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-literals': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-logical-assignment-operators': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-member-expression-literals': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-modules-amd': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-modules-commonjs': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-modules-systemjs': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-modules-umd': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-named-capturing-groups-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-new-target': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-nullish-coalescing-operator': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-numeric-separator': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-object-rest-spread': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-object-super': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-optional-catch-binding': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-optional-chaining': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-parameters': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-private-methods': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-private-property-in-object': 7.25.8(@babel/core@7.25.8) + '@babel/plugin-transform-property-literals': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-regenerator': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-reserved-words': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-shorthand-properties': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-spread': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-sticky-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-template-literals': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-typeof-symbol': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-unicode-escapes': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-unicode-property-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-unicode-regex': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-unicode-sets-regex': 7.25.7(@babel/core@7.25.8) + '@babel/preset-modules': 0.1.6-no-external-plugins(@babel/core@7.25.8) + babel-plugin-polyfill-corejs2: 0.4.11(@babel/core@7.25.8) + babel-plugin-polyfill-corejs3: 0.10.6(@babel/core@7.25.8) + babel-plugin-polyfill-regenerator: 0.6.2(@babel/core@7.25.8) core-js-compat: 3.38.1 semver: 6.3.1 transitivePeerDependencies: - supports-color - '@babel/preset-modules@0.1.6-no-external-plugins(@babel/core@7.25.7)': + '@babel/preset-modules@0.1.6-no-external-plugins(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/types': 7.25.8 esutils: 2.0.3 - '@babel/preset-typescript@7.25.7(@babel/core@7.25.7)': + '@babel/preset-typescript@7.25.7(@babel/core@7.25.8)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-plugin-utils': 7.25.7 '@babel/helper-validator-option': 7.25.7 - '@babel/plugin-syntax-jsx': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-modules-commonjs': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.7) + '@babel/plugin-syntax-jsx': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-modules-commonjs': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.8) transitivePeerDependencies: - supports-color @@ -11445,32 +11348,26 @@ snapshots: dependencies: regenerator-runtime: 0.14.1 - '@babel/standalone@7.25.7': {} + '@babel/standalone@7.25.8': {} '@babel/template@7.25.7': dependencies: '@babel/code-frame': 7.25.7 '@babel/parser': 7.25.8 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 '@babel/traverse@7.25.7': dependencies: '@babel/code-frame': 7.25.7 '@babel/generator': 7.25.7 - '@babel/parser': 7.25.7 + '@babel/parser': 7.25.8 '@babel/template': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 debug: 4.3.7 globals: 11.12.0 transitivePeerDependencies: - supports-color - '@babel/types@7.25.7': - dependencies: - '@babel/helper-string-parser': 7.25.7 - '@babel/helper-validator-identifier': 7.25.7 - to-fast-properties: 2.0.0 - '@babel/types@7.25.8': dependencies: '@babel/helper-string-parser': 7.25.7 @@ -11538,7 +11435,7 @@ snapshots: fs-extra: 7.0.1 mri: 1.2.0 p-limit: 2.3.0 - package-manager-detector: 0.2.0 + package-manager-detector: 0.2.2 picocolors: 1.1.0 resolve-from: 5.0.0 semver: 7.6.3 @@ -11761,58 +11658,58 @@ snapshots: '@cspell/cspell-bundled-dicts@8.15.1': dependencies: - '@cspell/dict-ada': 4.0.2 - '@cspell/dict-aws': 4.0.4 - '@cspell/dict-bash': 4.1.5 - '@cspell/dict-companies': 3.1.4 - '@cspell/dict-cpp': 5.1.19 - '@cspell/dict-cryptocurrencies': 5.0.0 - '@cspell/dict-csharp': 4.0.2 - '@cspell/dict-css': 4.0.13 - '@cspell/dict-dart': 2.2.1 - '@cspell/dict-django': 4.1.0 - '@cspell/dict-docker': 1.1.7 - '@cspell/dict-dotnet': 5.0.5 - '@cspell/dict-elixir': 4.0.3 - '@cspell/dict-en-common-misspellings': 2.0.4 + '@cspell/dict-ada': 4.0.5 + '@cspell/dict-aws': 4.0.7 + '@cspell/dict-bash': 4.1.8 + '@cspell/dict-companies': 3.1.7 + '@cspell/dict-cpp': 5.1.22 + '@cspell/dict-cryptocurrencies': 5.0.3 + '@cspell/dict-csharp': 4.0.5 + '@cspell/dict-css': 4.0.16 + '@cspell/dict-dart': 2.2.4 + '@cspell/dict-django': 4.1.3 + '@cspell/dict-docker': 1.1.10 + '@cspell/dict-dotnet': 5.0.8 + '@cspell/dict-elixir': 4.0.6 + '@cspell/dict-en-common-misspellings': 2.0.7 '@cspell/dict-en-gb': 1.1.33 - '@cspell/dict-en_us': 4.3.23 - '@cspell/dict-filetypes': 3.0.4 + '@cspell/dict-en_us': 4.3.26 + '@cspell/dict-filetypes': 3.0.7 '@cspell/dict-flutter': 1.0.3 - '@cspell/dict-fonts': 4.0.0 + '@cspell/dict-fonts': 4.0.3 '@cspell/dict-fsharp': 1.0.4 - '@cspell/dict-fullstack': 3.2.0 - '@cspell/dict-gaming-terms': 1.0.5 + '@cspell/dict-fullstack': 3.2.3 + '@cspell/dict-gaming-terms': 1.0.8 '@cspell/dict-git': 3.0.3 - '@cspell/dict-golang': 6.0.13 + '@cspell/dict-golang': 6.0.16 '@cspell/dict-google': 1.0.4 - '@cspell/dict-haskell': 4.0.1 - '@cspell/dict-html': 4.0.6 - '@cspell/dict-html-symbol-entities': 4.0.0 - '@cspell/dict-java': 5.0.7 + '@cspell/dict-haskell': 4.0.4 + '@cspell/dict-html': 4.0.9 + '@cspell/dict-html-symbol-entities': 4.0.3 + '@cspell/dict-java': 5.0.10 '@cspell/dict-julia': 1.0.4 - '@cspell/dict-k8s': 1.0.6 - '@cspell/dict-latex': 4.0.0 - '@cspell/dict-lorem-ipsum': 4.0.0 - '@cspell/dict-lua': 4.0.3 - '@cspell/dict-makefile': 1.0.0 - '@cspell/dict-monkeyc': 1.0.6 - '@cspell/dict-node': 5.0.1 - '@cspell/dict-npm': 5.1.5 - '@cspell/dict-php': 4.0.10 - '@cspell/dict-powershell': 5.0.10 - '@cspell/dict-public-licenses': 2.0.8 - '@cspell/dict-python': 4.2.8 - '@cspell/dict-r': 2.0.1 - '@cspell/dict-ruby': 5.0.4 - '@cspell/dict-rust': 4.0.6 - '@cspell/dict-scala': 5.0.3 - '@cspell/dict-software-terms': 4.1.7 - '@cspell/dict-sql': 2.1.5 - '@cspell/dict-svelte': 1.0.2 - '@cspell/dict-swift': 2.0.1 - '@cspell/dict-terraform': 1.0.2 - '@cspell/dict-typescript': 3.1.6 + '@cspell/dict-k8s': 1.0.9 + '@cspell/dict-latex': 4.0.3 + '@cspell/dict-lorem-ipsum': 4.0.3 + '@cspell/dict-lua': 4.0.6 + '@cspell/dict-makefile': 1.0.3 + '@cspell/dict-monkeyc': 1.0.9 + '@cspell/dict-node': 5.0.4 + '@cspell/dict-npm': 5.1.8 + '@cspell/dict-php': 4.0.13 + '@cspell/dict-powershell': 5.0.13 + '@cspell/dict-public-licenses': 2.0.11 + '@cspell/dict-python': 4.2.11 + '@cspell/dict-r': 2.0.4 + '@cspell/dict-ruby': 5.0.7 + '@cspell/dict-rust': 4.0.9 + '@cspell/dict-scala': 5.0.6 + '@cspell/dict-software-terms': 4.1.10 + '@cspell/dict-sql': 2.1.8 + '@cspell/dict-svelte': 1.0.5 + '@cspell/dict-swift': 2.0.4 + '@cspell/dict-terraform': 1.0.5 + '@cspell/dict-typescript': 3.1.9 '@cspell/dict-vue': 3.0.3 '@cspell/cspell-json-reporter@8.15.1': @@ -11829,113 +11726,113 @@ snapshots: '@cspell/cspell-types@8.15.1': {} - '@cspell/dict-ada@4.0.2': {} + '@cspell/dict-ada@4.0.5': {} - '@cspell/dict-aws@4.0.4': {} + '@cspell/dict-aws@4.0.7': {} - '@cspell/dict-bash@4.1.5': {} + '@cspell/dict-bash@4.1.8': {} - '@cspell/dict-companies@3.1.4': {} + '@cspell/dict-companies@3.1.7': {} - '@cspell/dict-cpp@5.1.19': {} + '@cspell/dict-cpp@5.1.22': {} - '@cspell/dict-cryptocurrencies@5.0.0': {} + '@cspell/dict-cryptocurrencies@5.0.3': {} - '@cspell/dict-csharp@4.0.2': {} + '@cspell/dict-csharp@4.0.5': {} - '@cspell/dict-css@4.0.13': {} + '@cspell/dict-css@4.0.16': {} - '@cspell/dict-dart@2.2.1': {} + '@cspell/dict-dart@2.2.4': {} - '@cspell/dict-data-science@2.0.2': {} + '@cspell/dict-data-science@2.0.5': {} - '@cspell/dict-django@4.1.0': {} + '@cspell/dict-django@4.1.3': {} - '@cspell/dict-docker@1.1.7': {} + '@cspell/dict-docker@1.1.10': {} - '@cspell/dict-dotnet@5.0.5': {} + '@cspell/dict-dotnet@5.0.8': {} - '@cspell/dict-elixir@4.0.3': {} + '@cspell/dict-elixir@4.0.6': {} - '@cspell/dict-en-common-misspellings@2.0.4': {} + '@cspell/dict-en-common-misspellings@2.0.7': {} '@cspell/dict-en-gb@1.1.33': {} - '@cspell/dict-en_us@4.3.23': {} + '@cspell/dict-en_us@4.3.26': {} - '@cspell/dict-filetypes@3.0.4': {} + '@cspell/dict-filetypes@3.0.7': {} '@cspell/dict-flutter@1.0.3': {} - '@cspell/dict-fonts@4.0.0': {} + '@cspell/dict-fonts@4.0.3': {} '@cspell/dict-fsharp@1.0.4': {} - '@cspell/dict-fullstack@3.2.0': {} + '@cspell/dict-fullstack@3.2.3': {} - '@cspell/dict-gaming-terms@1.0.5': {} + '@cspell/dict-gaming-terms@1.0.8': {} '@cspell/dict-git@3.0.3': {} - '@cspell/dict-golang@6.0.13': {} + '@cspell/dict-golang@6.0.16': {} '@cspell/dict-google@1.0.4': {} - '@cspell/dict-haskell@4.0.1': {} + '@cspell/dict-haskell@4.0.4': {} - '@cspell/dict-html-symbol-entities@4.0.0': {} + '@cspell/dict-html-symbol-entities@4.0.3': {} - '@cspell/dict-html@4.0.6': {} + '@cspell/dict-html@4.0.9': {} - '@cspell/dict-java@5.0.7': {} + '@cspell/dict-java@5.0.10': {} '@cspell/dict-julia@1.0.4': {} - '@cspell/dict-k8s@1.0.6': {} + '@cspell/dict-k8s@1.0.9': {} - '@cspell/dict-latex@4.0.0': {} + '@cspell/dict-latex@4.0.3': {} - '@cspell/dict-lorem-ipsum@4.0.0': {} + '@cspell/dict-lorem-ipsum@4.0.3': {} - '@cspell/dict-lua@4.0.3': {} + '@cspell/dict-lua@4.0.6': {} - '@cspell/dict-makefile@1.0.0': {} + '@cspell/dict-makefile@1.0.3': {} - '@cspell/dict-monkeyc@1.0.6': {} + '@cspell/dict-monkeyc@1.0.9': {} - '@cspell/dict-node@5.0.1': {} + '@cspell/dict-node@5.0.4': {} - '@cspell/dict-npm@5.1.5': {} + '@cspell/dict-npm@5.1.8': {} - '@cspell/dict-php@4.0.10': {} + '@cspell/dict-php@4.0.13': {} - '@cspell/dict-powershell@5.0.10': {} + '@cspell/dict-powershell@5.0.13': {} - '@cspell/dict-public-licenses@2.0.8': {} + '@cspell/dict-public-licenses@2.0.11': {} - '@cspell/dict-python@4.2.8': + '@cspell/dict-python@4.2.11': dependencies: - '@cspell/dict-data-science': 2.0.2 + '@cspell/dict-data-science': 2.0.5 - '@cspell/dict-r@2.0.1': {} + '@cspell/dict-r@2.0.4': {} - '@cspell/dict-ruby@5.0.4': {} + '@cspell/dict-ruby@5.0.7': {} - '@cspell/dict-rust@4.0.6': {} + '@cspell/dict-rust@4.0.9': {} - '@cspell/dict-scala@5.0.3': {} + '@cspell/dict-scala@5.0.6': {} - '@cspell/dict-software-terms@4.1.7': {} + '@cspell/dict-software-terms@4.1.10': {} - '@cspell/dict-sql@2.1.5': {} + '@cspell/dict-sql@2.1.8': {} - '@cspell/dict-svelte@1.0.2': {} + '@cspell/dict-svelte@1.0.5': {} - '@cspell/dict-swift@2.0.1': {} + '@cspell/dict-swift@2.0.4': {} - '@cspell/dict-terraform@1.0.2': {} + '@cspell/dict-terraform@1.0.5': {} - '@cspell/dict-typescript@3.1.6': {} + '@cspell/dict-typescript@3.1.9': {} '@cspell/dict-vue@3.0.3': {} @@ -11976,23 +11873,12 @@ snapshots: '@csstools/css-parser-algorithms': 3.0.2(@csstools/css-tokenizer@3.0.2) '@csstools/css-tokenizer': 3.0.2 - '@csstools/css-parser-algorithms@3.0.1(@csstools/css-tokenizer@3.0.1)': - dependencies: - '@csstools/css-tokenizer': 3.0.1 - '@csstools/css-parser-algorithms@3.0.2(@csstools/css-tokenizer@3.0.2)': dependencies: '@csstools/css-tokenizer': 3.0.2 - '@csstools/css-tokenizer@3.0.1': {} - '@csstools/css-tokenizer@3.0.2': {} - '@csstools/media-query-list-parser@3.0.1(@csstools/css-parser-algorithms@3.0.1(@csstools/css-tokenizer@3.0.1))(@csstools/css-tokenizer@3.0.1)': - dependencies: - '@csstools/css-parser-algorithms': 3.0.1(@csstools/css-tokenizer@3.0.1) - '@csstools/css-tokenizer': 3.0.1 - '@csstools/media-query-list-parser@3.0.1(@csstools/css-parser-algorithms@3.0.2(@csstools/css-tokenizer@3.0.2))(@csstools/css-tokenizer@3.0.2)': dependencies: '@csstools/css-parser-algorithms': 3.0.2(@csstools/css-tokenizer@3.0.2) @@ -12264,7 +12150,7 @@ snapshots: '@esbuild/aix-ppc64@0.21.5': optional: true - '@esbuild/aix-ppc64@0.23.1': + '@esbuild/aix-ppc64@0.24.0': optional: true '@esbuild/android-arm64@0.19.12': @@ -12276,7 +12162,7 @@ snapshots: '@esbuild/android-arm64@0.21.5': optional: true - '@esbuild/android-arm64@0.23.1': + '@esbuild/android-arm64@0.24.0': optional: true '@esbuild/android-arm@0.19.12': @@ -12288,7 +12174,7 @@ snapshots: '@esbuild/android-arm@0.21.5': optional: true - '@esbuild/android-arm@0.23.1': + '@esbuild/android-arm@0.24.0': optional: true '@esbuild/android-x64@0.19.12': @@ -12300,7 +12186,7 @@ snapshots: '@esbuild/android-x64@0.21.5': optional: true - '@esbuild/android-x64@0.23.1': + '@esbuild/android-x64@0.24.0': optional: true '@esbuild/darwin-arm64@0.19.12': @@ -12312,7 +12198,7 @@ snapshots: '@esbuild/darwin-arm64@0.21.5': optional: true - '@esbuild/darwin-arm64@0.23.1': + '@esbuild/darwin-arm64@0.24.0': optional: true '@esbuild/darwin-x64@0.19.12': @@ -12324,7 +12210,7 @@ snapshots: '@esbuild/darwin-x64@0.21.5': optional: true - '@esbuild/darwin-x64@0.23.1': + '@esbuild/darwin-x64@0.24.0': optional: true '@esbuild/freebsd-arm64@0.19.12': @@ -12336,7 +12222,7 @@ snapshots: '@esbuild/freebsd-arm64@0.21.5': optional: true - '@esbuild/freebsd-arm64@0.23.1': + '@esbuild/freebsd-arm64@0.24.0': optional: true '@esbuild/freebsd-x64@0.19.12': @@ -12348,7 +12234,7 @@ snapshots: '@esbuild/freebsd-x64@0.21.5': optional: true - '@esbuild/freebsd-x64@0.23.1': + '@esbuild/freebsd-x64@0.24.0': optional: true '@esbuild/linux-arm64@0.19.12': @@ -12360,7 +12246,7 @@ snapshots: '@esbuild/linux-arm64@0.21.5': optional: true - '@esbuild/linux-arm64@0.23.1': + '@esbuild/linux-arm64@0.24.0': optional: true '@esbuild/linux-arm@0.19.12': @@ -12372,7 +12258,7 @@ snapshots: '@esbuild/linux-arm@0.21.5': optional: true - '@esbuild/linux-arm@0.23.1': + '@esbuild/linux-arm@0.24.0': optional: true '@esbuild/linux-ia32@0.19.12': @@ -12384,7 +12270,7 @@ snapshots: '@esbuild/linux-ia32@0.21.5': optional: true - '@esbuild/linux-ia32@0.23.1': + '@esbuild/linux-ia32@0.24.0': optional: true '@esbuild/linux-loong64@0.19.12': @@ -12396,7 +12282,7 @@ snapshots: '@esbuild/linux-loong64@0.21.5': optional: true - '@esbuild/linux-loong64@0.23.1': + '@esbuild/linux-loong64@0.24.0': optional: true '@esbuild/linux-mips64el@0.19.12': @@ -12408,7 +12294,7 @@ snapshots: '@esbuild/linux-mips64el@0.21.5': optional: true - '@esbuild/linux-mips64el@0.23.1': + '@esbuild/linux-mips64el@0.24.0': optional: true '@esbuild/linux-ppc64@0.19.12': @@ -12420,7 +12306,7 @@ snapshots: '@esbuild/linux-ppc64@0.21.5': optional: true - '@esbuild/linux-ppc64@0.23.1': + '@esbuild/linux-ppc64@0.24.0': optional: true '@esbuild/linux-riscv64@0.19.12': @@ -12432,7 +12318,7 @@ snapshots: '@esbuild/linux-riscv64@0.21.5': optional: true - '@esbuild/linux-riscv64@0.23.1': + '@esbuild/linux-riscv64@0.24.0': optional: true '@esbuild/linux-s390x@0.19.12': @@ -12444,7 +12330,7 @@ snapshots: '@esbuild/linux-s390x@0.21.5': optional: true - '@esbuild/linux-s390x@0.23.1': + '@esbuild/linux-s390x@0.24.0': optional: true '@esbuild/linux-x64@0.19.12': @@ -12456,7 +12342,7 @@ snapshots: '@esbuild/linux-x64@0.21.5': optional: true - '@esbuild/linux-x64@0.23.1': + '@esbuild/linux-x64@0.24.0': optional: true '@esbuild/netbsd-x64@0.19.12': @@ -12468,10 +12354,10 @@ snapshots: '@esbuild/netbsd-x64@0.21.5': optional: true - '@esbuild/netbsd-x64@0.23.1': + '@esbuild/netbsd-x64@0.24.0': optional: true - '@esbuild/openbsd-arm64@0.23.1': + '@esbuild/openbsd-arm64@0.24.0': optional: true '@esbuild/openbsd-x64@0.19.12': @@ -12483,7 +12369,7 @@ snapshots: '@esbuild/openbsd-x64@0.21.5': optional: true - '@esbuild/openbsd-x64@0.23.1': + '@esbuild/openbsd-x64@0.24.0': optional: true '@esbuild/sunos-x64@0.19.12': @@ -12495,7 +12381,7 @@ snapshots: '@esbuild/sunos-x64@0.21.5': optional: true - '@esbuild/sunos-x64@0.23.1': + '@esbuild/sunos-x64@0.24.0': optional: true '@esbuild/win32-arm64@0.19.12': @@ -12507,7 +12393,7 @@ snapshots: '@esbuild/win32-arm64@0.21.5': optional: true - '@esbuild/win32-arm64@0.23.1': + '@esbuild/win32-arm64@0.24.0': optional: true '@esbuild/win32-ia32@0.19.12': @@ -12519,7 +12405,7 @@ snapshots: '@esbuild/win32-ia32@0.21.5': optional: true - '@esbuild/win32-ia32@0.23.1': + '@esbuild/win32-ia32@0.24.0': optional: true '@esbuild/win32-x64@0.19.12': @@ -12531,12 +12417,12 @@ snapshots: '@esbuild/win32-x64@0.21.5': optional: true - '@esbuild/win32-x64@0.23.1': + '@esbuild/win32-x64@0.24.0': optional: true - '@eslint-community/eslint-utils@4.4.0(eslint@9.12.0(jiti@2.2.1))': + '@eslint-community/eslint-utils@4.4.0(eslint@9.12.0(jiti@2.3.3))': dependencies: - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) eslint-visitor-keys: 3.4.3 '@eslint-community/regexpp@4.11.1': {} @@ -12610,7 +12496,7 @@ snapshots: '@humanwhocodes/retry@0.3.1': {} - '@iconify-json/logos@1.2.2': + '@iconify-json/logos@1.2.3': dependencies: '@iconify/types': 2.0.0 @@ -12641,7 +12527,7 @@ snapshots: debug: 4.3.7 kolorist: 1.8.0 local-pkg: 0.5.0 - mlly: 1.7.1 + mlly: 1.7.2 transitivePeerDependencies: - supports-color @@ -12666,7 +12552,7 @@ snapshots: escodegen: 2.1.0 estree-walker: 2.0.2 jsonc-eslint-parser: 2.4.0 - mlly: 1.7.1 + mlly: 1.7.2 source-map-js: 1.2.1 yaml-eslint-parser: 1.2.3 optionalDependencies: @@ -12691,9 +12577,9 @@ snapshots: '@intlify/shared@10.0.4': {} - '@intlify/unplugin-vue-i18n@5.2.0(@vue/compiler-dom@3.5.12)(eslint@9.12.0(jiti@2.2.1))(rollup@4.24.0)(typescript@5.6.3)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3))': + '@intlify/unplugin-vue-i18n@5.2.0(@vue/compiler-dom@3.5.12)(eslint@9.12.0(jiti@2.3.3))(rollup@4.24.0)(typescript@5.6.3)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3))': dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@intlify/bundle-utils': 9.0.0-beta.0(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3))) '@intlify/shared': 10.0.0 '@intlify/vue-i18n-extensions': 7.0.0(@intlify/shared@10.0.0)(@vue/compiler-dom@3.5.12)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3)) @@ -12721,7 +12607,7 @@ snapshots: '@intlify/vue-i18n-extensions@7.0.0(@intlify/shared@10.0.0)(@vue/compiler-dom@3.5.12)(vue-i18n@10.0.4(vue@3.5.12(typescript@5.6.3)))(vue@3.5.12(typescript@5.6.3))': dependencies: - '@babel/parser': 7.25.7 + '@babel/parser': 7.25.8 optionalDependencies: '@intlify/shared': 10.0.0 '@vue/compiler-dom': 3.5.12 @@ -12763,9 +12649,9 @@ snapshots: '@jspm/generator@2.3.1': dependencies: - '@babel/core': 7.25.7 - '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.7) - '@babel/preset-typescript': 7.25.7(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.8) + '@babel/preset-typescript': 7.25.7(@babel/core@7.25.8) '@jspm/import-map': 1.1.0 es-module-lexer: 1.5.4 make-fetch-happen: 8.0.14 @@ -12970,9 +12856,9 @@ snapshots: mkdirp: 1.0.4 rimraf: 3.0.2 - '@nuxt/kit@3.13.2(rollup@3.29.5)': + '@nuxt/kit@3.13.2(rollup@4.24.0)': dependencies: - '@nuxt/schema': 3.13.2(rollup@3.29.5) + '@nuxt/schema': 3.13.2(rollup@4.24.0) c12: 1.11.2 consola: 3.2.3 defu: 6.1.4 @@ -12983,22 +12869,22 @@ snapshots: jiti: 1.21.6 klona: 2.0.6 knitwork: 1.1.0 - mlly: 1.7.1 + mlly: 1.7.2 pathe: 1.1.2 - pkg-types: 1.2.0 + pkg-types: 1.2.1 scule: 1.3.0 semver: 7.6.3 ufo: 1.5.4 unctx: 2.3.1 - unimport: 3.13.1(rollup@3.29.5) - untyped: 1.5.0 + unimport: 3.13.1(rollup@4.24.0) + untyped: 1.5.1 transitivePeerDependencies: - magicast - rollup - supports-color - webpack-sources - '@nuxt/schema@3.13.2(rollup@3.29.5)': + '@nuxt/schema@3.13.2(rollup@4.24.0)': dependencies: compatx: 0.1.8 consola: 3.2.3 @@ -13010,8 +12896,8 @@ snapshots: std-env: 3.7.0 ufo: 1.5.4 uncrypto: 0.1.3 - unimport: 3.13.1(rollup@3.29.5) - untyped: 1.5.0 + unimport: 3.13.1(rollup@4.24.0) + untyped: 1.5.1 transitivePeerDependencies: - rollup - supports-color @@ -13123,9 +13009,9 @@ snapshots: optionalDependencies: rollup: 4.24.0 - '@rollup/plugin-babel@5.3.1(@babel/core@7.25.7)(rollup@2.79.2)': + '@rollup/plugin-babel@5.3.1(@babel/core@7.25.8)(rollup@2.79.2)': dependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-module-imports': 7.25.7 '@rollup/pluginutils': 3.1.0(rollup@2.79.2) rollup: 2.79.2 @@ -13402,9 +13288,9 @@ snapshots: '@stylistic/stylelint-plugin@3.1.1(stylelint@16.10.0(typescript@5.6.3))': dependencies: - '@csstools/css-parser-algorithms': 3.0.1(@csstools/css-tokenizer@3.0.1) - '@csstools/css-tokenizer': 3.0.1 - '@csstools/media-query-list-parser': 3.0.1(@csstools/css-parser-algorithms@3.0.1(@csstools/css-tokenizer@3.0.1))(@csstools/css-tokenizer@3.0.1) + '@csstools/css-parser-algorithms': 3.0.2(@csstools/css-tokenizer@3.0.2) + '@csstools/css-tokenizer': 3.0.2 + '@csstools/media-query-list-parser': 3.0.1(@csstools/css-parser-algorithms@3.0.2(@csstools/css-tokenizer@3.0.2))(@csstools/css-tokenizer@3.0.2) is-plain-object: 5.0.0 postcss-selector-parser: 6.1.2 postcss-value-parser: 4.2.0 @@ -13484,7 +13370,7 @@ snapshots: '@types/conventional-commits-parser@5.0.0': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 '@types/eslint@9.6.1': dependencies: @@ -13498,7 +13384,7 @@ snapshots: '@types/fs-extra@11.0.4': dependencies: '@types/jsonfile': 6.1.4 - '@types/node': 22.7.4 + '@types/node': 22.7.5 optional: true '@types/hast@3.0.4': @@ -13509,18 +13395,18 @@ snapshots: '@types/http-proxy@1.17.15': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 '@types/json-schema@7.0.15': {} '@types/jsonfile@6.1.4': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 optional: true '@types/jsonwebtoken@9.0.7': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 '@types/katex@0.16.7': {} @@ -13551,10 +13437,6 @@ snapshots: '@types/node@12.20.55': {} - '@types/node@22.7.4': - dependencies: - undici-types: 6.19.8 - '@types/node@22.7.5': dependencies: undici-types: 6.19.8 @@ -13571,11 +13453,11 @@ snapshots: '@types/qrcode@1.5.5': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 '@types/readdir-glob@1.1.5': dependencies: - '@types/node': 22.7.4 + '@types/node': 22.7.5 '@types/resolve@1.20.2': {} @@ -13589,15 +13471,15 @@ snapshots: '@types/web-bluetooth@0.0.20': {} - '@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': + '@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)': dependencies: '@eslint-community/regexpp': 4.11.1 - '@typescript-eslint/parser': 8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/parser': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) '@typescript-eslint/scope-manager': 8.8.1 - '@typescript-eslint/type-utils': 8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) - '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/type-utils': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) + '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) '@typescript-eslint/visitor-keys': 8.8.1 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) graphemer: 1.4.0 ignore: 5.3.2 natural-compare: 1.4.0 @@ -13607,14 +13489,14 @@ snapshots: transitivePeerDependencies: - supports-color - '@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': + '@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)': dependencies: '@typescript-eslint/scope-manager': 8.8.1 '@typescript-eslint/types': 8.8.1 '@typescript-eslint/typescript-estree': 8.8.1(typescript@5.6.3) '@typescript-eslint/visitor-keys': 8.8.1 debug: 4.3.7 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) optionalDependencies: typescript: 5.6.3 transitivePeerDependencies: @@ -13625,20 +13507,15 @@ snapshots: '@typescript-eslint/types': 7.18.0 '@typescript-eslint/visitor-keys': 7.18.0 - '@typescript-eslint/scope-manager@8.8.0': - dependencies: - '@typescript-eslint/types': 8.8.0 - '@typescript-eslint/visitor-keys': 8.8.0 - '@typescript-eslint/scope-manager@8.8.1': dependencies: '@typescript-eslint/types': 8.8.1 '@typescript-eslint/visitor-keys': 8.8.1 - '@typescript-eslint/type-utils@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': + '@typescript-eslint/type-utils@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)': dependencies: '@typescript-eslint/typescript-estree': 8.8.1(typescript@5.6.3) - '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) debug: 4.3.7 ts-api-utils: 1.3.0(typescript@5.6.3) optionalDependencies: @@ -13649,8 +13526,6 @@ snapshots: '@typescript-eslint/types@7.18.0': {} - '@typescript-eslint/types@8.8.0': {} - '@typescript-eslint/types@8.8.1': {} '@typescript-eslint/typescript-estree@7.18.0(typescript@5.6.3)': @@ -13668,21 +13543,6 @@ snapshots: transitivePeerDependencies: - supports-color - '@typescript-eslint/typescript-estree@8.8.0(typescript@5.6.3)': - dependencies: - '@typescript-eslint/types': 8.8.0 - '@typescript-eslint/visitor-keys': 8.8.0 - debug: 4.3.7 - fast-glob: 3.3.2 - is-glob: 4.0.3 - minimatch: 9.0.5 - semver: 7.6.3 - ts-api-utils: 1.3.0(typescript@5.6.3) - optionalDependencies: - typescript: 5.6.3 - transitivePeerDependencies: - - supports-color - '@typescript-eslint/typescript-estree@8.8.1(typescript@5.6.3)': dependencies: '@typescript-eslint/types': 8.8.1 @@ -13698,35 +13558,24 @@ snapshots: transitivePeerDependencies: - supports-color - '@typescript-eslint/utils@7.18.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': + '@typescript-eslint/utils@7.18.0(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)': dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@typescript-eslint/scope-manager': 7.18.0 '@typescript-eslint/types': 7.18.0 '@typescript-eslint/typescript-estree': 7.18.0(typescript@5.6.3) - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) transitivePeerDependencies: - supports-color - typescript - '@typescript-eslint/utils@8.8.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': + '@typescript-eslint/utils@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)': dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) - '@typescript-eslint/scope-manager': 8.8.0 - '@typescript-eslint/types': 8.8.0 - '@typescript-eslint/typescript-estree': 8.8.0(typescript@5.6.3) - eslint: 9.12.0(jiti@2.2.1) - transitivePeerDependencies: - - supports-color - - typescript - - '@typescript-eslint/utils@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)': - dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@typescript-eslint/scope-manager': 8.8.1 '@typescript-eslint/types': 8.8.1 '@typescript-eslint/typescript-estree': 8.8.1(typescript@5.6.3) - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) transitivePeerDependencies: - supports-color - typescript @@ -13736,11 +13585,6 @@ snapshots: '@typescript-eslint/types': 7.18.0 eslint-visitor-keys: 3.4.3 - '@typescript-eslint/visitor-keys@8.8.0': - dependencies: - '@typescript-eslint/types': 8.8.0 - eslint-visitor-keys: 3.4.3 - '@typescript-eslint/visitor-keys@8.8.1': dependencies: '@typescript-eslint/types': 8.8.1 @@ -13780,9 +13624,9 @@ snapshots: '@vitejs/plugin-vue-jsx@4.0.1(vite@5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1))(vue@3.5.12(typescript@5.6.3))': dependencies: - '@babel/core': 7.25.7 - '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.7) - '@vue/babel-plugin-jsx': 1.2.5(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.8) + '@vue/babel-plugin-jsx': 1.2.5(@babel/core@7.25.8) vite: 5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1) vue: 3.5.12(typescript@5.6.3) transitivePeerDependencies: @@ -13830,44 +13674,44 @@ snapshots: '@vitest/utils@2.1.2': dependencies: '@vitest/pretty-format': 2.1.2 - loupe: 3.1.1 + loupe: 3.1.2 tinyrainbow: 1.2.0 - '@volar/language-core@2.4.5': + '@volar/language-core@2.4.6': dependencies: - '@volar/source-map': 2.4.5 + '@volar/source-map': 2.4.6 - '@volar/source-map@2.4.5': {} + '@volar/source-map@2.4.6': {} - '@volar/typescript@2.4.5': + '@volar/typescript@2.4.6': dependencies: - '@volar/language-core': 2.4.5 + '@volar/language-core': 2.4.6 path-browserify: 1.0.1 vscode-uri: 3.0.8 '@vue/babel-helper-vue-transform-on@1.2.5': {} - '@vue/babel-plugin-jsx@1.2.5(@babel/core@7.25.7)': + '@vue/babel-plugin-jsx@1.2.5(@babel/core@7.25.8)': dependencies: '@babel/helper-module-imports': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 - '@babel/plugin-syntax-jsx': 7.25.7(@babel/core@7.25.7) + '@babel/plugin-syntax-jsx': 7.25.7(@babel/core@7.25.8) '@babel/template': 7.25.7 '@babel/traverse': 7.25.7 - '@babel/types': 7.25.7 + '@babel/types': 7.25.8 '@vue/babel-helper-vue-transform-on': 1.2.5 - '@vue/babel-plugin-resolve-type': 1.2.5(@babel/core@7.25.7) + '@vue/babel-plugin-resolve-type': 1.2.5(@babel/core@7.25.8) html-tags: 3.3.1 svg-tags: 1.0.0 optionalDependencies: - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 transitivePeerDependencies: - supports-color - '@vue/babel-plugin-resolve-type@1.2.5(@babel/core@7.25.7)': + '@vue/babel-plugin-resolve-type@1.2.5(@babel/core@7.25.8)': dependencies: '@babel/code-frame': 7.25.7 - '@babel/core': 7.25.7 + '@babel/core': 7.25.8 '@babel/helper-module-imports': 7.25.7 '@babel/helper-plugin-utils': 7.25.7 '@babel/parser': 7.25.8 @@ -13875,14 +13719,6 @@ snapshots: transitivePeerDependencies: - supports-color - '@vue/compiler-core@3.5.11': - dependencies: - '@babel/parser': 7.25.8 - '@vue/shared': 3.5.11 - entities: 4.5.0 - estree-walker: 2.0.2 - source-map-js: 1.2.1 - '@vue/compiler-core@3.5.12': dependencies: '@babel/parser': 7.25.8 @@ -13891,28 +13727,11 @@ snapshots: estree-walker: 2.0.2 source-map-js: 1.2.1 - '@vue/compiler-dom@3.5.11': - dependencies: - '@vue/compiler-core': 3.5.11 - '@vue/shared': 3.5.11 - '@vue/compiler-dom@3.5.12': dependencies: '@vue/compiler-core': 3.5.12 '@vue/shared': 3.5.12 - '@vue/compiler-sfc@3.5.11': - dependencies: - '@babel/parser': 7.25.7 - '@vue/compiler-core': 3.5.11 - '@vue/compiler-dom': 3.5.11 - '@vue/compiler-ssr': 3.5.11 - '@vue/shared': 3.5.11 - estree-walker: 2.0.2 - magic-string: 0.30.12 - postcss: 8.4.47 - source-map-js: 1.2.1 - '@vue/compiler-sfc@3.5.12': dependencies: '@babel/parser': 7.25.8 @@ -13925,11 +13744,6 @@ snapshots: postcss: 8.4.47 source-map-js: 1.2.1 - '@vue/compiler-ssr@3.5.11': - dependencies: - '@vue/compiler-dom': 3.5.11 - '@vue/shared': 3.5.11 - '@vue/compiler-ssr@3.5.12': dependencies: '@vue/compiler-dom': 3.5.12 @@ -13961,7 +13775,7 @@ snapshots: '@vue/devtools-kit@7.4.6': dependencies: '@vue/devtools-shared': 7.4.6 - birpc: 0.2.17 + birpc: 0.2.19 hookable: 5.5.3 mitt: 3.0.1 perfect-debounce: 1.0.0 @@ -13974,7 +13788,7 @@ snapshots: '@vue/language-core@2.1.6(typescript@5.6.3)': dependencies: - '@volar/language-core': 2.4.5 + '@volar/language-core': 2.4.6 '@vue/compiler-dom': 3.5.12 '@vue/compiler-vue2': 2.7.16 '@vue/shared': 3.5.12 @@ -14007,8 +13821,6 @@ snapshots: '@vue/shared': 3.5.12 vue: 3.5.12(typescript@5.6.3) - '@vue/shared@3.5.11': {} - '@vue/shared@3.5.12': {} '@vue/test-utils@2.4.6': @@ -14334,7 +14146,7 @@ snapshots: autoprefixer@10.4.20(postcss@8.4.47): dependencies: browserslist: 4.24.0 - caniuse-lite: 1.0.30001667 + caniuse-lite: 1.0.30001668 fraction.js: 4.3.7 normalize-range: 0.1.2 picocolors: 1.1.0 @@ -14354,34 +14166,34 @@ snapshots: axios@1.7.7: dependencies: follow-redirects: 1.15.9 - form-data: 4.0.0 + form-data: 4.0.1 proxy-from-env: 1.1.0 transitivePeerDependencies: - debug b4a@1.6.7: {} - babel-plugin-polyfill-corejs2@0.4.11(@babel/core@7.25.7): + babel-plugin-polyfill-corejs2@0.4.11(@babel/core@7.25.8): dependencies: '@babel/compat-data': 7.25.8 - '@babel/core': 7.25.7 - '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.8) semver: 6.3.1 transitivePeerDependencies: - supports-color - babel-plugin-polyfill-corejs3@0.10.6(@babel/core@7.25.7): + babel-plugin-polyfill-corejs3@0.10.6(@babel/core@7.25.8): dependencies: - '@babel/core': 7.25.7 - '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.8) core-js-compat: 3.38.1 transitivePeerDependencies: - supports-color - babel-plugin-polyfill-regenerator@0.6.2(@babel/core@7.25.7): + babel-plugin-polyfill-regenerator@0.6.2(@babel/core@7.25.8): dependencies: - '@babel/core': 7.25.7 - '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/helper-define-polyfill-provider': 0.6.2(@babel/core@7.25.8) transitivePeerDependencies: - supports-color @@ -14406,7 +14218,7 @@ snapshots: bintrees@1.0.2: {} - birpc@0.2.17: {} + birpc@0.2.19: {} boolbase@1.0.0: {} @@ -14436,8 +14248,8 @@ snapshots: browserslist@4.24.0: dependencies: - caniuse-lite: 1.0.30001667 - electron-to-chromium: 1.5.32 + caniuse-lite: 1.0.30001668 + electron-to-chromium: 1.5.36 node-releases: 2.0.18 update-browserslist-db: 1.1.1(browserslist@4.24.0) @@ -14461,16 +14273,16 @@ snapshots: c12@1.11.2: dependencies: chokidar: 3.6.0 - confbox: 0.1.7 + confbox: 0.1.8 defu: 6.1.4 dotenv: 16.4.5 giget: 1.2.3 jiti: 1.21.6 - mlly: 1.7.1 + mlly: 1.7.2 ohash: 1.1.4 pathe: 1.1.2 perfect-debounce: 1.0.0 - pkg-types: 1.2.0 + pkg-types: 1.2.1 rc9: 2.1.2 cac@6.7.14: {} @@ -14526,11 +14338,11 @@ snapshots: caniuse-api@3.0.0: dependencies: browserslist: 4.24.0 - caniuse-lite: 1.0.30001667 + caniuse-lite: 1.0.30001668 lodash.memoize: 4.1.2 lodash.uniq: 4.5.0 - caniuse-lite@1.0.30001667: {} + caniuse-lite@1.0.30001668: {} ccount@2.0.1: {} @@ -14539,7 +14351,7 @@ snapshots: assertion-error: 2.0.1 check-error: 2.1.1 deep-eql: 5.0.2 - loupe: 3.1.1 + loupe: 3.1.2 pathval: 2.0.0 chalk-template@1.1.0: @@ -14584,10 +14396,10 @@ snapshots: domutils: 3.1.0 encoding-sniffer: 0.2.0 htmlparser2: 9.1.0 - parse5: 7.1.2 - parse5-htmlparser2-tree-adapter: 7.0.0 + parse5: 7.2.0 + parse5-htmlparser2-tree-adapter: 7.1.0 parse5-parser-stream: 7.1.2 - undici: 6.19.8 + undici: 6.20.0 whatwg-mimetype: 4.0.0 chokidar@3.6.0: @@ -14614,17 +14426,17 @@ snapshots: circular-dependency-scanner@2.3.0: dependencies: - '@ast-grep/napi': 0.27.2 - '@vue/compiler-sfc': 3.5.11 + '@ast-grep/napi': 0.27.3 + '@vue/compiler-sfc': 3.5.12 commander: 12.1.0 get-tsconfig: 4.8.1 graph-cycles: 3.0.0 listr2: 8.2.5 minimatch: 9.0.5 node-cleanup: 2.1.2 - typescript: 5.6.2 + typescript: 5.6.3 update-notifier: 7.3.1 - zx: 8.1.8 + zx: 8.1.9 citty@0.1.6: dependencies: @@ -14767,8 +14579,6 @@ snapshots: concat-map@0.0.1: {} - confbox@0.1.7: {} - confbox@0.1.8: {} config-chain@1.1.13: @@ -15185,9 +14995,9 @@ snapshots: depcheck@1.4.7: dependencies: - '@babel/parser': 7.25.7 + '@babel/parser': 7.25.8 '@babel/traverse': 7.25.7 - '@vue/compiler-sfc': 3.5.11 + '@vue/compiler-sfc': 3.5.12 callsite: 1.0.0 camelcase: 6.3.0 cosmiconfig: 7.1.0 @@ -15338,7 +15148,7 @@ snapshots: dependencies: jake: 10.9.2 - electron-to-chromium@1.5.32: {} + electron-to-chromium@1.5.36: {} element-plus@2.8.5(vue@3.5.12(typescript@5.6.3)): dependencies: @@ -15567,32 +15377,32 @@ snapshots: '@esbuild/win32-ia32': 0.21.5 '@esbuild/win32-x64': 0.21.5 - esbuild@0.23.1: + esbuild@0.24.0: optionalDependencies: - '@esbuild/aix-ppc64': 0.23.1 - '@esbuild/android-arm': 0.23.1 - '@esbuild/android-arm64': 0.23.1 - '@esbuild/android-x64': 0.23.1 - '@esbuild/darwin-arm64': 0.23.1 - '@esbuild/darwin-x64': 0.23.1 - '@esbuild/freebsd-arm64': 0.23.1 - '@esbuild/freebsd-x64': 0.23.1 - '@esbuild/linux-arm': 0.23.1 - '@esbuild/linux-arm64': 0.23.1 - '@esbuild/linux-ia32': 0.23.1 - '@esbuild/linux-loong64': 0.23.1 - '@esbuild/linux-mips64el': 0.23.1 - '@esbuild/linux-ppc64': 0.23.1 - '@esbuild/linux-riscv64': 0.23.1 - '@esbuild/linux-s390x': 0.23.1 - '@esbuild/linux-x64': 0.23.1 - '@esbuild/netbsd-x64': 0.23.1 - '@esbuild/openbsd-arm64': 0.23.1 - '@esbuild/openbsd-x64': 0.23.1 - '@esbuild/sunos-x64': 0.23.1 - '@esbuild/win32-arm64': 0.23.1 - '@esbuild/win32-ia32': 0.23.1 - '@esbuild/win32-x64': 0.23.1 + '@esbuild/aix-ppc64': 0.24.0 + '@esbuild/android-arm': 0.24.0 + '@esbuild/android-arm64': 0.24.0 + '@esbuild/android-x64': 0.24.0 + '@esbuild/darwin-arm64': 0.24.0 + '@esbuild/darwin-x64': 0.24.0 + '@esbuild/freebsd-arm64': 0.24.0 + '@esbuild/freebsd-x64': 0.24.0 + '@esbuild/linux-arm': 0.24.0 + '@esbuild/linux-arm64': 0.24.0 + '@esbuild/linux-ia32': 0.24.0 + '@esbuild/linux-loong64': 0.24.0 + '@esbuild/linux-mips64el': 0.24.0 + '@esbuild/linux-ppc64': 0.24.0 + '@esbuild/linux-riscv64': 0.24.0 + '@esbuild/linux-s390x': 0.24.0 + '@esbuild/linux-x64': 0.24.0 + '@esbuild/netbsd-x64': 0.24.0 + '@esbuild/openbsd-arm64': 0.24.0 + '@esbuild/openbsd-x64': 0.24.0 + '@esbuild/sunos-x64': 0.24.0 + '@esbuild/win32-arm64': 0.24.0 + '@esbuild/win32-ia32': 0.24.0 + '@esbuild/win32-x64': 0.24.0 escalade@3.2.0: {} @@ -15614,15 +15424,15 @@ snapshots: optionalDependencies: source-map: 0.6.1 - eslint-compat-utils@0.5.1(eslint@9.12.0(jiti@2.2.1)): + eslint-compat-utils@0.5.1(eslint@9.12.0(jiti@2.3.3)): dependencies: - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) semver: 7.6.3 - eslint-config-turbo@2.1.3(eslint@9.12.0(jiti@2.2.1)): + eslint-config-turbo@2.1.3(eslint@9.12.0(jiti@2.3.3)): dependencies: - eslint: 9.12.0(jiti@2.2.1) - eslint-plugin-turbo: 2.1.3(eslint@9.12.0(jiti@2.2.1)) + eslint: 9.12.0(jiti@2.3.3) + eslint-plugin-turbo: 2.1.3(eslint@9.12.0(jiti@2.3.3)) eslint-import-resolver-node@0.3.9: dependencies: @@ -15632,30 +15442,30 @@ snapshots: transitivePeerDependencies: - supports-color - eslint-plugin-command@0.2.6(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-command@0.2.6(eslint@9.12.0(jiti@2.3.3)): dependencies: '@es-joy/jsdoccomment': 0.48.0 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) - eslint-plugin-es-x@7.8.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-es-x@7.8.0(eslint@9.12.0(jiti@2.3.3)): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@eslint-community/regexpp': 4.11.1 - eslint: 9.12.0(jiti@2.2.1) - eslint-compat-utils: 0.5.1(eslint@9.12.0(jiti@2.2.1)) + eslint: 9.12.0(jiti@2.3.3) + eslint-compat-utils: 0.5.1(eslint@9.12.0(jiti@2.3.3)) - eslint-plugin-eslint-comments@3.2.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-eslint-comments@3.2.0(eslint@9.12.0(jiti@2.3.3)): dependencies: escape-string-regexp: 1.0.5 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) ignore: 5.3.2 - eslint-plugin-import-x@4.3.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3): + eslint-plugin-import-x@4.3.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3): dependencies: - '@typescript-eslint/utils': 8.8.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) debug: 4.3.7 doctrine: 3.0.0 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) eslint-import-resolver-node: 0.3.9 get-tsconfig: 4.8.1 is-glob: 4.0.3 @@ -15667,40 +15477,40 @@ snapshots: - supports-color - typescript - eslint-plugin-jsdoc@50.3.2(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-jsdoc@50.3.2(eslint@9.12.0(jiti@2.3.3)): dependencies: '@es-joy/jsdoccomment': 0.48.0 are-docs-informative: 0.0.2 comment-parser: 1.4.1 debug: 4.3.7 escape-string-regexp: 4.0.0 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) espree: 10.2.0 esquery: 1.6.0 parse-imports: 2.2.1 semver: 7.6.3 spdx-expression-parse: 4.0.0 - synckit: 0.9.1 + synckit: 0.9.2 transitivePeerDependencies: - supports-color - eslint-plugin-jsonc@2.16.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-jsonc@2.16.0(eslint@9.12.0(jiti@2.3.3)): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) - eslint: 9.12.0(jiti@2.2.1) - eslint-compat-utils: 0.5.1(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) + eslint: 9.12.0(jiti@2.3.3) + eslint-compat-utils: 0.5.1(eslint@9.12.0(jiti@2.3.3)) espree: 9.6.1 graphemer: 1.4.0 jsonc-eslint-parser: 2.4.0 natural-compare: 1.4.0 synckit: 0.6.2 - eslint-plugin-n@17.11.1(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-n@17.11.1(eslint@9.12.0(jiti@2.3.3)): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) enhanced-resolve: 5.17.1 - eslint: 9.12.0(jiti@2.2.1) - eslint-plugin-es-x: 7.8.0(eslint@9.12.0(jiti@2.2.1)) + eslint: 9.12.0(jiti@2.3.3) + eslint-plugin-es-x: 7.8.0(eslint@9.12.0(jiti@2.3.3)) get-tsconfig: 4.8.1 globals: 15.11.0 ignore: 5.3.2 @@ -15709,52 +15519,52 @@ snapshots: eslint-plugin-no-only-tests@3.3.0: {} - eslint-plugin-perfectionist@3.8.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)(vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.2.1))): + eslint-plugin-perfectionist@3.8.0(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)(vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.3.3))): dependencies: - '@typescript-eslint/types': 8.8.0 - '@typescript-eslint/utils': 8.8.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) - eslint: 9.12.0(jiti@2.2.1) + '@typescript-eslint/types': 8.8.1 + '@typescript-eslint/utils': 8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) + eslint: 9.12.0(jiti@2.3.3) minimatch: 9.0.5 natural-compare-lite: 1.4.0 optionalDependencies: - vue-eslint-parser: 9.4.3(eslint@9.12.0(jiti@2.2.1)) + vue-eslint-parser: 9.4.3(eslint@9.12.0(jiti@2.3.3)) transitivePeerDependencies: - supports-color - typescript - eslint-plugin-prettier@5.2.1(@types/eslint@9.6.1)(eslint@9.12.0(jiti@2.2.1))(prettier@3.3.3): + eslint-plugin-prettier@5.2.1(@types/eslint@9.6.1)(eslint@9.12.0(jiti@2.3.3))(prettier@3.3.3): dependencies: - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) prettier: 3.3.3 prettier-linter-helpers: 1.0.0 - synckit: 0.9.1 + synckit: 0.9.2 optionalDependencies: '@types/eslint': 9.6.1 - eslint-plugin-regexp@2.6.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-regexp@2.6.0(eslint@9.12.0(jiti@2.3.3)): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@eslint-community/regexpp': 4.11.1 comment-parser: 1.4.1 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) jsdoc-type-pratt-parser: 4.1.0 refa: 0.12.1 regexp-ast-analysis: 0.7.1 scslre: 0.3.0 - eslint-plugin-turbo@2.1.3(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-turbo@2.1.3(eslint@9.12.0(jiti@2.3.3)): dependencies: dotenv: 16.0.3 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) - eslint-plugin-unicorn@56.0.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-unicorn@56.0.0(eslint@9.12.0(jiti@2.3.3)): dependencies: '@babel/helper-validator-identifier': 7.25.7 - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) ci-info: 4.0.0 clean-regexp: 1.0.0 core-js-compat: 3.38.1 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) esquery: 1.6.0 globals: 15.11.0 indent-string: 4.0.0 @@ -15767,33 +15577,33 @@ snapshots: semver: 7.6.3 strip-indent: 3.0.0 - eslint-plugin-unused-imports@4.1.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-unused-imports@4.1.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3)): dependencies: - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) optionalDependencies: - '@typescript-eslint/eslint-plugin': 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/eslint-plugin': 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) - eslint-plugin-vitest@0.5.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3)(vitest@2.1.2(@types/node@22.7.5)(happy-dom@15.7.4)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)): + eslint-plugin-vitest@0.5.4(@typescript-eslint/eslint-plugin@8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3)(vitest@2.1.2(@types/node@22.7.5)(happy-dom@15.7.4)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)): dependencies: - '@typescript-eslint/utils': 7.18.0(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) - eslint: 9.12.0(jiti@2.2.1) + '@typescript-eslint/utils': 7.18.0(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) + eslint: 9.12.0(jiti@2.3.3) optionalDependencies: - '@typescript-eslint/eslint-plugin': 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3))(eslint@9.12.0(jiti@2.2.1))(typescript@5.6.3) + '@typescript-eslint/eslint-plugin': 8.8.1(@typescript-eslint/parser@8.8.1(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3))(eslint@9.12.0(jiti@2.3.3))(typescript@5.6.3) vitest: 2.1.2(@types/node@22.7.5)(happy-dom@15.7.4)(less@4.2.0)(sass@1.79.5)(terser@5.34.1) transitivePeerDependencies: - supports-color - typescript - eslint-plugin-vue@9.29.0(eslint@9.12.0(jiti@2.2.1)): + eslint-plugin-vue@9.29.0(eslint@9.12.0(jiti@2.3.3)): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) - eslint: 9.12.0(jiti@2.2.1) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) + eslint: 9.12.0(jiti@2.3.3) globals: 13.24.0 natural-compare: 1.4.0 nth-check: 2.1.1 postcss-selector-parser: 6.1.2 semver: 7.6.3 - vue-eslint-parser: 9.4.3(eslint@9.12.0(jiti@2.2.1)) + vue-eslint-parser: 9.4.3(eslint@9.12.0(jiti@2.3.3)) xml-name-validator: 4.0.0 transitivePeerDependencies: - supports-color @@ -15812,9 +15622,9 @@ snapshots: eslint-visitor-keys@4.1.0: {} - eslint@9.12.0(jiti@2.2.1): + eslint@9.12.0(jiti@2.3.3): dependencies: - '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.2.1)) + '@eslint-community/eslint-utils': 4.4.0(eslint@9.12.0(jiti@2.3.3)) '@eslint-community/regexpp': 4.11.1 '@eslint/config-array': 0.18.0 '@eslint/core': 0.6.0 @@ -15850,7 +15660,7 @@ snapshots: optionator: 0.9.4 text-table: 0.2.0 optionalDependencies: - jiti: 2.2.1 + jiti: 2.3.3 transitivePeerDependencies: - supports-color @@ -16049,7 +15859,7 @@ snapshots: cross-spawn: 7.0.3 signal-exit: 4.1.0 - form-data@4.0.0: + form-data@4.0.1: dependencies: asynckit: 0.4.0 combined-stream: 1.0.8 @@ -16133,8 +15943,6 @@ snapshots: get-east-asian-width@1.2.0: {} - get-func-name@2.0.2: {} - get-intrinsic@1.2.4: dependencies: es-errors: 1.3.0 @@ -16755,7 +16563,7 @@ snapshots: jiti@1.21.6: {} - jiti@2.2.1: {} + jiti@2.3.3: {} jju@1.4.0: {} @@ -16927,8 +16735,8 @@ snapshots: get-port-please: 3.1.2 h3: 1.13.0 http-shutdown: 1.2.2 - jiti: 2.2.1 - mlly: 1.7.1 + jiti: 2.3.3 + mlly: 1.7.2 node-forge: 1.3.1 pathe: 1.1.2 std-env: 3.7.0 @@ -17037,9 +16845,7 @@ snapshots: dependencies: js-tokens: 4.0.0 - loupe@3.1.1: - dependencies: - get-func-name: 2.0.2 + loupe@3.1.2: {} lower-case@2.0.2: dependencies: @@ -17070,10 +16876,6 @@ snapshots: dependencies: sourcemap-codec: 1.4.8 - magic-string@0.30.11: - dependencies: - '@jridgewell/sourcemap-codec': 1.5.0 - magic-string@0.30.12: dependencies: '@jridgewell/sourcemap-codec': 1.5.0 @@ -17256,33 +17058,26 @@ snapshots: mkdirp@1.0.4: {} - mkdist@1.5.9(sass@1.79.5)(typescript@5.6.3)(vue-tsc@2.1.6(typescript@5.6.3)): + mkdist@1.6.0(sass@1.79.5)(typescript@5.6.3)(vue-tsc@2.1.6(typescript@5.6.3)): dependencies: autoprefixer: 10.4.20(postcss@8.4.47) citty: 0.1.6 cssnano: 7.0.6(postcss@8.4.47) defu: 6.1.4 - esbuild: 0.23.1 - fast-glob: 3.3.2 + esbuild: 0.24.0 jiti: 1.21.6 - mlly: 1.7.1 + mlly: 1.7.2 pathe: 1.1.2 - pkg-types: 1.2.0 + pkg-types: 1.2.1 postcss: 8.4.47 postcss-nested: 6.2.0(postcss@8.4.47) semver: 7.6.3 + tinyglobby: 0.2.9 optionalDependencies: sass: 1.79.5 typescript: 5.6.3 vue-tsc: 2.1.6(typescript@5.6.3) - mlly@1.7.1: - dependencies: - acorn: 8.12.1 - pathe: 1.1.2 - pkg-types: 1.2.0 - ufo: 1.5.4 - mlly@1.7.2: dependencies: acorn: 8.12.1 @@ -17330,7 +17125,7 @@ snapshots: highlight.js: 11.10.0 lodash: 4.17.21 lodash-es: 4.17.21 - seemly: 0.3.8 + seemly: 0.3.9 treemate: 0.3.11 vdirs: 0.1.8(vue@3.5.12(typescript@5.6.3)) vooks: 0.2.12(vue@3.5.12(typescript@5.6.3)) @@ -17394,17 +17189,17 @@ snapshots: klona: 2.0.6 knitwork: 1.1.0 listhen: 1.9.0 - magic-string: 0.30.11 + magic-string: 0.30.12 mime: 4.0.4 - mlly: 1.7.1 + mlly: 1.7.2 mri: 1.2.0 node-fetch-native: 1.6.4 - ofetch: 1.4.0 + ofetch: 1.4.1 ohash: 1.1.4 openapi-typescript: 6.7.6 pathe: 1.1.2 perfect-debounce: 1.0.0 - pkg-types: 1.2.0 + pkg-types: 1.2.1 pretty-bytes: 6.1.1 radix3: 1.1.2 rollup: 4.24.0 @@ -17551,7 +17346,7 @@ snapshots: has-symbols: 1.0.3 object-keys: 1.1.1 - ofetch@1.4.0: + ofetch@1.4.1: dependencies: destr: 2.0.3 node-fetch-native: 1.6.4 @@ -17671,7 +17466,7 @@ snapshots: registry-url: 6.0.1 semver: 7.6.3 - package-manager-detector@0.2.0: {} + package-manager-detector@0.2.2: {} param-case@3.0.4: dependencies: @@ -17704,16 +17499,16 @@ snapshots: parse-passwd@1.0.0: {} - parse5-htmlparser2-tree-adapter@7.0.0: + parse5-htmlparser2-tree-adapter@7.1.0: dependencies: domhandler: 5.0.3 - parse5: 7.1.2 + parse5: 7.2.0 parse5-parser-stream@7.1.2: dependencies: - parse5: 7.1.2 + parse5: 7.2.0 - parse5@7.1.2: + parse5@7.2.0: dependencies: entities: 4.5.0 @@ -17772,9 +17567,9 @@ snapshots: pify@4.0.1: {} - pinia-plugin-persistedstate@4.1.1(pinia@2.2.2(typescript@5.6.3)(vue@3.5.12(typescript@5.6.3)))(rollup@3.29.5): + pinia-plugin-persistedstate@4.1.1(pinia@2.2.2(typescript@5.6.3)(vue@3.5.12(typescript@5.6.3)))(rollup@4.24.0): dependencies: - '@nuxt/kit': 3.13.2(rollup@3.29.5) + '@nuxt/kit': 3.13.2(rollup@4.24.0) deep-pick-omit: 1.2.1 defu: 6.1.4 destr: 2.0.3 @@ -17796,12 +17591,6 @@ snapshots: pirates@4.0.6: {} - pkg-types@1.2.0: - dependencies: - confbox: 0.1.7 - mlly: 1.7.1 - pathe: 1.1.2 - pkg-types@1.2.1: dependencies: confbox: 0.1.8 @@ -18665,7 +18454,7 @@ snapshots: extend-shallow: 2.0.1 kind-of: 6.0.3 - seemly@0.3.8: {} + seemly@0.3.9: {} semver-compare@1.0.0: {} @@ -19176,7 +18965,7 @@ snapshots: dependencies: tslib: 2.7.0 - synckit@0.9.1: + synckit@0.9.2: dependencies: '@pkgr/core': 0.1.1 tslib: 2.7.0 @@ -19409,8 +19198,6 @@ snapshots: typescript@5.4.2: {} - typescript@5.6.2: {} - typescript@5.6.3: {} ufo@1.5.4: {} @@ -19438,16 +19225,16 @@ snapshots: globby: 13.2.2 hookable: 5.5.3 jiti: 1.21.6 - magic-string: 0.30.11 - mkdist: 1.5.9(sass@1.79.5)(typescript@5.6.3)(vue-tsc@2.1.6(typescript@5.6.3)) - mlly: 1.7.1 + magic-string: 0.30.12 + mkdist: 1.6.0(sass@1.79.5)(typescript@5.6.3)(vue-tsc@2.1.6(typescript@5.6.3)) + mlly: 1.7.2 pathe: 1.1.2 - pkg-types: 1.2.0 + pkg-types: 1.2.1 pretty-bytes: 6.1.1 rollup: 3.29.5 rollup-plugin-dts: 6.1.1(rollup@3.29.5)(typescript@5.6.3) scule: 1.3.0 - untyped: 1.5.0 + untyped: 1.5.1 optionalDependencies: typescript: 5.6.3 transitivePeerDependencies: @@ -19472,7 +19259,7 @@ snapshots: dependencies: '@fastify/busboy': 2.1.1 - undici@6.19.8: {} + undici@6.20.0: {} unenv@1.10.0: dependencies: @@ -19497,25 +19284,6 @@ snapshots: unicorn-magic@0.3.0: {} - unimport@3.13.1(rollup@3.29.5): - dependencies: - '@rollup/pluginutils': 5.1.2(rollup@3.29.5) - acorn: 8.12.1 - escape-string-regexp: 5.0.0 - estree-walker: 3.0.3 - fast-glob: 3.3.2 - local-pkg: 0.5.0 - magic-string: 0.30.12 - mlly: 1.7.1 - pathe: 1.1.2 - pkg-types: 1.2.0 - scule: 1.3.0 - strip-literal: 2.1.0 - unplugin: 1.14.1 - transitivePeerDependencies: - - rollup - - webpack-sources - unimport@3.13.1(rollup@4.24.0): dependencies: '@rollup/pluginutils': 5.1.2(rollup@4.24.0) @@ -19525,9 +19293,9 @@ snapshots: fast-glob: 3.3.2 local-pkg: 0.5.0 magic-string: 0.30.12 - mlly: 1.7.1 + mlly: 1.7.2 pathe: 1.1.2 - pkg-types: 1.2.0 + pkg-types: 1.2.1 scule: 1.3.0 strip-literal: 2.1.0 unplugin: 1.14.1 @@ -19578,7 +19346,7 @@ snapshots: dependencies: '@rollup/pluginutils': 5.1.2(rollup@4.24.0) es-module-lexer: 1.5.4 - magic-string: 0.30.11 + magic-string: 0.30.12 unplugin: 1.14.1 transitivePeerDependencies: - rollup @@ -19599,7 +19367,7 @@ snapshots: lru-cache: 10.4.3 mri: 1.2.0 node-fetch-native: 1.6.4 - ofetch: 1.4.0 + ofetch: 1.4.1 ufo: 1.5.4 optionalDependencies: ioredis: 5.4.1 @@ -19612,13 +19380,13 @@ snapshots: consola: 3.2.3 pathe: 1.1.2 - untyped@1.5.0: + untyped@1.5.1: dependencies: - '@babel/core': 7.25.7 - '@babel/standalone': 7.25.7 - '@babel/types': 7.25.7 + '@babel/core': 7.25.8 + '@babel/standalone': 7.25.8 + '@babel/types': 7.25.8 defu: 6.1.4 - jiti: 2.2.1 + jiti: 2.3.3 mri: 1.2.0 scule: 1.3.0 transitivePeerDependencies: @@ -19628,9 +19396,9 @@ snapshots: dependencies: knitwork: 1.1.0 magic-string: 0.30.12 - mlly: 1.7.1 + mlly: 1.7.2 pathe: 1.1.2 - pkg-types: 1.2.0 + pkg-types: 1.2.1 unplugin: 1.14.1 transitivePeerDependencies: - webpack-sources @@ -19726,13 +19494,13 @@ snapshots: dependencies: '@microsoft/api-extractor': 7.47.7(@types/node@22.7.5) '@rollup/pluginutils': 5.1.2(rollup@4.24.0) - '@volar/typescript': 2.4.5 + '@volar/typescript': 2.4.6 '@vue/language-core': 2.1.6(typescript@5.6.3) compare-versions: 6.1.1 debug: 4.3.7 kolorist: 1.8.0 local-pkg: 0.5.0 - magic-string: 0.30.11 + magic-string: 0.30.12 typescript: 5.6.3 optionalDependencies: vite: 5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1) @@ -19778,12 +19546,12 @@ snapshots: '@rollup/pluginutils': 5.1.2(rollup@4.24.0) es-module-lexer: 1.5.4 rollup: 4.24.0 - xe-utils: 3.5.30 + xe-utils: 3.5.31 vite-plugin-lib-inject-css@2.1.1(vite@5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)): dependencies: '@ast-grep/napi': 0.22.6 - magic-string: 0.30.11 + magic-string: 0.30.12 picocolors: 1.1.0 vite: 5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1) @@ -19816,12 +19584,12 @@ snapshots: vite-plugin-vue-inspector@5.2.0(vite@5.4.8(@types/node@22.7.5)(less@4.2.0)(sass@1.79.5)(terser@5.34.1)): dependencies: - '@babel/core': 7.25.7 - '@babel/plugin-proposal-decorators': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.7) - '@babel/plugin-syntax-import-meta': 7.10.4(@babel/core@7.25.7) - '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.7) - '@vue/babel-plugin-jsx': 1.2.5(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/plugin-proposal-decorators': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-syntax-import-attributes': 7.25.7(@babel/core@7.25.8) + '@babel/plugin-syntax-import-meta': 7.10.4(@babel/core@7.25.8) + '@babel/plugin-transform-typescript': 7.25.7(@babel/core@7.25.8) + '@vue/babel-plugin-jsx': 1.2.5(@babel/core@7.25.8) '@vue/compiler-dom': 3.5.12 kolorist: 1.8.0 magic-string: 0.30.12 @@ -19843,7 +19611,7 @@ snapshots: vitepress-plugin-group-icons@1.2.4: dependencies: - '@iconify-json/logos': 1.2.2 + '@iconify-json/logos': 1.2.3 '@iconify-json/vscode-icons': 1.2.2 '@iconify/utils': 2.1.33 transitivePeerDependencies: @@ -19909,7 +19677,7 @@ snapshots: '@vitest/utils': 2.1.2 chai: 5.1.1 debug: 4.3.7 - magic-string: 0.30.11 + magic-string: 0.30.12 pathe: 1.1.2 std-env: 3.7.0 tinybench: 2.9.0 @@ -19948,10 +19716,10 @@ snapshots: dependencies: vue: 3.5.12(typescript@5.6.3) - vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.2.1)): + vue-eslint-parser@9.4.3(eslint@9.12.0(jiti@2.3.3)): dependencies: debug: 4.3.7 - eslint: 9.12.0(jiti@2.2.1) + eslint: 9.12.0(jiti@2.3.3) eslint-scope: 7.2.2 eslint-visitor-keys: 3.4.3 espree: 9.6.1 @@ -19975,7 +19743,7 @@ snapshots: vue-tsc@2.1.6(typescript@5.6.3): dependencies: - '@volar/typescript': 2.4.5 + '@volar/typescript': 2.4.6 '@vue/language-core': 2.1.6(typescript@5.6.3) semver: 7.6.3 typescript: 5.6.3 @@ -20001,7 +19769,7 @@ snapshots: '@juggle/resize-observer': 3.4.0 css-render: 0.15.14 evtd: 0.2.4 - seemly: 0.3.8 + seemly: 0.3.9 vdirs: 0.1.8(vue@3.5.12(typescript@5.6.3)) vooks: 0.2.12(vue@3.5.12(typescript@5.6.3)) vue: 3.5.12(typescript@5.6.3) @@ -20010,7 +19778,7 @@ snapshots: dependencies: '@vxe-ui/core': 4.0.13 - vxe-table@4.7.86: + vxe-table@4.7.87: dependencies: vxe-pc-ui: 4.2.19 @@ -20102,10 +19870,10 @@ snapshots: workbox-build@7.1.1: dependencies: '@apideck/better-ajv-errors': 0.3.6(ajv@8.17.1) - '@babel/core': 7.25.7 - '@babel/preset-env': 7.25.8(@babel/core@7.25.7) + '@babel/core': 7.25.8 + '@babel/preset-env': 7.25.8(@babel/core@7.25.8) '@babel/runtime': 7.25.7 - '@rollup/plugin-babel': 5.3.1(@babel/core@7.25.7)(rollup@2.79.2) + '@rollup/plugin-babel': 5.3.1(@babel/core@7.25.8)(rollup@2.79.2) '@rollup/plugin-node-resolve': 15.3.0(rollup@2.79.2) '@rollup/plugin-replace': 2.4.2(rollup@2.79.2) '@rollup/plugin-terser': 0.4.4(rollup@2.79.2) @@ -20236,8 +20004,6 @@ snapshots: xdg-basedir@5.1.0: {} - xe-utils@3.5.30: {} - xe-utils@3.5.31: {} xml-name-validator@4.0.0: {} @@ -20329,7 +20095,7 @@ snapshots: zwitch@2.0.4: {} - zx@8.1.8: + zx@8.1.9: optionalDependencies: '@types/fs-extra': 11.0.4 - '@types/node': 22.7.4 + '@types/node': 22.7.5